Bean Scopes in Spring
Spring offers several bean scopes, each with its advantages and disadvantages. This post will delve into the various bean scopes, explore their pros and cons, and discuss which scope developers should prefer and why.
What is Bean Scope?
In Spring, a bean is an object instantiated, assembled, and managed by the Spring IoC container. Bean scope determines the lifecycle and visibility of these beans within the Spring container. Spring offers several bean scopes, including singleton, prototype, request, session, and application scopes.
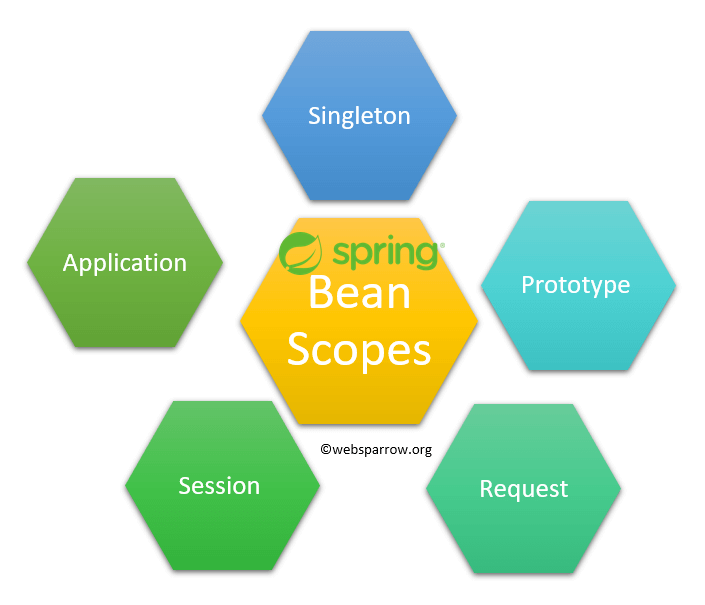
Similar Post: Spring Bean Life Cycle Management Example
1. Singleton Scope
Pros:
- Default scope in Spring.
- One instance per Spring IoC container.
- Suitable for stateless beans.
- Promotes performance as only one instance is created.
Cons:
- Not suitable for stateful beans where each client needs its own instance.
- Can lead to thread safety issues if not handled properly.
Code Snippet:
import org.springframework.context.annotation.Scope;
import org.springframework.stereotype.Component;
@Component
@Scope("singleton")
public class SingletonBean {
// Class implementation
}
2. Prototype Scope
Pros:
- A new instance is created each time the bean is requested.
- Suitable for stateful beans where each client needs its own instance.
- No synchronization issues as instances are independent.
Cons:
- Can lead to performance overhead due to frequent object creation.
- Requires proper resource management to avoid memory leaks.
Code Snippet:
import org.springframework.context.annotation.Scope;
import org.springframework.stereotype.Component;
@Component
@Scope("prototype")
public class PrototypeBean {
// Class implementation
}
3. Request Scope
Pros:
- One instance per HTTP request.
- Suitable for web applications to maintain state during a single request.
- Prevents thread safety issues in a multi-threaded environment.
Cons:
- Limited to web applications.
- Increased memory consumption for large-scale applications with numerous concurrent requests.
Code Snippet:
import org.springframework.context.annotation.Scope;
import org.springframework.stereotype.Component;
import org.springframework.web.context.WebApplicationContext;
import org.springframework.web.context.annotation.RequestScope;
@Component
@RequestScope
public class RequestScopedBean {
// Class implementation
}
4. Session Scope
Pros:
- One instance per HTTP session.
- Maintains state across multiple requests from the same client.
- Useful for storing user-specific data in web applications.
Cons:
- Consumes memory as long as the session is active.
- Not suitable for applications with heavy session usage or limited memory resources.
Code Snippet:
import org.springframework.context.annotation.Scope;
import org.springframework.stereotype.Component;
import org.springframework.web.context.WebApplicationContext;
import org.springframework.web.context.annotation.SessionScope;
@Component
@SessionScope
public class SessionScopedBean {
// Class implementation
}
5. Application Scope
Pros:
- One instance per ServletContext.
- Useful for storing global objects accessible throughout the application.
- Provides a centralized location for shared resources.
Cons:
- Limited to web applications.
- Potential for memory leaks if objects are not properly managed.
Code Snippet:
import org.springframework.context.annotation.Scope;
import org.springframework.stereotype.Component;
import org.springframework.web.context.WebApplicationContext;
import org.springframework.web.context.annotation.ApplicationScope;
@Component
@ApplicationScope
public class ApplicationScopedBean {
// Class implementation
}
Which Scope to Prefer?
The choice of bean scope depends on the specific requirements and characteristics of the application:
- Singleton: Use when a single instance of the bean is sufficient for the entire application and when performance optimization is crucial.
- Prototype: Suitable for stateful beans where each client needs its own instance or when the bean’s state needs to be reset on each request.
- Request and Session: Ideal for web applications to maintain state across HTTP requests. Choose request scope for short-lived state and session scope for longer-lived state.
- Application: Use when global objects or resources need to be shared across the entire application.
Summary
Understanding Spring bean scope is essential for designing scalable, maintainable, and efficient applications. Each scope has its advantages and limitations, and the choice depends on the specific requirements of the application. By selecting the appropriate scope, you can optimize resource utilization and ensure the desired behavior of their Spring beans.