Struts 2 and Hibernate Integration Example- XML based
In this Struts 2 tutorial, we will show you how to integrate the Struts 2 application with Hibernate and save the data into the database.
Check the key points.
- Register the ServletContextListener.
- Initialize the Hibernate session and store it into the servlet context.
- In action class, get the Hibernate session from the servlet context.
Software Used
- Eclipse IDE
- Tomcat 8
- JDK 8
- MySQL Database
Project Structure in Eclipse
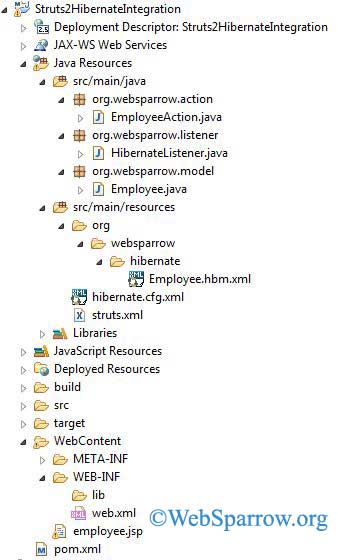
Required Dependencies
You can directly add all core JARs of struts2.x.x and hibernate3.5.x in your application or add below to your pom.xml
if your application maven based.
<dependencies>
<!--struts 2-->
<dependency>
<groupId>org.apache.struts</groupId>
<artifactId>struts2-core</artifactId>
<version>2.3.16</version>
</dependency>
<!--hibernate-->
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-annotations</artifactId>
<version>3.5.6-Final</version>
</dependency>
<dependency>
<groupId>org.hibernate.javax.persistence</groupId>
<artifactId>hibernate-jpa-2.0-api</artifactId>
<version>1.0.1.Final</version>
</dependency>
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-validator</artifactId>
<version>4.1.0.Final</version>
</dependency>
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-api</artifactId>
<version>1.7.7</version>
</dependency>
<dependency>
<groupId>log4j</groupId>
<artifactId>log4j</artifactId>
<version>1.2.16</version>
</dependency>
<!--mysql connector-->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>5.1.17</version>
</dependency>
</dependencies>
Table Script
Create an employee table in your database to store the information.
CREATE TABLE `employee` (
`emp_id` int(5) NOT NULL AUTO_INCREMENT,
`emp_name` varchar(50) DEFAULT NULL,
`emp_email` varchar(60) DEFAULT NULL,
`emp_dept` varchar(20) DEFAULT NULL,
`emp_salary` int(10) DEFAULT '0',
PRIMARY KEY (`emp_id`)
);
Hibernate Utils
Create the Hibernate configuration file.
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE hibernate-configuration PUBLIC
"-//Hibernate/Hibernate Configuration DTD 3.0//EN"
"http://www.hibernate.org/dtd/hibernate-configuration-3.0.dtd">
<hibernate-configuration>
<session-factory>
<property name="hibernate.connection.driver_class">org.gjt.mm.mysql.Driver</property>
<property name="hibernate.connection.url">jdbc:mysql://127.0.0.1:3306/websparrow</property>
<property name="hibernate.connection.username">root</property>
<property name="hibernate.dialect">org.hibernate.dialect.MySQLDialect</property>
<property name="show_sql">true</property>
<property name="format_sql">true</property>
<mapping resource="org/websparrow/hibernate/Employee.hbm.xml" />
</session-factory>
</hibernate-configuration>
Now create a model class for employee parameters and generates its getters and setters….
package org.websparrow.model;
import java.io.Serializable;
public class Employee implements Serializable {
private static final long serialVersionUID = 4513107453540343598L;
// getters and setters...
private Long empId;
private String empName;
private String empDept;
private String empSalary;
private String empEmail;
public Employee() {
// TODO Auto-generated constructor stub
}
public Employee(String empName, String empDept, String empSalary, String empEmail) {
this.empDept = empDept;
this.empEmail = empEmail;
this.empName = empName;
this.empSalary = empSalary;
}
}
Map the all employee columns in hibernate mapping xml file.
