Spring Boot- Send email using FreeMarker
On this page, we’ll explore how to send an email using Spring Boot and FreeMarker. FreeMarkerTemplateUtils
is a utility class for working with FreeMarker template to send the email.
String htmlText= FreeMarkerTemplateUtils.processTemplateIntoString(Template template, Object model);
It returns the processed HTML in the form of a string and throws:
IOException
– if a template is not available on the specified path.
TemplateException
– parse exception if something specified wrong in the template.
See the code snippet that only different from the how to send email using Spring Boot example.
....
FreeMarkerConfigurer freemarkerConfig;
{
Template t = freemarkerConfig.getConfiguration().getTemplate("/email/welcome.ftlh");
String htmlText= FreeMarkerTemplateUtils.processTemplateIntoString(t, dataMap);
}
....
// We can also get a Template object like this...
...
Configuration config;
{
Template t = config.getTemplate("/email/welcome.ftlh");
String htmlText= FreeMarkerTemplateUtils.processTemplateIntoString(t, dataMap);
}
...
Note: Spring Boot recently changed the default extension from
.ftl
to.ftlh
Technology Used
Find the list of all tools/technologies used in this application.
- Spring Tool Suite 4
- JDK 8
- Spring Boot 2.2.6.RELEASE
- FreeMarker 2.3.30
- Java Mail 1.6.2
- Maven 3.2
Dependencies Required
Add the following dependencies to the pom.xml.
<!-- FreeMarker -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-freemarker</artifactId>
</dependency>
<!-- Java Mailer -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-mail</artifactId>
</dependency>
Project Structure
The final project structure of our application in STS 4 IDE will look like as follows:
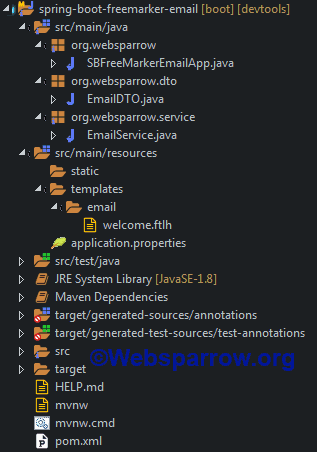
Mail Server Properties
Define the mail server properties in the application.properties file.
# Mail server properties
spring.mail.host=smtp.gmail.com
spring.mail.port=587
spring.mail.username=XXXXXXXXXXX
spring.mail.password=xxxxxxx
spring.mail.properties.mail.smtp.auth=true
spring.mail.properties.mail.smtp.connectiontimeout=5000
spring.mail.properties.mail.smtp.timeout=5000
spring.mail.properties.mail.smtp.writetimeout=5000
spring.mail.properties.mail.smtp.starttls.enable=true
Email DTO
EmailDTO
object is used to pass as an argument for our EmailService
to encapsulate the details of an email like recipient email, email subject, etc.
package org.websparrow.dto;
import java.util.Map;
public class EmailDTO {
private String to;
private String subject;
private Map<String, Object> emailData;
// Generate Getters and Setters...
}
Email Service
A service class that actually pullulate the data to the template and send an email to the recipient address.
package org.websparrow.service;
import java.nio.charset.StandardCharsets;
import javax.mail.internet.MimeMessage;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.mail.javamail.JavaMailSender;
import org.springframework.mail.javamail.MimeMessageHelper;
import org.springframework.stereotype.Service;
import org.springframework.ui.freemarker.FreeMarkerTemplateUtils;
import org.springframework.web.servlet.view.freemarker.FreeMarkerConfigurer;
import org.websparrow.dto.EmailDTO;
@Service
public class EmailService {
@Autowired
private JavaMailSender mailSender;
@Autowired
private FreeMarkerConfigurer freemarkerConfig;
public void sendWelcomeEmail(EmailDTO emailDTO) {
System.out.println("##### Started sending welcome email ####");
MimeMessage mimeMessage = mailSender.createMimeMessage();
try {
MimeMessageHelper helper = new MimeMessageHelper(mimeMessage,
MimeMessageHelper.MULTIPART_MODE_MIXED_RELATED,
StandardCharsets.UTF_8.name());
String templateContent = FreeMarkerTemplateUtils
.processTemplateIntoString(freemarkerConfig.getConfiguration()
.getTemplate("/email/welcome.ftlh"),
emailDTO.getEmailData());
helper.setTo(emailDTO.getTo());
helper.setSubject(emailDTO.getSubject());
helper.setText(templateContent, true);
mailSender.send(mimeMessage);
System.out.println("######## Welcome email sent ######");
} catch (Exception e) {
System.out.println("Sending welcome email failed, check log...");
e.printStackTrace();
}
}
}
FreeMarker HTML Email Template
Create your beautiful HTML email template and put it under the src/main/resources/templates/email
folder. Make sure the file extension of the template must be .ftlh
.
<!DOCTYPE html>
<html>
<head>
<title>Welcome Letter</title>
</head>
<body>
<h3>Dear ${name},</h3>
<p>A warm welcome and lots of good wishes on becoming part of our growing team.</p>
<p>We are pleased to inform you that you will work with the following team members:</p>
<ol>
<#list teamMembers as teamMember>
<li>${teamMember}</li>
</#list>
</ol>
<p>Regards</p>
<p>Websparrow.org Team</p>
<p>Location: ${location}</p>
</body>
</html>
Run it
Create an object of EmailDTO
and sets the necessary data, call the EmailService
to send an email.
package org.websparrow;
import java.util.Arrays;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.CommandLineRunner;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.websparrow.dto.EmailDTO;
import org.websparrow.service.EmailService;
@SpringBootApplication
public class SBFreeMarkerEmailApp implements CommandLineRunner {
@Autowired
private EmailService emailService;
@Override
public void run(String... args) throws Exception {
System.out.println("###### Email sending initiated ######");
EmailDTO email = new EmailDTO();
email.setTo("[email protected]");
email.setSubject("Welcome Letter via Spring Boot + FreeMarker");
// Populate the template data
Map<String, Object> templateData = new HashMap<>();
templateData.put("name", "Atul Rai");
// List of team members...
List<String> teamMembers = Arrays.asList("Tendulkar", "Manish", "Dhirendra");
templateData.put("teamMembers", teamMembers);
templateData.put("location", "India");
email.setEmailData(templateData);
// Calling email service
emailService.sendWelcomeEmail(email);
}
public static void main(String[] args) {
SpringApplication.run(SBFreeMarkerEmailApp.class, args);
}
}
Test it
Start your application by executing the above class and you will receive an email on the provided email address if everything configured correctly.
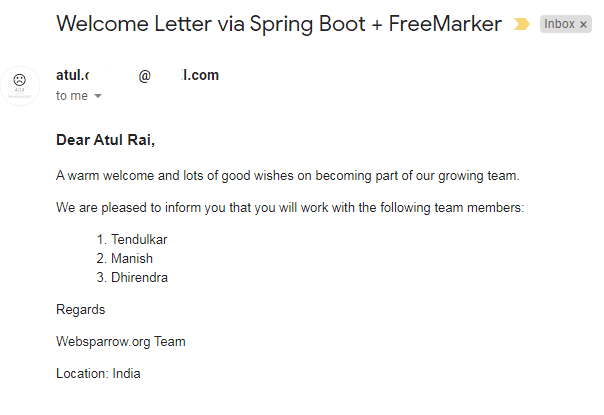
Download Source Code: spring-boot-send-email-using-freemarker.zip
References
- Spring Boot + FreeMarker Example
- How to send email using Spring Boot
- Example of sending email in Java using Gmail SMTP
- javax.mail.AuthenticationFailedException