Versioning of REST API in Spring Boot: Types and Benefits
In this blog, we’ll explore the various types of API versioning in Spring Boot and the benefits they offer.
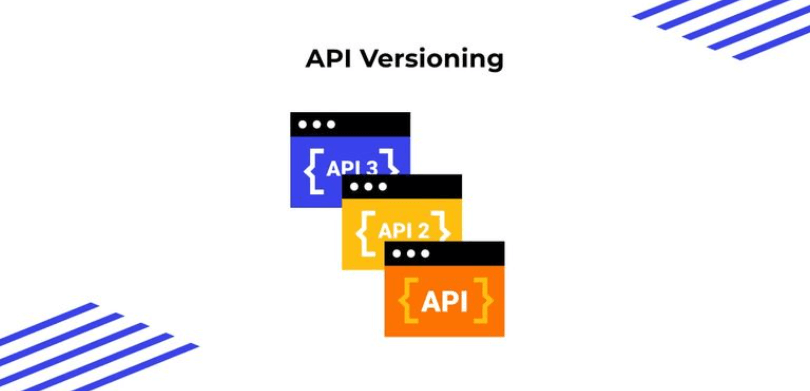
Why API Versioning?
API versioning allows you to make changes to your API while ensuring that existing clients are not affected. Without proper versioning, any change to the API can potentially break client applications, leading to unexpected issues and disruptions. Versioning is essential for maintaining backward compatibility and a smooth transition as your API evolves.
Types of API Versioning in Spring Boot
Spring Boot provides several approaches for versioning your REST APIs. Let’s delve into some of the commonly used methods:
1. URI Versioning
URI versioning involves including the version number in the URL path. This is one of the most straightforward methods for versioning REST APIs. For example, you might have endpoints like /v1/resource
and /v2/resource
to indicate different versions of the same resource.
GET /v1/resource
GET /v2/resource
Benefits of URI versioning:
- Explicit: It’s clear which version of the API you’re accessing from the URL.
- Cacheability: Caching is easier because different versions have distinct URLs.
- Simplicity: Easy to implement and understand.
Drawbacks of URI versioning:
- Cluttered URIs: As versions accumulate, the URLs can become complex and hard to read.
- Semantic issues: Versions in the URI don’t provide information about the content or purpose of the version.
2. Request Header Versioning
In this approach, the version is specified in a custom request header. The actual URI remains the same, but the version is defined in the Accept
or Custom
header.
GET /api/resource
Host: example.com
Accept: application/vnd.myapp.v1+json
In this example, the Accept
header includes a custom media type with the version (e.g., v1
) embedded in it.
Benefits of request header versioning:
- Clean URIs: The URI remains concise and doesn’t clutter with version information.
- Separation of concerns: Versioning is a concern of the client, not the server.
Drawbacks of request header versioning:
- Client complexity: Clients need to specify the version in the request header, which may not be as intuitive for some developers.
- Cacheability: Caching becomes challenging because the URI doesn’t change.
3. Media Type (MIME) Versioning
In this method, the version is indicated through the media type (MIME type) in the Accept
header. Different versions of the API are represented as different media types.
GET /api/resource
Host: example.com
Accept: application/vnd.myapp.v1+json
In this example, the Accept
header specifies the custom media type application/vnd.myapp.v1+json
, indicating version 1 of the API.
Benefits of media type versioning:
- Clean URIs: Like request header versioning, the URI remains clean.
- Semantic clarity: The media type provides a semantic indication of the version.
- Cacheability: Caching can be more efficient since different versions have distinct media types.
Drawbacks of media type versioning:
- Complex headers: The headers can become complex when dealing with multiple versions.
- Learning curve: Developers may need to understand the media types for each version.
4. Custom Request Parameter Versioning
This approach involves specifying the version as a custom query parameter in the URL.
GET /resource?version=1
Benefits of custom request parameter versioning:
- Simple for clients: Clients can specify the version directly in the URL, making it clear and easy to use.
- Clear separation of versions: Different versions are cleanly separated.
Drawbacks of custom request parameter versioning:
- Cluttered URIs: Like URI versioning, the URL can become cluttered with version information.
- Semantic issues: Versions in the query parameter don’t provide information about the content or purpose of the version.
Benefits of API Versioning
Now that we’ve covered the different types of API versioning in Spring Boot, let’s explore the advantages they offer:
- Backward Compatibility: API versioning ensures that existing clients continue to work as expected, even as the API evolves.
- Improved Development Workflow: Developers can work on new features and updates in parallel, knowing that changes won’t disrupt existing clients.
- Clear Documentation: Versioning makes it clear which version of the API a client should use, simplifying documentation and onboarding for new developers.
- Graceful Deprecation: Older versions can be deprecated and eventually retired without impacting current clients.
- Better Error Handling: With versioning, error responses can include information about the supported versions, helping clients handle issues gracefully.
- Caching Optimization: Different URIs or media types for each version allow for more efficient caching of responses.