Customizing Swagger 2 RESTful API Documentation
Swagger 2 is very flexible to customize our RESTful API documentation information like title, description, contact details, etc. Swagger 2 provides the ApiInfo
class which helps to customize the API metadata and Contact
class for contact details in springfox.documentation.service
package. To learn more about API documentation, refer our Spring Boot + Swagger 2 API Documentation example.
By default, Swagger 2 gives you the following info:
public static final Contact DEFAULT_CONTACT = new Contact("", "", "");
public static final ApiInfo DEFAULT = new ApiInfo("Api Documentation", "Api Documentation", "1.0", "urn:tos",
DEFAULT_CONTACT, "Apache 2.0", "http://www.apache.org/licenses/LICENSE-2.0");
which results looks like on your API documentation web page as follow:
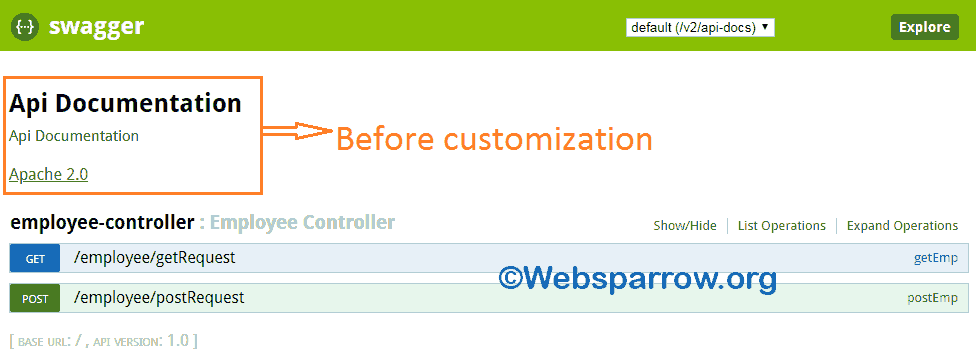
And to for customizing the information, edit the above information and add it to your Swagger2Config
file as given below:
apiInfo(ApiInfo apiInfo)
method – Sets the API’s meta information as included in the JSON ResourceListing response.
package org.websparrow.swagger.config;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import springfox.documentation.builders.RequestHandlerSelectors;
import springfox.documentation.service.ApiInfo;
import springfox.documentation.service.Contact;
import springfox.documentation.spi.DocumentationType;
import springfox.documentation.spring.web.plugins.Docket;
import springfox.documentation.swagger2.annotations.EnableSwagger2;
@Configuration
@EnableSwagger2
public class Swagger2Config {
@Bean
public Docket empApiDoc() {
return new Docket(DocumentationType.SWAGGER_2).select()
.apis(RequestHandlerSelectors.basePackage("org.websparrow.controller")).
build().apiInfo(NEW_API_INFO);
}
public static final Contact NEW_CONTACT_DETAILS = new Contact("Websparrow.org Team", "https://websparrow.org/",
"[email protected]");
public static final ApiInfo NEW_API_INFO = new ApiInfo("Employee RESTful API Documentation",
"This RESTful service produces the employee deatils in JSON formats.", "1.0",
"https://websparrow.org/terms-of-service", NEW_CONTACT_DETAILS, "Apache 2.0",
"http://www.apache.org/licenses/LICENSE-2.0");
}
The above customization results look like on Swagger UI as follow:
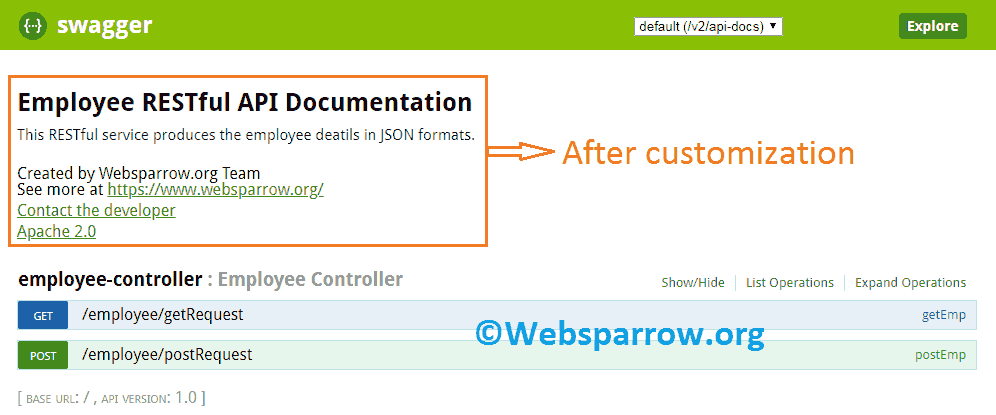
Note: You can also use the
ApiInfo
class constructor for metadata customization but it is deprecated since2.4.0
version.@Deprecated public ApiInfo( String title, String description, String version, String termsOfServiceUrl, String contactName, String license, String licenseUrl) { this(title, description, version, termsOfServiceUrl, new Contact(contactName, "", ""), license, licenseUrl); }
References
Spring Boot RESTful API Documentation with Swagger 2