Spring @Value Annotation Example
In this guide, we’ll show you some tips & tricks of Spring @Value
annotation. @Value
annotation is used to assign values to variables and method arguments. It was introduced in Spring’s version 3.0.
@Value
annotation is widely used to get values from the properties file in a Spring/Boot application. It is very useful when we develop a microservice-based application and fetching the configurations from a properties file.
Similar Posts:
- Spring @Autowired annotation example
- Spring 5 @Qualifier annotation example
- Spring @RestController, @GetMapping, @PostMapping, @PutMapping, and @DeleteMapping Annotation Example
1. Set Default/Static Value
We can assign a class member variable with default/static value using @Value
annotation.
// Set static string value
@Value("Success")
private String stringValue;
// Set default boolean value
@Value("true")
private boolean booleanValue;
// Set static integer value
@Value("101")
private int integerValue;
2. Get Value from Properties File
@Value
annotation is not only used to set static/default values, but it can be also used to read values from the properties file.
2.1 Get String Value
We know a properties file contains the values in the form of key and value pair.
emp.message=Hi, I'm Manish and this message picked from a properties file.
To get the value of key emp.message and set it to the class member variable, Spring gives us some syntax i.e. double quotes » dollar sign » curly braces » and inside the curly braces your key that we’ve defined in the properties file.
@Value("${emp.message}")
private String empMessage;
By default @Value annotation searches the key in application.properties file in a Spring Boot application.
Question: Hold a second, what happens when the key is missing or we forgot to define it in properties file that we’ve mentioned in
@Value
annotation?Answer: It will throw
BeanCreationException
: Error creating bean with name ‘employeeController‘: Injection of autowired dependencies failed; nested exception isjava.lang.IllegalArgumentException
: Could not resolve placeholder ‘emp.message‘ in value"${emp.message}"
.
2.2 Set Default Value when KEY is Missing
The above exception can be handled by setting the default value when a key is missing or not found in the properties file.
The syntax is almost the same as mentioned above, the only change is we have to set our default message followed by a colon (:)
just after the key.
double quotes » dollar sign » curly braces » key » colon(:) » your default message.
@Value("${emp.message.default: Hi, I'm employee default message.}")
private String degaultEmpMessage;
2.3 Get List Value
@Value
can assign the comma-separated values to a List
. Suppose we have a key emp.list which holds the names of employees separated by a comma in the properties file.
emp.list=Atul, Manish, Santosh, Dhirendra
The emp.list values can be assigned to list like:
@Value("${emp.list}")
private List<String> empList;
2.4 Get Map Value
We can also set a key value in the form of key and value pairs inside the properties file and assign those values to a Map
.
emp.details={firstName: 'Manish', lastName: 'Fartiyal', company: 'Websparrow.org India Pvt. Ltd.'}
It can be assigned to a Map
using Spring Expression Language (SpEL). In this, we have to surround all the above syntax by a HASH (#)
symbol.
@Value("#{${emp.details}}")
private Map<String, String> empDetails;
Lets just to the actual piece of coding and build a running application.
3. application.properties
This is our default properties file where we set the values in the key-value pair.
emp.message=Hi, I'm Manish and this message picked from properties file.
emp.list=Atul, Manish, Santosh, Dhirendra
emp.details={firstName: 'Manish', lastName: 'Fartiyal', company: 'Websparrow.org India Pvt. Ltd.'}
4. Controller
The EmployeeController
class where we get the values from properties file using @Value
annotation and expose a REST endpoint to display on the browser.
package org.websparrow.controller;
import java.util.List;
import java.util.Map;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class EmployeeController {
@Value("Hi, My name is Atul.")
private String staticMessage;
@Value("${emp.message}")
private String empMessage;
@Value("${emp.message.default: Hi, I'm employee default message.}")
private String defaultEmpMessage;
@Value("${emp.list}")
private List<String> empList;
@Value("#{${emp.details}}")
private Map<String, String> empDetails;
@GetMapping("/employee")
public String employeeInfo() {
return toString();
}
@Override
public String toString() {
return "Static Message= " + staticMessage + "</br>"
+ " Employee Message=" + empMessage + "</br>"
+ "Default employee message when key is not found= " + defaultEmpMessage + "</br>"
+ " List of all employees= " + empList + "</br>"
+ " Size of employees= " + empList.size() + "</br>"
+ "Empoyee details= " + empDetails;
}
}
5. Run the application
package org.websparrow;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class SpringValueAnnotationApp {
public static void main(String[] args) {
SpringApplication.run(SpringValueAnnotationApp.class, args);
}
}
6. Test the application
To test the application, start the Spring Boot application by executing the above class and hit the below API in your favorite web browser:
API: http://localhost:8080/employee
And you will get the following response:
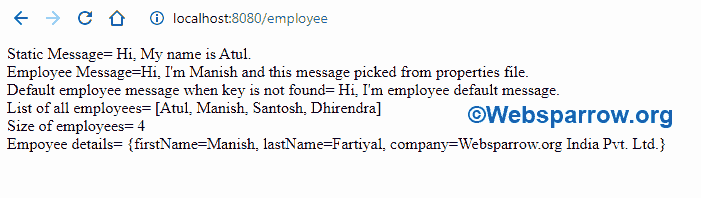