How to send data from JSP to Struts action class using jQuery Ajax
This tutorial will explain the detail of How to send the Form value from JSP to Action class using Ajax. In the below example, we are going to use jQuery Ajax
to send the requests and getting the responses on the same page.
Code Snippet
function sendMyValue() {
var name = $("#name").val();
$.ajax({
type : "GET",
url : "sendvaluetoaction",
data : "name=" + name,
success : function(data) {
var html = "
" + data.msg;
$("#info").html(html);
},
error : function(data) {
alert("Some error occured.");
}
});
}
How to Send Data
You can send the JSP data in three ways to action class.
Way 1:
data : "name=" + name
Way 2:
data : {name : name}
Way 3:
url : "sendvaluetoaction?name="+name
Required JAR
- all struts 2 core jars
- struts2-json-plugin-2.x.x.jar
- commons-lang3-3.2.jar
struts2-json-plugin-2.x.x.jar file allows you to serialize the Action class attribute which has getter and setter into a JSON object
.
Project Structure in Eclipse
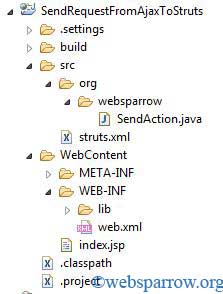
Add Struts Filter
Define the struts 2 filters into web.xml
web.xml
<filter>
<filter-name>struts2</filter-name>
<filter-class>org.apache.struts2.dispatcher.ng.filter.StrutsPrepareAndExecuteFilter</filter-class>
</filter>
<filter-mapping>
<filter-name>struts2</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
Create Action Class
SendAction.java
package org.websparrow;
import com.opensymphony.xwork2.ActionSupport;
public class SendAction extends ActionSupport {
private String name;
private String msg;
@Override
public String execute() throws Exception {
try {
setMsg("Hello " + getName());
System.out.println(msg);
} catch (Exception e) {
e.printStackTrace();
}
return "SUCCESS";
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getMsg() {
return msg;
}
public void setMsg(String msg) {
this.msg = msg;
}
}
Map the Action Class
Now map your action class in struts.xml. We will need to set package extends="json-default"
result type is json
because data comes in json format.
struts.xml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE struts PUBLIC
"-//Apache Software Foundation//DTD Struts Configuration 2.0//EN"
"http://struts.apache.org/dtds/struts-2.0.dtd">
<struts>
<constant name="struts.devMode" value="true" />
<package name="default" extends="json-default" namespace="/">
<action name="sendvaluetoaction" class="org.websparrow.SendAction">
<result name="SUCCESS" type="json">/index.jsp</result>
</action>
</package>
</struts>
Design UI
Finally we need to design JSP page that send the value to Action Class and on the same page we will display the response handled by jQuery.
index.jsp
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.1.1/jquery.min.js"></script>
<title>Send Value to Struts Action Class using Ajax</title>
<script>
function sendMyValue() {
var name = $("#name").val();
$.ajax({
type : "GET",
url : "sendvaluetoaction",
data : "name=" + name,
success : function(data) {
var html = "<br>" + data.msg;
$("#info").html(html);
},
error : function(data) {
alert("Some error occured.");
}
});
}
</script>
</head>
<body>
<h1>Send Value to Struts Action Class using Ajax</h1>
<input type="text" id="name" placeholder="Enter your name">
<button type="submit" onclick="sendMyValue()" style="background-color: #008CBA;">Submit</button>
<div id="info" style="color: green; font-size: 35px;"></div>
</body>
</html>
Run Application
To run the application start your server and hit this URL in address bar localhost:8080/SendRequestFromAjaxToStruts/ and respected output is gievn below
Screen 1
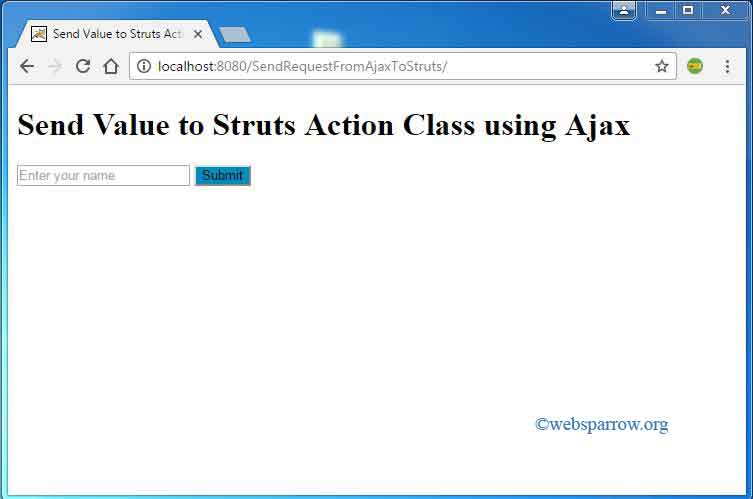
Screen 2
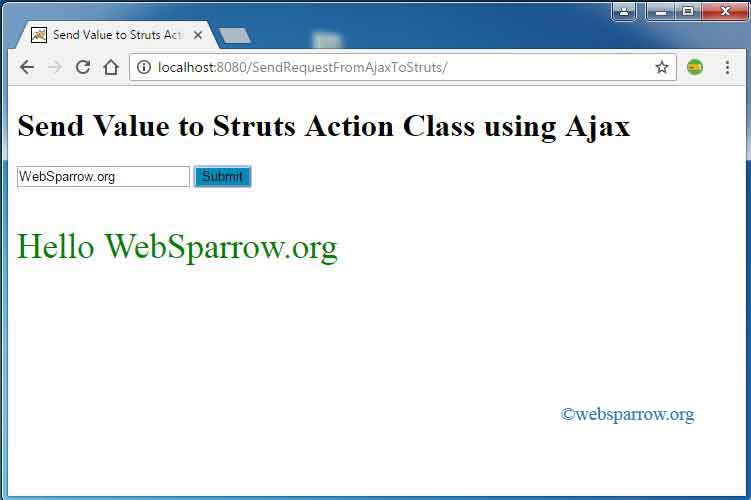
Download Source Code – how-to-send-data-from-jsp-to-struts-action-class-using-jquery-ajax.zip