Spring Boot- Display image from database and classpath
On this page, we are going to learn how to display image from the database and classpath location using Spring Boot RESTful web service. Returning image/media data with Spring Boot REST application, we have multiple options:
- Using the
ResponseEntity
- Using the
HttpServletResponse
Producing an image/media using REST service, Spring Framework has MediaType
class inside the org.springframework.http
package. We can use it according to our need like MediaType.IMAGE_JPEG
or MediaType.IMAGE_JPEG_VALUE
.
Similar Post: How to fetch image from database using Spring MVC
1. Using the ResponseEntity
You can return an image as byte[]
wrapped in the ResponseEntity
. We need to define the return type of the method as ResponseEntity<byte[]>
and create returning ResponseEntity
object in the method body.
1.1 From Database
Use the JPA to fetch the details from the database. get the image bytes, set the content type and pass the image byte array to the ResponseEntity
body.
@GetMapping("database/{id}")
public ResponseEntity<byte[]> fromDatabaseAsResEntity(@PathVariable("id") Integer id)
throws SQLException {
Optional<PrimeMinisterOfIndia> primeMinisterOfIndia = imageRepository.findById(id);
byte[] imageBytes = null;
if (primeMinisterOfIndia.isPresent()) {
imageBytes = primeMinisterOfIndia.get().getPhoto().getBytes(1,
(int) primeMinisterOfIndia.get().getPhoto().length());
}
return ResponseEntity.ok().contentType(MediaType.IMAGE_JPEG).body(imageBytes);
}
1.2 From Classpath
Pass the image classpath location to ClassPathResource
constructor, get the image bytes by calling StreamUtils.copyToByteArray(imageFile.getInputStream())
method and pass the image byte array to the ResponseEntity
body.
@GetMapping(value = "classpath")
public ResponseEntity<byte[]> fromClasspathAsResEntity() throws IOException {
ClassPathResource imageFile = new ClassPathResource("pm-india/modi.jpg");
byte[] imageBytes = StreamUtils.copyToByteArray(imageFile.getInputStream());
return ResponseEntity.ok().contentType(MediaType.IMAGE_JPEG).body(imageBytes);
}
2. Using the HttpServletResponse
Serving images/media using HttpServletResponse
is the most basic approach. It is the pure Servlet
implementation and used from a decade.
2.1 From Database
@GetMapping(value = "database1/{id}", produces = MediaType.IMAGE_JPEG_VALUE)
public void fromDatabaseAsHttpServResp(@PathVariable("id") Integer id, HttpServletResponse response)
throws SQLException, IOException {
Optional<PrimeMinisterOfIndia> primeMinisterOfIndia = imageRepository.findById(id);
if (primeMinisterOfIndia.isPresent()) {
Blob image = primeMinisterOfIndia.get().getPhoto();
StreamUtils.copy(image.getBinaryStream(), response.getOutputStream());
}
}
2.2 From Classpath
@GetMapping(value = "classpath1", produces = MediaType.IMAGE_JPEG_VALUE)
public void fromClasspathAsHttpServResp(HttpServletResponse response) throws IOException {
ClassPathResource imageFile = new ClassPathResource("pm-india/vajpayee.jpg");
StreamUtils.copy(imageFile.getInputStream(), response.getOutputStream());
}
Now let’s jump to the actual implementation of Spring Boot- Display image from database and classpath.
What we’ll build
In this example, we will create a Spring Boot application which fetches the image from the database using the ID and renders it via calling REST API.
Similarly, fetches the image from the classpath location and renders it via calling REST API.
Technologies Used
Find the list of all technologies used in this application.
- Spring Tool Suite 4
- JDK 8
- Maven 3
- Spring-boot 2.1.6.RELEASE
- MySQL Database
Project Structure
Final project structure of our application in STS ide will look like as follows.
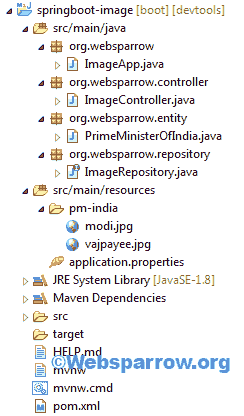
Dependencies Required
Add the following dependencies to the pom.xmlto work with Spring Boot REST application.
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<scope>runtime</scope>
</dependency>
</dependencies>
application.properties
application.properties file contains the database connection strings so the application can communicate with the database.
# MySQL database connection strings
spring.datasource.url=jdbc:mysql://localhost:3306/demo
spring.datasource.username=root
spring.datasource.password=root
# JPA property settings
spring.jpa.hibernate.ddl-auto=update
spring.jpa.properties.hibernate.show_sql=true
Entity
The entity class is the skeleton of the table.
package org.websparrow.entity;
@Entity
@Table(name = "pm_of_india")
public class PrimeMinisterOfIndia {
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Integer id;
private Blob photo;
// Generate Getters and Setters...
