How to add JavaScript file in Angular project
In this tutorial, we are going to show how to add an external JavaScript (.js) file in the Angular project. Sometimes it’s required to add an external file to perform some task and in Angular project, an external file can not be added directly.
For example, you wanna show some alert messages when the page gets loaded through your JavaScript file. In Angular, it can be added in two ways:
Let’s see you have created a nand.js file which conation a function name()
.
function name(){
alert("I am Lucifer");
}
Solution 1
Step 1: Create a js named directory inside the assets directory and put the JavaScript (nand.js) file inside it.
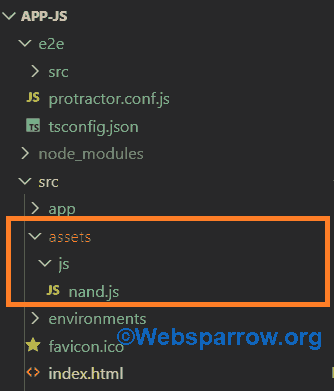
Step 2: Open the index.html and add a script tag to add the JavaScript file.
<script src="assets/js/nand.js"></script>
Step 3: Open the component where you want to use this JS file.
Add below line to declare a variable:
declare var name: any;
Use below line to call the function:
new name();
import { Component, OnInit } from '@angular/core';
declare var name: any;
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit{
ngOnInit(): void {
new name();
}
title = 'app-js';
}
Solution 2
Step 1: Repeat Steps 1 & 2 already discussed in the above solution.
Step 2: Open the angular.json and add the JS file path in the “scripts” array.
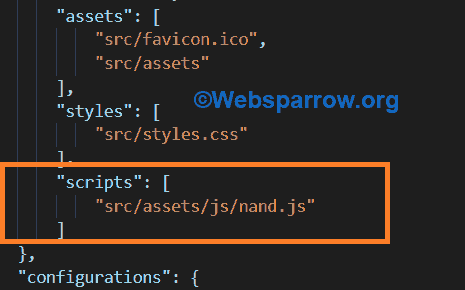
Step 3: Open the component where you want to use this JS file.
Add below line to declare a variable:
declare var name: any;
Use below line to call the function:
new name();
import { Component, OnInit } from '@angular/core';
declare var name: any;
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit{
ngOnInit(): void {
new name();
}
title = 'app-js';
}
Test the application
“Voila”, now run your application and test. As soon as the application load it will open an alert box saying “I am Lucifer”.