How to generate QR Code in Java
QRGen library provides an API to generate the QR Code in a Java application. It is built on the top of ZXing. Add the following dependency to your project’s pom.xml file.
<repositories>
<repository>
<id>jitpack.io</id>
<url>https://jitpack.io</url>
</repository>
</repositories>
<dependencies>
<dependency>
<groupId>com.github.kenglxn.qrgen</groupId>
<artifactId>javase</artifactId>
<version>2.6.0</version>
</dependency>
</dependencies>
P.S. The QRGen library is no longer deployed to Maven Central, but it’s available on jitpack.io.
The sample of QR Code generated through QRGen library:
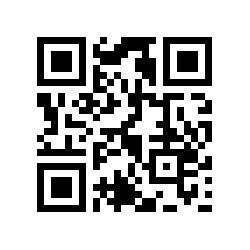
QRCodeDemo.java
package org.websparrow;
import net.glxn.qrgen.javase.QRCode;
import javax.imageio.ImageIO;
import java.awt.image.BufferedImage;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.io.IOException;
public class QRCodeDemo {
private static final String BARCODE_TEXT = "https://websparrow.org";
public static void main(String[] args) {
try (ByteArrayOutputStream bos = QRCode.from(BARCODE_TEXT).withSize(250, 250).stream(); ) {
ByteArrayInputStream bis = new ByteArrayInputStream(bos.toByteArray());
ImageIO.write(ImageIO.read(bis), "PNG", new File("QRCode.png"));
System.out.println("QR Code successfully generated");
} catch (IOException e) {
e.printStackTrace();
}
}
}