}
Repository
ImageRepository
extends the JpaRepository
of Spring Data to query with the database and retrieves the images.
package org.websparrow.repository;
import org.springframework.data.jpa.repository.JpaRepository;
import org.springframework.stereotype.Repository;
import org.websparrow.entity.PrimeMinisterOfIndia;
@Repository
public interface ImageRepository extends JpaRepository<PrimeMinisterOfIndia, Integer> {
}
Controller
The ImageController
class exposes the REST endpoint through which we can get the images from the database and classpath location as well.
package org.websparrow.controller;
import java.io.IOException;
import java.sql.Blob;
import java.sql.SQLException;
import java.util.Optional;
import javax.servlet.http.HttpServletResponse;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.core.io.ClassPathResource;
import org.springframework.http.MediaType;
import org.springframework.http.ResponseEntity;
import org.springframework.util.StreamUtils;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import org.websparrow.entity.PrimeMinisterOfIndia;
import org.websparrow.repository.ImageRepository;
@RestController
@RequestMapping("image")
public class ImageController {
@Autowired
private ImageRepository imageRepository;
// Return the image from the database using ResponseEntity
@GetMapping("database/{id}")
public ResponseEntity<byte[]> fromDatabaseAsResEntity(@PathVariable("id") Integer id) throws SQLException {
Optional<PrimeMinisterOfIndia> primeMinisterOfIndia = imageRepository.findById(id);
byte[] imageBytes = null;
if (primeMinisterOfIndia.isPresent()) {
imageBytes = primeMinisterOfIndia.get().getPhoto().getBytes(1,
(int) primeMinisterOfIndia.get().getPhoto().length());
}
return ResponseEntity.ok().contentType(MediaType.IMAGE_JPEG).body(imageBytes);
}
// Return the image from the database using HttpServletResponse
@GetMapping(value = "database1/{id}", produces = MediaType.IMAGE_JPEG_VALUE)
public void fromDatabaseAsHttpServResp(@PathVariable("id") Integer id, HttpServletResponse response)
throws SQLException, IOException {
Optional<PrimeMinisterOfIndia> primeMinisterOfIndia = imageRepository.findById(id);
if (primeMinisterOfIndia.isPresent()) {
Blob image = primeMinisterOfIndia.get().getPhoto();
StreamUtils.copy(image.getBinaryStream(), response.getOutputStream());
}
}
// Return the image from the classpath location using ResponseEntity
@GetMapping(value = "classpath")
public ResponseEntity<byte[]> fromClasspathAsResEntity() throws IOException {
ClassPathResource imageFile = new ClassPathResource("pm-india/modi.jpg");
byte[] imageBytes = StreamUtils.copyToByteArray(imageFile.getInputStream());
return ResponseEntity.ok().contentType(MediaType.IMAGE_JPEG).body(imageBytes);
}
// Return the image from the classpath location using HttpServletResponse
@GetMapping(value = "classpath1", produces = MediaType.IMAGE_JPEG_VALUE)
public void fromClasspathAsHttpServResp(HttpServletResponse response) throws IOException {
ClassPathResource imageFile = new ClassPathResource("pm-india/vajpayee.jpg");
StreamUtils.copy(imageFile.getInputStream(), response.getOutputStream());
}
}
Run it
Now all is well, create an ImageApp
class and run it.
package org.websparrow;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class ImageApp {
public static void main(String[] args) {
SpringApplication.run(ImageApp.class, args);
}
}
Test it
When the application successfully started, open your favorite web browser and hit the below URL’s:
1. From database as ResponseEntity → http://localhost:8080/image/database/{id}
2. From database as HttpServletResponse → http://localhost:8080/image/database1/{id}
3. From classpath as ResponseEntity → http://localhost:8080/image/classpath
4. From classpath as HttpServletResponse → http://localhost:8080/image/classpath1
Attention: In this example, we have not written any logic to insert images into the database. Inserting an image into the database is done manually for the below table structure:
CREATE TABLE `pm_of_india` ( `id` int(11) NOT NULL, `photo` longblob, PRIMARY KEY (`id`) );
The SQL query to insert the images:
INSERT INTO pm_of_india(id,photo) VALUES(101,LOAD_FILE('E:/Images/modi.jpg'));
Download Source Code: spring-boot-display-image-from-database-and-classpath.zip
References
- Spring Boot RESTful Web Service with JPA and MySQL
- How to fetch image from database using Spring MVC
- Class ResponseEntity<T>