Spring Boot RESTful Web Service Example
On this page, you will learn to build a RESTful web service using Spring Boot. Spring Boot makes the task easier to build a REST web service. You don’t need to do lots of configuration and that’s the power of Spring Boot. In short, you will do zero configuration.
What you’ll build
In this example, we will build a simple RESTful web service that will accept HTTP GET
requests at:
http://localhost:8888/student/2
and it will produce the data in JSON format.
{
"rollNo": 2,
"name": "Sandeep Sharma",
"course": "BCA",
"college": "MIT"
}
Technologies Used
Find the list of all technologies used in this application.
- Spring Tool Suite 4
- JDK 8
- Maven 3
- Spring-boot 2.0.5.RELEASE
Dependencies Required
Building a RESTful web service in Spring Boot, you need the following dependencies. Add the following code in your pom.xml.
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.websparrow</groupId>
<artifactId>sring-boot-rest-example</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>jar</packaging>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.0.5.RELEASE</version>
<relativePath />
</parent>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
Project Structure
Final project structure of our application in STS ide will look like as follows.
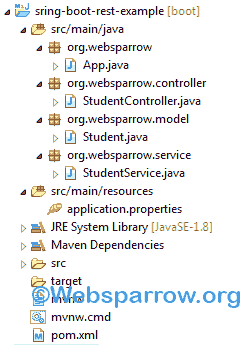
Model Class
Create a Student
model class along with its attributes and a parameterized constructor will all attributes.
package org.websparrow.model;
public class Student {
// Generate Getters and Setters...
private Integer rollNo;
private String name;
private String course;
private String college;
public Student(Integer rollNo, String name, String course, String college) {
this.rollNo = rollNo;
this.name = name;
this.course = course;
this.college = college;
}
}
Service Class
StudentService
class provides the services to the controller based on the request either you want all student data or one and @Service
annotation indicates that an annotated class is a “Service”.
package org.websparrow.service;
import java.util.Hashtable;
import org.springframework.stereotype.Service;
import org.websparrow.model.Student;
@Service
public class StudentService {
Hashtable<Integer, Student> ht = new Hashtable<>();
public StudentService() {
Student student = new Student(1, "Atul Rai", "MCA", "RSMT");
ht.put(1, student);
student = new Student(2, "Sandeep Sharma", "BCA", "MIT");
ht.put(2, student);
student = new Student(3, "Prince", "B.Sc", "AKG");
ht.put(3, student);
student = new Student(4, "Abhinav", "B.Pharma", "Amity");
ht.put(4, student);
}
public Student findByRollNo(Integer rollNo) {
if (ht.containsKey(rollNo)) {
return ht.get(rollNo);
} else {
return null;
}
}
public Hashtable<Integer, Student> findAll() {
return ht;
}
}
Controller Class
In Spring’s approach to building RESTful web services, HTTP requests are handled by a controller. These components are easily identified by the @RestController
annotation, and the StudentController
below handles GET
requests.
Post you may like: Spring MVC @Controller, @RequestMapping, @RequestParam, and @PathVariable Annotation Example
package org.websparrow.controller;
import java.util.Hashtable;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import org.websparrow.model.Student;
import org.websparrow.service.StudentService;
@RestController
@RequestMapping("/student")
public class StudentController {
@Autowired
StudentService studentService;
@RequestMapping("/{rollNo}")
public Student getOne(@PathVariable("rollNo") Integer rollNo) {
return studentService.findByRollNo(rollNo);
}
@RequestMapping("/all")
public Hashtable<Integer, Student> getAll() {
return studentService.findAll();
}
}
Run it
Create an App
class and execute it.
package org.websparrow;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class App {
public static void main(String[] args) {
SpringApplication.run(App.class, args);
}
}
Test the Service
Now that the service is up, visit http://localhost:8888/student/all, where you see:
{
"1": {
"rollNo": 1,
"name": "Atul Rai",
"course": "MCA",
"college": "RSMT"
},
"2": {
"rollNo": 2,
"name": "Sandeep Sharma",
"course": "BCA",
"college": "MIT"
},
"3": {
"rollNo": 3,
"name": "Prince",
"course": "B.Sc",
"college": "AKG"
},
"4": {
"rollNo": 4,
"name": "Abhinav",
"course": "B.Pharma",
"college": "Amity"
}
}
if you want to fetch the data of specific student visit http://localhost:8888/student/2, you will get:
{
"rollNo": 2,
"name": "Sandeep Sharma",
"course": "BCA",
"college": "MIT"
}
Note: If the embedded Tomcat was unable to start, add the below code in your application.properties file to change the Tomcat server port.
server.port=8888
References
- Spring @Autowired annotation example
- Spring MVC @Controller, @RequestMapping, @RequestParam, and @PathVariable Annotation Example
- Building a RESTful Web Service
Download Source Code: spring-boot-restful-web-service-example.zip