How to connect Spring Boot application with MongoDB
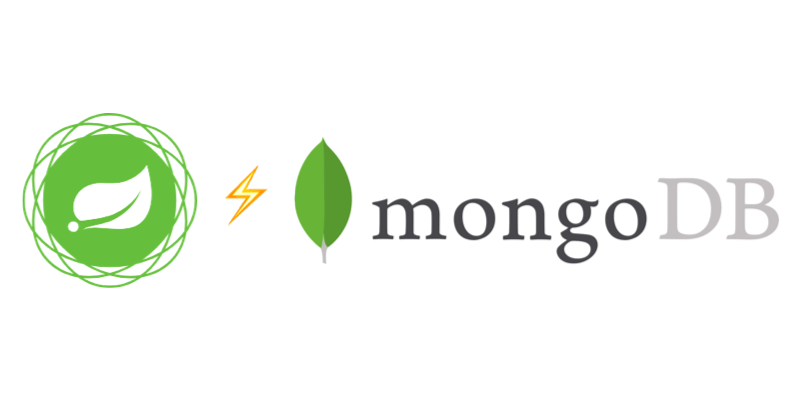
This guide will help you to establish a database connection bridge between the Spring Boot application and MongoDB database. You can connect the Spring Boot application with the MongoDB database in three ways:
- Defining connection strings in the application.properties file.
- Java-based configuration
- XML configuration
1. application.properties
As we know Spring Boot follows the “convention over configuration” model which helps developers to focus on the application’s core logic rather than configuration. You can define the MongoDB database credentials in the application.properties files as given below and the rest jobs will be done by the Spring Boot.
application.properties
#Database connection strings
spring.data.mongodb.host=localhost # host name where mongodb is running
spring.data.mongodb.port=27017 #port number, by default MongoDB uses the 27017 port
spring.data.mongodb.database=mydreamapp #name of the database
spring.data.mongodb.username=admin #user name for db
spring.data.mongodb.password=admin123 # your secret password
2. Java-based Configuration
We can use the @Configuration
annotation to established the connection bridge between the Spring Boot application and MongoDB database.
package org.websparrow.config;
import com.mongodb.*;
import org.springframework.context.annotation.Configuration;
import org.springframework.data.mongodb.config.AbstractMongoClientConfiguration;
import java.util.Collections;
@Configuration
public class MongoDBConfig extends AbstractMongoClientConfiguration {
@Override
protected String getDatabaseName() {
return "myapp";
}
@Override
protected void configureClientSettings(MongoClientSettings.Builder builder) {
builder.credential(MongoCredential.createCredential(
"username", "databasename", "password".toCharArray())
)
.applyToClusterSettings(settings -> {
settings.hosts(Collections.singletonList(new ServerAddress("hostname", 27017)));
});
}
}
Alternatively, we can also use the below configurations:
package org.websparrow.config;
import com.mongodb.*;
import org.springframework.context.annotation.Configuration;
import org.springframework.data.mongodb.config.AbstractMongoClientConfiguration;
import java.util.Collection;
import java.util.Collections;
@Configuration
public class MongoDBConfig extends AbstractMongoClientConfiguration {
@Override
protected String getDatabaseName() {
return "my_dream_app";
}
@Override
public MongoClient mongoClient() {
ConnectionString connectionString = new ConnectionString("mongodb://localhost:27017/my_dream_app");
MongoClientSettings mongoClientSettings = MongoClientSettings.builder()
.applyConnectionString(connectionString)
.build();
return MongoClients.create(mongoClientSettings);
}
@Override
public Collection<String> getMappingBasePackages() {
return Collections.singleton("org.websparrow");
}
}
3. XML Configuration
We’ll update shortly.
References
- Getting Started with Spring Boot and MongoDB
- Spring Data MongoDB – Reference Documentation
- MongoDB Website
- MongoDB Docs
Similar Posts
- Failed to start bean ‘documentationPluginsBootstrapper’; nested exception is java.lang.NullPointerException
- Spring Boot Security- Change default username and password parameter
- Spring Boot + Activiti Service Task Example
- Spring Bean Life Cycle Management Example
- Spring Boot REST API File Upload/Save Example