Spring Boot Security- Remember Me Example
This page will guide you on how to configure Spring Boot Security- Remember Me functionality in the application. Remember Me feature help users to access the application without re-login. Mostly, we use the checkbox on the login page to enable it.
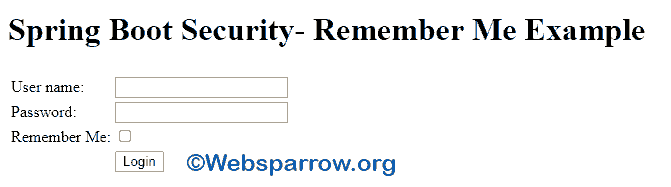
Spring Security- Remember Me feature stores user’s login information into the web browser cookies which able to identify the user across multiple sessions.
Note: This example is based on the Simple Hash-Based Token Approach which uses the hashing technique to create the unique token. In this technique, a token is created using the key, expiry date, password, and username. Cookie being composed as follows:
base64(username + ":" + expirationTime + ":" + md5Hex(username + ":" + expirationTime + ":" password + ":" + key)) username: As identifiable to the UserDetailsService password: That matches the one in the retrieved UserDetails expirationTime: The date and time when the remember-me token expires, expressed in milliseconds key: A private key to prevent modification of the remember-me token
We have one more approach i.e. Persistent Token Approach. In this approach, we will store the token into the database. Table “persistent_logins” will be created to store the login token and series.
Technologies Used
Find the list of all technologies used in this application.
- Spring Boot 2.1.2.RELEASE
- Spring Security 5.1.4.RELEASE
- JDK 8
- Maven 3
- STS 3
- Embedded Tomcat Server
Dependencies Required
To resolve the JAR dependency, add the following code to your pom.xml.
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- JSP compiler jar -->
<dependency>
<groupId>org.apache.tomcat.embed</groupId>
<artifactId>tomcat-embed-jasper</artifactId>
<scope>provided</scope>
</dependency>
</dependencies>
Project Structure
The final project structure of our application in STS IDE will look like as follows.
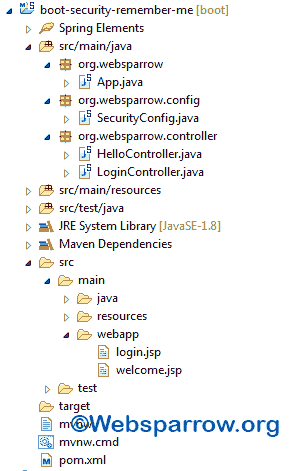
1. Login Form
Create a simple custom login form and add a checkbox to enable Remember Me feature.
<form action="login" method="post">
<table>
<tr style="color: red;">
<td></td>
<td>${SPRING_SECURITY_LAST_EXCEPTION.message}</td>
</tr>
<tr>
<td>User name:</td>
<td><input type="text" name="username"></td>
</tr>
<tr>
<td>Password:</td>
<td><input type="password" name="password"></td>
</tr>
<tr>
<td>Remember Me:</td>
<td><input type="checkbox" name="remember-me" /></td>
</tr>
<tr>
<td></td>
<td><input type="submit" value="Login"></td>
</tr>
</table>
</form>
2. Security Configuration
Spring security configuration file is used to implement the remember me functionality as given below:
package org.websparrow.config;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.authentication.builders.AuthenticationManagerBuilder;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.csrf().disable()
.authorizeRequests().antMatchers("/login").permitAll()
.anyRequest().authenticated()
.and()
.formLogin()
.loginPage("/login").permitAll();
//remember me configuration
http
.rememberMe()
.key("myUniqueKey")
.rememberMeCookieName("websparrow-login-remember-me")
.tokenValiditySeconds(10000000);
http
.logout()
.logoutUrl("/logout");
}
@Autowired
public void configureGlobal(AuthenticationManagerBuilder auth) throws Exception {
auth
.inMemoryAuthentication()
.withUser("websparrow").password("{noop}web123").roles("USER");
}
}
In the above Java configuration class:
rememberMe() method return the RememberMeConfigurer
class for further customizations.
key(String key) method sets the key to identify tokens created for remember me authentication.
rememberMeCookieName(String rememberMeCookieName) method sets the name of cookie which stores the token for remember me authentication. Defaults to ‘remember-me’.
tokenValiditySeconds(int tokenValiditySeconds) method allows specifying how long (in seconds) a token is valid for.
3. Test the Application
To test the Remember Me feature of the application follows the below steps:
1. Run your application as Spring Boot.
2. Login with your username, password, and don’t forget to check the Remember Me checkbox.
3. After the successful login, close the browser and reopen it. Try to access your secure pages, this time it will not ask to reenter the username and password.
4. This is because it stores user’s login information into the web browser cookies.
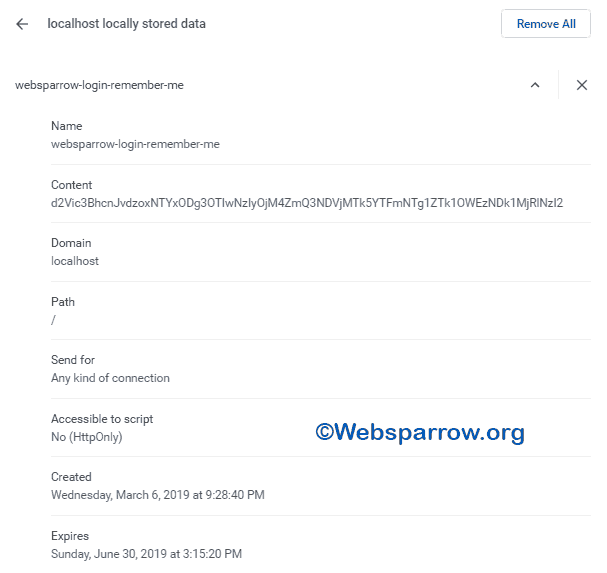
Download Source Code: spring-boot-security-remember-me-example.zip