Struts 2 Set Tag Example
In this tutorial, we are going to tell how to use Struts 2 set tag (<s:set />
). The set tag assigns a value to a variable in a specified scope.
The scope is the following…
- application – the value will be set in application scope.
- session– the value will be set in session scope.
- request – the value will be set in request scope.
- page – the value will be set in page scope.
- action – the value will be set in the request scope and Struts action context using the name as a key.
Note: The value of set tag can be property value or hard-coded.
Assigning the property value in set tag variable.
<s:set var="ws" value="country" />
Assigning the hard-coded value in set tag variable.
<s:set var="ws" value="%{'INDIA'}" />
In this example, we check the condition using a set tag variable, if the condition is true
it will be going in IF block otherwise ELSE block.
Web.xml
Add Struts 2 filters in web.xml
<filter>
<filter-name>struts2</filter-name>
<filter-class>org.apache.struts2.dispatcher.ng.filter.StrutsPrepareAndExecuteFilter</filter-class>
</filter>
<filter-mapping>
<filter-name>struts2</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
Action Class
The action class has a country
property.
package org.websparrow;
import com.opensymphony.xwork2.ActionSupport;
public class SetTagAction extends ActionSupport {
private static final long serialVersionUID = 6971245991310816510L;
String country = "";
@Override
public String execute() throws Exception {
return "SUCCESS";
}
public String getCountry() {
return country;
}
public void setCountry(String country) {
this.country = country;
}
}
JSP Page
On the JSP page, we assign the property value into the set tag variable and check the condition.
<%@taglib prefix="s" uri="/struts-tags"%>
<html>
<head>
<title>Struts 2 Set Tag Example</title>
</head>
<body>
<h1>Struts 2 Set Tag Example</h1>
<%-- <s:set var="ws" value="%{'INDIA'}" /> --%>
<s:set var="ws" value="country" />
<s:if test="%{#ws=='INDIA'}">
<p>Hi, You are from INDIA.</p>
</s:if>
<s:else>
<p>Sorry, You are not from INDIA.</p>
</s:else>
</body>
</html>
Struts.xml
Mapping the action URL and class.
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE struts PUBLIC
"-//Apache Software Foundation//DTD Struts Configuration 2.0//EN"
"http://struts.apache.org/dtds/struts-2.0.dtd">
<struts>
<package name="default" extends="struts-default">
<action name="setTag" class="org.websparrow.SetTagAction">
<result name="SUCCESS">/index.jsp</result>
</action>
</package>
</struts>
Output
Now all is set, to check whether is it working or not, deploy the application and use the below URL. If country==INDIA it will be going in IF block otherwise ELSE block.
URL- localhost:8083/Struts2SetTagExample/setTag?country=INDIA
Screen 1
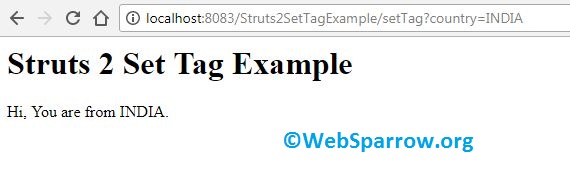
Screen 2
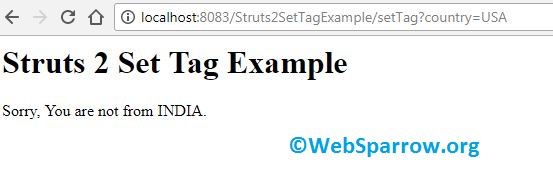
Download Source Code – struts2-set-tag-example.zip