Getting Started with Spring Boot and MongoDB
In this article, we’ll learn how to connect the Spring Boot application with the MongoDB database and access data from the MongoDB database. MongoDB is a cross-platform document-oriented database commonly known as a NoSQL database. MongoDB is a document database, which means it stores data in JSON-like documents.
Spring provides inbuilt interfaces and annotations that help to access and connect with MongoDB database like:
MongoRepository<T, ID>
is an interface that provides basic CRUD methods similar to CrudRepository Interface.@Document
annotation helps to define the name of the document similar to the@Table
annotation.
What we’ll build
On this page, we’ll create a brand new Spring Boot application and connect the application with the MongoDB database to insert and fetch the data from the database.
Technology Used
Find the list of all tools and technologies used in this application.
- IntelliJ IDEA
- JDK 8
- Spring Boot 2.3.3.RELEASE
- MongoDB 4.4.1 Database
- Maven 3.6
Dependencies Required
Accessing data with MongoDB, the following dependencies must be available in the application classpath.
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.3.3.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>org.websparrow</groupId>
<artifactId>springboot-mongodb</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>springboot-mongodb</name>
<properties>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-mongodb</artifactId>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
Project Structure
The final project structure of our application in IntelliJ IDEA IDE will look like as follows:
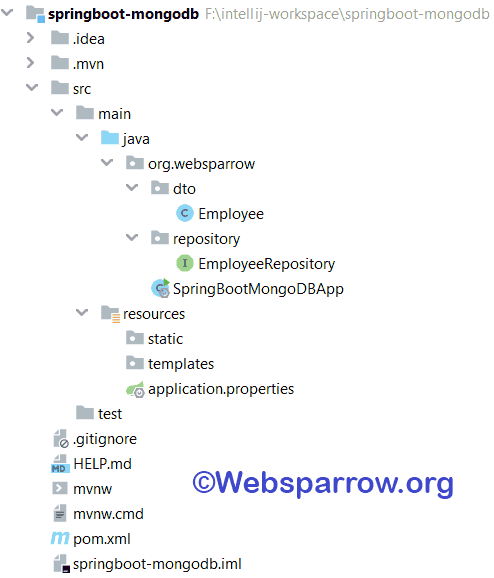
Define a Simple Document (POJO)
Create a simple Employee
class with its attributes and generates the getters and setters and parameterized constructors.
package org.websparrow.dto;
import org.springframework.data.annotation.Id;
import org.springframework.data.mongodb.core.mapping.Document;
@Document
public class Employee {
@Id
private String id;
private String firstName;
private String lastName;
private Character gender;
private int age;
// Generate Getters and Setters...
public Employee() {
}
public Employee(String firstName, String lastName, Character gender, int age) {
this.firstName = firstName;
this.lastName = lastName;
this.gender = gender;
this.age = age;
}
@Override
public String toString() {
return "Employee{" +
"id='" + id + '\'' +
", firstName='" + firstName + '\'' +
", lastName='" + lastName + '\'' +
", gender=" + gender +
", age=" + age +
'}';
}
}
By default, Spring Data MongoDB uses the class name to create the collection name in the MongoDB database. We can use the
@Document(collection = "employees")
annotation on the class to change the collection name.
Create Repository
In order to query with the MongoDB database, the EmployeeRepository
interface will extend the MongoRepository<T, ID>
interface which provides the basic CRUD operation methods and we can also create our Derived findBy Query Methods.
package org.websparrow.repository;
import org.springframework.data.mongodb.repository.MongoRepository;
import org.springframework.stereotype.Repository;
import org.websparrow.dto.Employee;
import java.util.List;
@Repository
public interface EmployeeRepository extends MongoRepository<Employee, String> {
List<Employee> findByFirstName(String firstName);
List<Employee> findByGender(Character gender);
}
Define Database Connection Strings
application.properties file comes in the picture to define the MongoDB database connection strings.
#Database connection strings
spring.data.mongodb.host=localhost
spring.data.mongodb.port=27017
spring.data.mongodb.database=springboot
spring.data.mongodb.username={db-username}
spring.data.mongodb.password={your-secret-password}
By default, Spring Data try to connect the application at localhost:27017/test if we don’t define the connection parameters.