<?xml version="1.0"?>
<!DOCTYPE hibernate-mapping PUBLIC "-//Hibernate/Hibernate Mapping DTD 3.0//EN"
"http://hibernate.sourceforge.net/hibernate-mapping-3.0.dtd">
<hibernate-mapping>
<class name="org.websparrow.model.Employee" table="employee" catalog="websparrow">
<id name="empId" type="java.lang.Long">
<column name="emp_id" />
<generator class="identity" />
</id>
<property name="empName" type="string">
<column name="emp_name" />
</property>
<property name="empEmail" type="string">
<column name="emp_email" />
</property>
<property name="empDept" type="string">
<column name="emp_dept" />
</property>
<property name="empSalary" type="string">
<column name="emp_salary" />
</property>
</class>
</hibernate-mapping>
Register the ServletContextListener and , initialize the Hibernate session and store it into the servlet context.
package org.websparrow.listener;
import java.net.URL;
import javax.servlet.ServletContextEvent;
import javax.servlet.ServletContextListener;
import org.hibernate.SessionFactory;
import org.hibernate.cfg.Configuration;
public class HibernateListener implements ServletContextListener {
private Configuration config;
private SessionFactory factory;
private static Class<HibernateListener> cls = HibernateListener.class;
public static final String KEY_NANE = cls.getName();
@Override
public void contextDestroyed(ServletContextEvent arg) {
// TODO Auto-generated method stub
}
@Override
public void contextInitialized(ServletContextEvent arg) {
try {
URL url = HibernateListener.class.getResource("/hibernate.cfg.xml");
config = new Configuration().configure(url);
factory = config.buildSessionFactory();
arg.getServletContext().setAttribute(KEY_NANE, factory);
} catch (Exception e) {
e.printStackTrace();
}
}
}
Add Struts 2 Filter and Register Hibernate Listener
Define the struts 2 filter and register the listener in web.xml.
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd"
id="WebApp_ID" version="2.5">
<display-name>Struts2HibernateIntegration</display-name>
<welcome-file-list>
<welcome-file>employee.jsp</welcome-file>
</welcome-file-list>
<filter>
<filter-name>struts2</filter-name>
<filter-class>org.apache.struts2.dispatcher.ng.filter.StrutsPrepareAndExecuteFilter</filter-class>
</filter>
<filter-mapping>
<filter-name>struts2</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
<listener>
<listener-class>org.websparrow.listener.HibernateListener</listener-class>
</listener>
</web-app>
Action Class
Create an action class for employee and get the Hibernate session from the servlet context.
package org.websparrow.action;
import java.util.ArrayList;
import java.util.List;
import org.apache.struts2.ServletActionContext;
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.websparrow.listener.HibernateListener;
import org.websparrow.model.Employee;
import com.opensymphony.xwork2.ActionSupport;
import com.opensymphony.xwork2.ModelDriven;
public class EmployeeAction extends ActionSupport implements ModelDriven<Object> {
private static final long serialVersionUID = -7234472936649108818L;
Employee employee = new Employee();
List<Employee> empList = new ArrayList<>();
@Override
public String execute() throws Exception {
return SUCCESS;
}
public Object getModel() {
return employee;
}
// add employee in database
@SuppressWarnings("unchecked")
public String addEmployee() throws Exception {
SessionFactory sessionFactory = (SessionFactory) ServletActionContext.getServletContext()
.getAttribute(HibernateListener.KEY_NANE);
Session session = sessionFactory.openSession();
session.beginTransaction();
session.save(employee);
session.getTransaction().commit();
empList = null;
empList = session.createQuery("from Employee").list();
return SUCCESS;
}
// fetch employees from database
@SuppressWarnings("unchecked")
public String fetchEmployee() throws Exception {
SessionFactory sessionFactory = (SessionFactory) ServletActionContext.getServletContext()
.getAttribute(HibernateListener.KEY_NANE);
Session session = sessionFactory.openSession();
empList = session.createQuery("from Employee").list();
return SUCCESS;
}
public List<Employee> getEmpList() {
return empList;
}
public void setEmpList(List<Employee> empList) {
this.empList = empList;
}
}
JSP Pages
Create a jsp page to get and display the employee information.
