Struts 2 pagination using display tag
In this Struts 2 tutorial, we will talk about pagination using display tag library. Showing a huge amount of records on a single page is not a good idea. You can divide it into several pages. In Struts 2, you can do that using display tag library. This library is reached in features like…
- Paging
- Sorting
- Grouping
- Exporting, etc.
Similar tutorial- Pagination in Struts 2 using jQuery datatable
Dependencies Required
To resolve the JARs dependency, you can add the following in your pom.xml file.
<dependencies>
<dependency>
<groupId>org.apache.struts</groupId>
<artifactId>struts2-core</artifactId>
<version>2.5</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>5.1.17</version>
</dependency>
<dependency>
<groupId>displaytag</groupId>
<artifactId>displaytag</artifactId>
<version>1.2</version>
</dependency>
</dependencies>
Or you can directly download it by clicking here.
Tag Description
Here is some tag and their properties that we are going to use in this example.
First of all, to work with display tag, the following tag extension should be placed in each JSP page that uses the display taglib.
<%@taglib prefix="display" uri="http://displaytag.sf.net"%>
<display:table> tag: Displays a list in an HTML table, formatting each item in the list according to the Column tags nested inside of this tag.
Attribute Name | Description |
---|---|
name | Name of the list that contains records. |
pagesize | Number of records to be displayed on a single page. |
requestedURI | URL that you are requested for pagination. |
length | Number of records to be shown out of the total. |
export | Enable/disable export. Valid values are true or false. |
sort | Use ‘page’ if you want to sort only visible records, or ‘list’ if you want to sort the full list, or ‘external’ if the data is sorted outside displaytag. |
<display:column> tag: Displays a property of a row object inside a table. MUST be nested inside of a Table tag.
Attribute Name | Description |
---|---|
property | Name of the property in the bean specified in the parent table tag (via the “name” attribute) mapped to this column. |
title | Title of the column (text for the th cell). |
sortable | Set to ‘true’ to make the column sortable. Defaults to ‘false’. |
value | Static value to be used for the column. |
<display:caption> tag: Simple tag which mimics the html caption tag. Use it inside a table tag to display a caption.
<display:caption>City list of State Andhra Pradesh</display:caption>
<display:footer> tag:
Tag which should be nested into a table tag to provide a custom table footer. The body of the tag is into the tfoot section of the table.
<display:footer>
<tr>
<td>Thank You</td>
<td>For</td>
<td>Learning</td>
<tr>
</display:footer>
<display:setProperty> tag:
Sets the indicated property on the enclosing Table tag. MUST be nested within a Table tag.
<display:setProperty name="paging.banner.placement" value="bottom" />
Struts 2 Filter
Define the Struts 2 filter in web.xml file.
<filter>
<filter-name>struts2</filter-name>
<filter-class>org.apache.struts2.dispatcher.filter.StrutsPrepareAndExecuteFilter</filter-class>
</filter>
<filter-mapping>
<filter-name>struts2</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
DAO Class
Create the Data Access Object class to fetch the city list details from the table.
package org.websparrow.dao;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
public class DisplayDao {
public static ResultSet report() throws SQLException {
ResultSet rs = null;
try {
Class.forName("com.mysql.jdbc.Driver");
Connection con = DriverManager.getConnection("jdbc:mysql://127.0.0.1:3306/websparrow", "root", "");
PreparedStatement ps = con.prepareStatement("SELECT * FROM cities where city_state='Andhra Pradesh'");
rs = ps.executeQuery();
} catch (Exception e) {
e.printStackTrace();
}
return rs;
}
}
Action Class
Inside the Action class add all records into an ArrayList
.
package org.websparrow.action;
import java.sql.ResultSet;
import java.util.ArrayList;
import java.util.List;
import com.opensymphony.xwork2.ActionSupport;
public class DisplayAction extends ActionSupport {
private static final long serialVersionUID = -7591893545033222898L;
private DisplayAction dto = null;
private int cityId;
private String cityName;
private String cityState;
private List<DisplayAction> cityList = null;
@Override
public String execute() throws Exception {
ResultSet rs = org.websparrow.dao.DisplayDao.report();
cityList = new ArrayList<DisplayAction>();
if (rs != null) {
while (rs.next()) {
dto = new DisplayAction();
dto.setCityId(rs.getInt(1));
dto.setCityName(rs.getString(2));
dto.setCityState(rs.getString(3));
cityList.add(dto);
}
}
return SUCCESS;
}
public List<DisplayAction> getCityList() {
return cityList;
}
public void setCityList(List<DisplayAction> cityList) {
this.cityList = cityList;
}
public int getCityId() {
return cityId;
}
public void setCityId(int cityId) {
this.cityId = cityId;
}
public String getCityName() {
return cityName;
}
public void setCityName(String cityName) {
this.cityName = cityName;
}
public String getCityState() {
return cityState;
}
public void setCityState(String cityState) {
this.cityState = cityState;
}
}
Configuration
Finally, map the action class inside the struts.xml.
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE struts PUBLIC
"-//Apache Software Foundation//DTD Struts Configuration 2.5//EN"
"http://struts.apache.org/dtds/struts-2.5.dtd">
<struts>
<constant name="struts.devMode" value="true" />
<package name="default" extends="struts-default" namespace="/">
<action name="pagination" class="org.websparrow.action.DisplayAction">
<result name="success">/index.jsp</result>
</action>
</package>
</struts>
UI Component
Create the JSP page for the user interface.
<%@taglib prefix="s" uri="/struts-tags"%>
<%@taglib prefix="display" uri="http://displaytag.sf.net"%>
<html>
<head>
<style type="text/css">
table, th, td {
border: 1px solid black;
}
table {
width: 38%;
}
</style>
</head>
<body>
<h2>Struts 2 pagination using display tag</h2>
<display:table name="cityList" pagesize="12" requestURI="pagination">
<display:caption>City list of State Andhra Pradesh</display:caption>
<display:column property="cityId" title="City ID" />
<display:column property="cityName" title="City Name" />
<display:column property="cityState" title="State" />
<display:setProperty name="paging.banner.placement" value="bottom" />
</display:table>
</body>
</html>
Now everything is all set. Deploy the project inside the Tomcat » webapps folder, start the server and hit the following URL localhost:8087/Struts2DisplayTag/pagination. You will get the output as shown in the image.
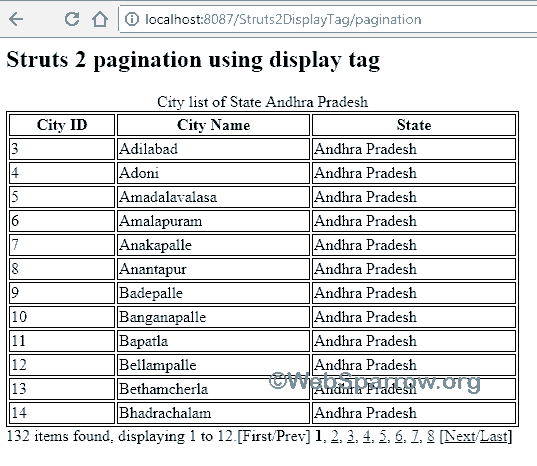
Sorting
If you want to sort the table records, add the sortable="true"
attribute to <display:column>
tag.
This will sort the record for current page only but if you want to apply sorting on whole records, add sort="list"
attribute on <display:table>
tag.
Exporting
To enable the exporting, add the export="true"
attribute on <display:table>
tag.
Download Source Code- struts2-pagination-using-display-tag.zip