Generate QR Code using Spring Boot REST API
This tutorial will help you to expose REST API to generate the QR Code using the Spring Boot framework. QRGen library provides an API to generate the QR Code that explained in one of our previous tutorials “How to generate QR Code in Java“.
ResponseEntity<T>
of the package org.springframework.http
will help to produce QR Code image on the web browser by setting the content type MediaType.IMAGE_JPEG
or MediaType.IMAGE_PNG
.
You can visit Spring Boot- Display image from database and classpath to learn more about how to display using the REST API.
Technology Used
Find the list of tools/technologies/software/libraries used in this application.
- JDK 1.8
- Spring Boot 2.3.4.RELEASE
- QRGen library
- Maven 3.2+
- Your favorite IDE:
- Spring Tool Suite (STS)
- Eclipse
- IntelliJ IDEA
Dependencies Required
Here is the pom.xml file including the required dependencies used in this project.
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.3.4.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>org.websparrow</groupId>
<artifactId>spring-boot-qr-code</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>spring-boot-qr-code</name>
<properties>
<java.version>1.8</java.version>
</properties>
<repositories>
<repository>
<id>jitpack.io</id>
<url>https://jitpack.io</url>
</repository>
</repositories>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>com.github.kenglxn.qrgen</groupId>
<artifactId>javase</artifactId>
<version>2.6.0</version>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
Service
Create a QRCodeService
interface and declare generate
method which accepts 3 parameters i.e. text, width, height, and its return type is byte []
.
text
is a String type that displays when you scan the QR code.width
is an Integer type that defines the width of the QR code.- and
height
is also an Integer type that defines the height of the QR code.
package org.websparrow.service;
public interface QRCodeService {
byte[] generate(String text, int width, int height);
}
QRCodeServiceImpl
is the implementation of the QRCodeService
interface which calls the static method from(text)
and withSize(width, height)
of the QRCode
class provided by QRGen library.
package org.websparrow.service;
import net.glxn.qrgen.javase.QRCode;
import org.springframework.stereotype.Service;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
@Service
public class QRCodeServiceImpl implements QRCodeService {
@Override
public byte[] generate(String text, int width, int height) {
try (ByteArrayOutputStream bos = QRCode.from(text).withSize(width, height).stream(); ) {
return bos.toByteArray();
} catch (IOException e) {
e.printStackTrace();
return null;
}
}
}
Controller
QRCodeController
exposes the REST end-point for the end-user to display the QR code on the web browser. To keep it simple, we define the hardcoded value of QR text, width, and height.
package org.websparrow.controller;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.MediaType;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
import org.websparrow.service.QRCodeService;
@RestController
public class QRCodeController {
private final int WIDTH = 250;
private final int HEIGHT = 250;
private final String QR_TEXT = "Spring Boot REST API to generate QR Code - Websparrow.org";
@Autowired private QRCodeService qrCodeService;
@GetMapping("qr-code")
public ResponseEntity<byte[]> getQrCode() {
byte[] qrImage = qrCodeService.generate(QR_TEXT, WIDTH, HEIGHT);
return ResponseEntity.ok().contentType(MediaType.IMAGE_PNG).body(qrImage);
}
}
Run the application
This class is used to start the Spring Boot application.
package org.websparrow;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class SpringBootQRCodeApp {
public static void main(String[] args) {
SpringApplication.run(SpringBootQRCodeApp.class, args);
}
}
Test the application
When the application starts successfully, hit the below API in your favorite web browser.
API- http://localhost:8080/qr-code
You will find your QR code as given below:
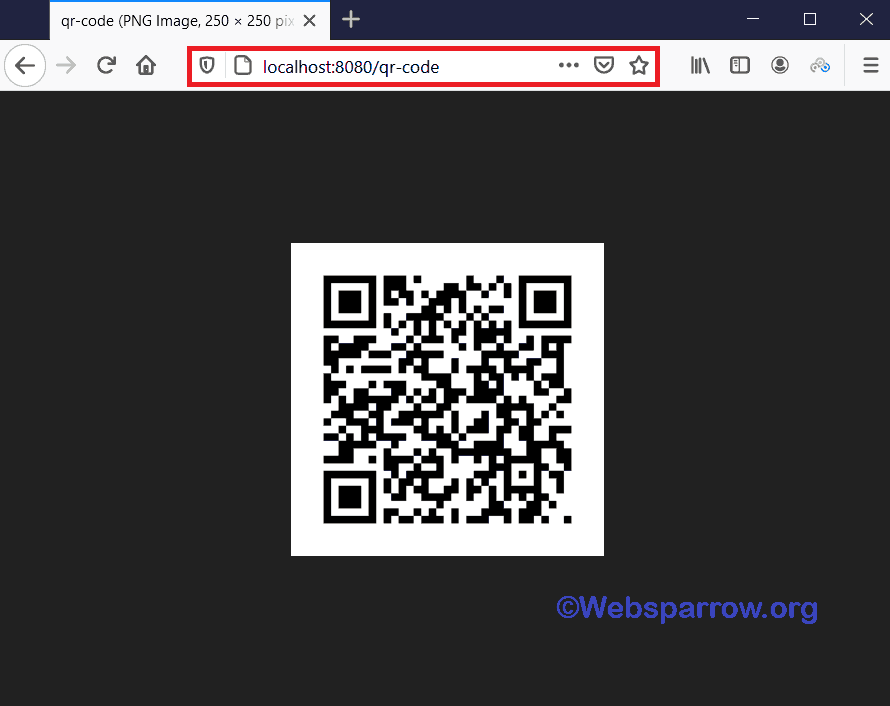
References
- How to generate QR Code in Java
- Spring Boot- Display image from database and classpath
- QRGen- Github
- QRGen- JitPack