How to send email using Spring Boot
On this page, we’ll learn how to send an email using the Spring Boot application via SMTP. Spring provides a JavaMailSender
interface on top of JavaMail APIs. And we can get it by adding spring-boot-starter-mail
dependency to the pom.xml file.
You can send the following types of email using Spring’s JavaMailSender
API:
1. Simple Text Email: It will send the plain text email to the recipient email address.
// Some code
simpleMessage.setTo("[email protected]");
simpleMessage.setSubject("Spring Boot=> Sending simple email");
simpleMessage.setText("Dear JackSparrow, Hope you are doing well.");
javaMailSender.send(simpleMessage);
// Some more code
2. Email with Attachment: Sending email with an attachment.
// Some code
try {
MimeMessageHelper mimeMessageHelper = new MimeMessageHelper(mimeMessage, true);
mimeMessageHelper.setTo("[email protected]");
mimeMessageHelper.setSubject("Spring Boot=> Sending email with attachment");
mimeMessageHelper.setText("Dear Atul, I have sent you Websparrow.org new logo. PFA.");
// Attach the attachment
mimeMessageHelper.addAttachment("logo.png", new ClassPathResource("logo-100.png"));
javaMailSender.send(mimeMessage);
}catch(Exception e){
// Handle the exception
}
You can also attach the attachment from the file system, like this:
String attachmentPath = "C:\\Users\\Sparrow\\Desktop\\new logo\\final\\youtube.jpg"; FileSystemResource file = new FileSystemResource(new File(attachmentPath)); mimeMessageHelper.addAttachment("Attachment.jpg", file);
3. HTML Email: In this, you can use the HTML tag to design your email. It is kind similar to the Email with Attachment. And we can also attach the attachment with HTML email.
// Some code
try {
MimeMessageHelper mimeMessageHelper = new MimeMessageHelper(mimeMessage, true);
mimeMessageHelper.setTo("[email protected]");
mimeMessageHelper.setSubject("Spring Boot=> Sending HTML email");
String html = "<h3>Dear Manish</h3></br>"
+ "<p>Many many congratulation for joining "
+ "<strong>Websparrow.org Team</strong>.</p>"
+ "</br></br>"
+ "<p>You are entitled for <code>Rs.5000</code> "
+ "as joning bonus.</p>";
mimeMessageHelper.setText(html, true);
javaMailSender.send(mimeMessage);
}catch(Exception e){
// Handle the exception
}
1. Technology Used
Find the list of all tools/technologies used in this application.
- Spring Tool Suite 4
- JDK 8
- Spring Boot 2.2.6.RELEASE
- Maven 3.2
2. Dependencies Required
Add the below dependencies to your pom.xml file.
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-mail</artifactId>
</dependency>
3. Project Structure
The final project structure of our application in STS 4 IDE will look like as follows:
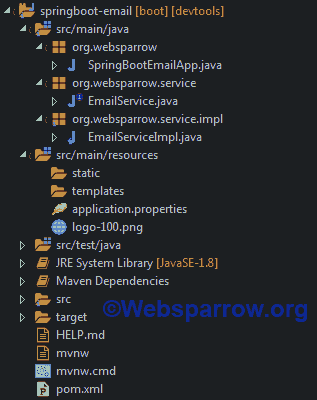
4. Mail Server Properties
application.properties file of Spring Boot application is used to define the mail server properties. For example, the properties for Gmail SMTP Server can be specified as:
# Mail server properties
spring.mail.host=smtp.gmail.com
spring.mail.port=587
spring.mail.username=<your-gmail-id>
spring.mail.password=<gamil-password>
spring.mail.properties.mail.smtp.auth=true
spring.mail.properties.mail.smtp.connectiontimeout=5000
spring.mail.properties.mail.smtp.timeout=5000
spring.mail.properties.mail.smtp.writetimeout=5000
spring.mail.properties.mail.smtp.starttls.enable=true
5. Sending Email
For sending an email to the recipient’s email address, you need to autowire the JavaMailSender
in the EmailServiceImpl
class.
5.1 Email Service Interface
Declaration of methods which will be implemented by EmailServiceImpl
class.
package org.websparrow.service;
import org.springframework.stereotype.Service;
@Service
public interface EmailService {
void sendTextEmail();
void sendEmailWithAttachment();
void sendHTMLEmail();
}
5.2 Email Service Implementation
Implementation of the EmailService
interface.
