Data Binding in Angular
This guide walks you through the Data Binding in Angular. Data binding is one of the most important concepts of Angular which lets the component interact with the view, so the application becomes more interactive and users don’t have to do write any code to achieve the same.
Angular manages applications via using components and HTML views. Component interacts with a view via API properties and methods.
Data binding in the Angular framework can be achieved in 4 ways:
- Interpolation
- Property Binding
- Event Binding
- Two-way Data Binding
1. Interpolation
Interpolation displays component’s field value into view. It is a one-way binding in which syntax ( {{name}}
) is replaced with the value of the component. Interpolation is used “only for string” expressions.
1. 1 Syntax: double curly braces {{ }}
with field value name e.g. {{name}}
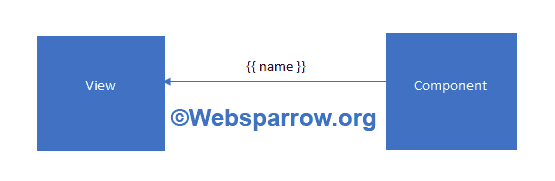
1.2 Usage: declare a variable in the component and use the String Interpolation and the value of the name variable will be displayed on the HTML view:
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
name = 'lucifer';
}
<p>Hey! My name is {{name}} </p>
2. Property Binding
Property binding sets the property of the child component from the parent component. Property binding allows variable value to change only at the component level.
2.1 Syntax: variable name followed by property name in square brackets e.g. [property]="name"
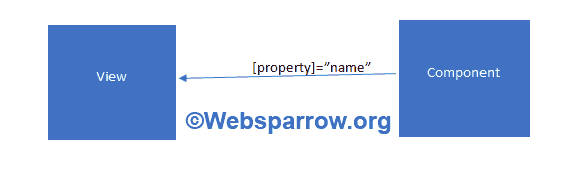
2.2 Usage:
import { Component } from '@angular/core';
@Component({
selector: 'parent-component',
templateUrl: './parent.component.html',
styleUrls: ['./parent.component.css']
})
export class ParentComponent {
private obj: any = { 'nand', 'lucifer' }
}
<child-component [data]="obj"></child-component>
3. Event Binding
Event binding is used to send data from view to component. It binds function defined in component with events.
3.1 Syntax: ()
parenthesis e.g. (eventName) = "callEvent($event)"
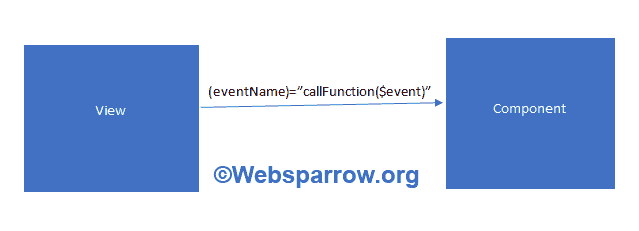
3.2 Usage:
import { Component } from '@angular/core';
@Component({
selector: 'nand-root',
templateUrl: './nand.component.html',
styleUrls: ['./nand.component.css']
})
export class NandComponent {
public callMe(event): void {
alert('it called me.');
}
}
<button (click)="callMe($event)"></button>
4. Two-way Data Binding
Two-way data binding is the combination of both Property and Event binding. It continuously synchronizes data from component to view and from view to the component. If model data in the component is changed then it will reflect on view and if any changes made on view it will update the model in the component.
4.1 Syntax: [()]
parenthesis inside square brackets e.g. [(ngModel)]="obj"
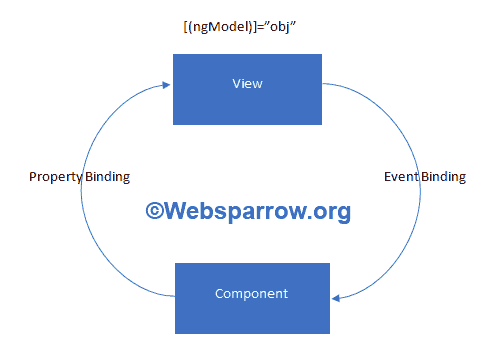
4.2 Usage:
<input [(ngModel)] = "name"></input>
<h3>My name is {{name}} </h3>
import { Component } from '@angular/core';
@Component({
selector: 'nand-root',
templateUrl: './nand.component.html',
styleUrls: ['./nand.component.css']
})
export class NandComponent {
name: string = 'lucifer'
}
NgModel
is a part of the FormsModule
, so you have to import it to your app.module.ts.