<%@taglib uri="/struts-tags" prefix="s"%>
<!DOCTYPE>
<html>
<head>
<title>Struts 2 and Hibernate Integration Example</title>
</head>
<body>
<!-- registering employees -->
<h1>Struts 2 and Hibernate Integration Example</h1>
<s:form action="registerEmployee" method="POST">
<s:textfield label="Emp Name" name="empName"></s:textfield>
<s:textfield label="Emp Email" name="empEmail"></s:textfield>
<s:textfield label="Emp Dept" name="empDept"></s:textfield>
<s:textfield label="Emp Salary" name="empSalary"></s:textfield>
<s:submit value="Submit"></s:submit>
</s:form>
<!-- listing employees -->
<s:if test="empList.size() > 0">
<h2>List of All Employees</h2>
<table border="1px" cellpadding="8px">
<tr>
<th>Emp Id</th>
<th>Name</th>
<th>Email</th>
<th>Department</th>
<th>Salary</th>
</tr>
<s:iterator value="empList">
<tr>
<td><s:property value="empId" /></td>
<td><s:property value="empName" /></td>
<td><s:property value="empEmail" /></td>
<td><s:property value="empDept" /></td>
<td><s:property value="empSalary" /></td>
</tr>
</s:iterator>
</table>
</s:if>
</body>
</html>
Map Action Class in Struts.xml
List all the action classes in struts.xml and corresponding jsp.
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE struts PUBLIC
"-//Apache Software Foundation//DTD Struts Configuration 2.0//EN"
"http://struts.apache.org/dtds/struts-2.0.dtd">
<struts>
<constant name="struts.devMode" value="true" />
<package name="default" namespace="/" extends="struts-default">
<action name="registerEmployee" class="org.websparrow.action.EmployeeAction" method="addEmployee">
<result name="success">/employee.jsp</result>
</action>
<action name="listEmployee" class="org.websparrow.action.EmployeeAction" method="fetchEmployee">
<result name="success">/employee.jsp</result>
</action>
</package>
</struts>
Output
Now everything’s completed. Run your application and see the results.
To display list of employee – localhost:8083/Struts2HibernateIntegration/listEmployee and register new employee – localhost:8083/Struts2HibernateIntegration/registerEmployee
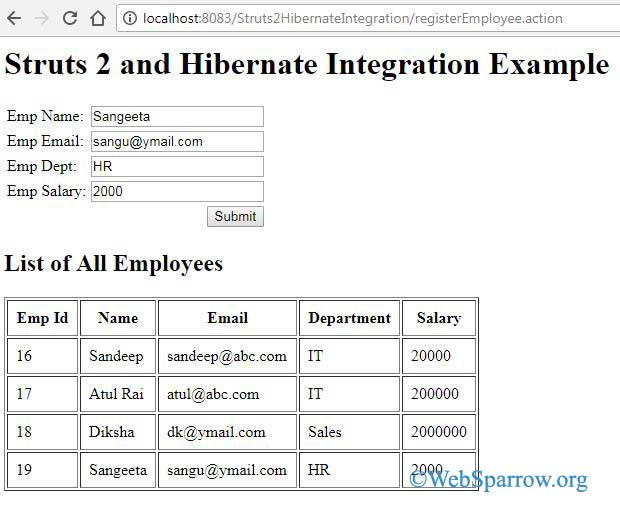
Console Log:
Hibernate: insert into websparrow.employee (emp_name, emp_email, emp_dept, emp_salary) values (?, ?, ?, ?)
Hibernate: select employee0_.emp_id as emp1_0_, employee0_.emp_name as emp2_0_, employee0_.emp_email as emp3_0_, employee0_.emp_dept as emp4_0_, employee0_.emp_salary as emp5_0_ from websparrow.employee employee0_
Download Source Code – struts-2-and-hibernate-integration-example-xml-based.zip