Execute it
The final step is executing the application. To do that, create a Spring Boot runner class i.e. SpringBootMongoDBApp
which implements the CommandLineRunner
interface. Overrides it’s run()
method and call the required methods of the EmployeeRepository
interface.
package org.websparrow;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.CommandLineRunner;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.websparrow.dto.Employee;
import org.websparrow.repository.EmployeeRepository;
import java.util.List;
@SpringBootApplication
public class SpringBootMongoDBApp implements CommandLineRunner {
public static void main(String[] args) {
SpringApplication.run(SpringBootMongoDBApp.class, args);
}
@Autowired
private EmployeeRepository employeeRepository;
@Override
public void run(String... args) throws Exception {
// Save the employees
employeeRepository.save(new Employee("Atul", "Rai", 'M', 28));
employeeRepository.save(new Employee("Pallavi", "Tripathi", 'F', 25));
employeeRepository.save(new Employee("Manish", "Fartiyal", 'M', 30));
employeeRepository.save(new Employee("Lucifer", "**", 'F', 33));
// Fetch the all employees
List<Employee> employees = employeeRepository.findAll();
System.out.println("============ List of all employees ============");
System.out.println(employees);
// Fetch the all employees by first name
List<Employee> employeesByFirstName = employeeRepository.findByFirstName("Manish");
System.out.println("============ List of all employees by first name ============");
System.out.println(employeesByFirstName);
// Fetch the all employees by gender
List<Employee> employeesByGender = employeeRepository.findByGender('F');
System.out.println("============ List of all employees by gender ============");
System.out.println(employeesByGender);
}
}
Output
After the successful execution, you will find the below output on your IDE console log.
============ List of all employees ============
[
Employee{id='5f66fcebf00ba2568d60b7e0', firstName='Atul', lastName='Rai', gender=M, age=28}, Employee{id='5f66fcebf00ba2568d60b7e1', firstName='Pallavi', lastName='Tripathi', gender=F, age=25}, Employee{id='5f66fcebf00ba2568d60b7e2', firstName='Manish', lastName='Fartiyal', gender=M, age=30}, Employee{id='5f66fcebf00ba2568d60b7e3', firstName='Lucifer', lastName='**', gender=F, age=33}
]
============ List of all employees by first name ============
[
Employee{id='5f66fcebf00ba2568d60b7e2', firstName='Manish', lastName='Fartiyal', gender=M, age=30}
]
============ List of all employees by gender ============
[
Employee{id='5f66fcebf00ba2568d60b7e1', firstName='Pallavi', lastName='Tripathi', gender=F, age=25}, Employee{id='5f66fcebf00ba2568d60b7e3', firstName='Lucifer', lastName='**', gender=F, age=33}
]
To verify it, you can also check the MongoDB database.
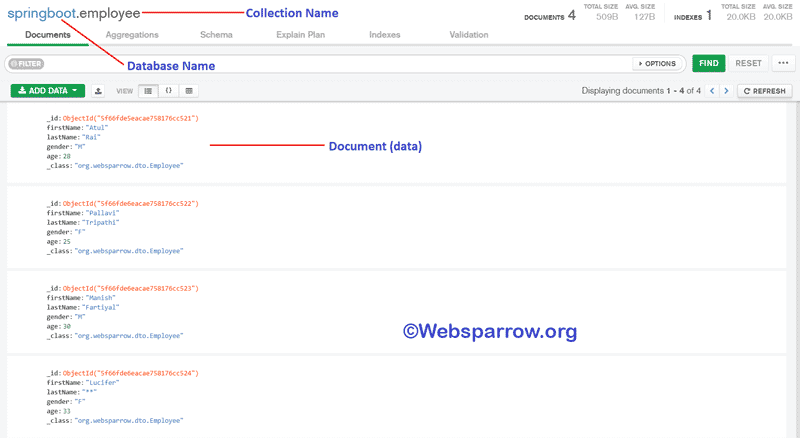
Download Source Code: getting-started-with-spring-boot-and-mongodb.zip