package org.websparrow.service.impl;
import javax.mail.MessagingException;
import javax.mail.internet.MimeMessage;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.core.io.ClassPathResource;
import org.springframework.mail.SimpleMailMessage;
import org.springframework.mail.javamail.JavaMailSender;
import org.springframework.mail.javamail.MimeMessageHelper;
import org.springframework.stereotype.Service;
import org.websparrow.service.EmailService;
@Service
public class EmailServiceImpl implements EmailService {
private static final Logger logger = LoggerFactory
.getLogger(EmailServiceImpl.class);
@Autowired
private JavaMailSender javaMailSender;
@Override
public void sendTextEmail() {
logger.info("Simple Email sending start");
SimpleMailMessage simpleMessage = new SimpleMailMessage();
simpleMessage.setTo("[email protected]");
simpleMessage.setSubject("Spring Boot=> Sending simple email");
simpleMessage.setText("Dear Dhirendra, Hope you are doing well.");
javaMailSender.send(simpleMessage);
logger.info("Simple Email sent");
}
@Override
public void sendEmailWithAttachment() {
logger.info("Sending email with attachment start");
MimeMessage mimeMessage = javaMailSender.createMimeMessage();
try {
// Set multipart mime message true
MimeMessageHelper mimeMessageHelper = new MimeMessageHelper(mimeMessage,
true);
mimeMessageHelper.setTo("[email protected]");
mimeMessageHelper
.setSubject("Spring Boot=> Sending email with attachment");
mimeMessageHelper.setText(
"Dear Santosh, I have sent you Websparrow.org new logo. PFA.");
// Attach the attachment
mimeMessageHelper.addAttachment("logo.png",
new ClassPathResource("logo-100.png"));
javaMailSender.send(mimeMessage);
} catch (MessagingException e) {
logger.error("Exeception=>sendEmailWithAttachment ", e);
}
logger.info("Email with attachment sent");
}
@Override
public void sendHTMLEmail() {
logger.info("HTML email sending start");
MimeMessage mimeMessage = javaMailSender.createMimeMessage();
try {
// Set multipart mime message true
MimeMessageHelper mimeMessageHelper = new MimeMessageHelper(mimeMessage,
true);
mimeMessageHelper.setTo("[email protected]");
mimeMessageHelper.setSubject("Spring Boot=> Sending HTML email");
String html = "<h3>Dear Manish</h3></br>"
+ "<p>Many many congratulation for joining "
+ "<strong>Websparrow.org Team</strong>.</p>" + "</br></br>"
+ "<p>You are entitled for <code>Rs.5000</code> "
+ "as joning bonus.</p>";
mimeMessageHelper.setText(html, true);
javaMailSender.send(mimeMessage);
} catch (MessagingException e) {
logger.error("Exeception=>sendHTMLEmail ", e);
}
logger.info("HTML email sent");
}
}
6. Calling Email Service
Open your Spring Boot starter class and autowire the EmailService
interface as shown below:
package org.websparrow;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.CommandLineRunner;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.websparrow.service.EmailService;
@SpringBootApplication
public class SpringBootEmailApp implements CommandLineRunner {
private static final Logger logger = LoggerFactory
.getLogger(SpringBootEmailApp.class);
@Autowired
private EmailService emailService;
public static void main(String[] args) {
SpringApplication.run(SpringBootEmailApp.class, args);
}
@Override
public void run(String... args) throws Exception {
logger.info("Sending an email initiated");
emailService.sendTextEmail();
emailService.sendEmailWithAttachment();
emailService.sendHTMLEmail();
}
}
You might be encountered the javax.mail.AuthenticationFailedException
Here is the log from STS console.
. ____ _ __ _ _
/\\ / ___'_ __ _ _(_)_ __ __ _ \ \ \ \
( ( )\___ | '_ | '_| | '_ \/ _` | \ \ \ \
\\/ ___)| |_)| | | | | || (_| | ) ) ) )
' |____| .__|_| |_|_| |_\__, | / / / /
=========|_|==============|___/=/_/_/_/
[32m :: Spring Boot :: [39m [2m (v2.2.6.RELEASE)[0;39m
.......
.............
21:36:31.443 [restartedMain] DEBUG org.websparrow.SpringBootEmailApp - Running with Spring Boot v2.2.6.RELEASE, Spring v5.2.5.RELEASE
21:36:39.047 [restartedMain] INFO org.websparrow.SpringBootEmailApp - Started SpringBootEmailApp in 8.328 seconds (JVM running for 9.533)
21:36:39.049 [restartedMain] INFO org.websparrow.SpringBootEmailApp - Sending an email initiated
21:36:39.049 [restartedMain] INFO o.w.service.impl.EmailServiceImpl - Simple Email sending start
21:36:49.658 [restartedMain] INFO o.w.service.impl.EmailServiceImpl - Simple Email sent
21:36:49.658 [restartedMain] INFO o.w.service.impl.EmailServiceImpl - Sending email with attachment start
21:36:56.433 [restartedMain] INFO o.w.service.impl.EmailServiceImpl - Email with attachment sent
21:36:56.434 [restartedMain] INFO o.w.service.impl.EmailServiceImpl - HTML email sending start
21:37:00.794 [restartedMain] INFO o.w.service.impl.EmailServiceImpl - HTML email sent
And the screenshot of the mail that delivered to my email address for testing.
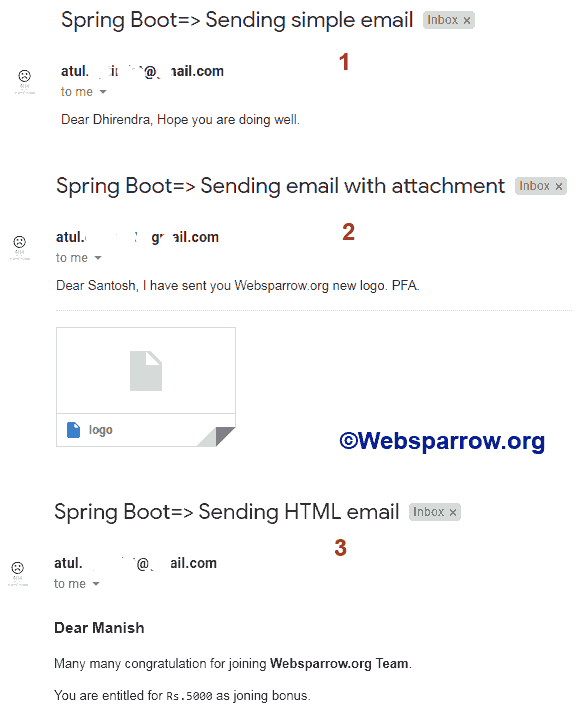
Download Source Code: how-to-send-email-using-spring-boot.zip
References
- Example of sending email in Java using Gmail SMTP
- Send email with attachment in Java using Gmail
- Email- Spring Boot Doc
- Sending Email- Spring Boot features