Login and Logout using Session in Struts 2
This Struts 2 tutorial will explain how to manage the Session
in Struts 2 and develop a login and logout module on the basis of Session
.
In this example, we are going to use SessionAware
interface that must be implemented by the Action class. SessionAware
interface has only one method setSession
.
void setSession(Map<String,Object> session) – ->Sets the Map of session attributes in the implementing class.
What is Session and How it will work?
A session is piece information that stored in web browser cache and destroyed when the web browser is closed.
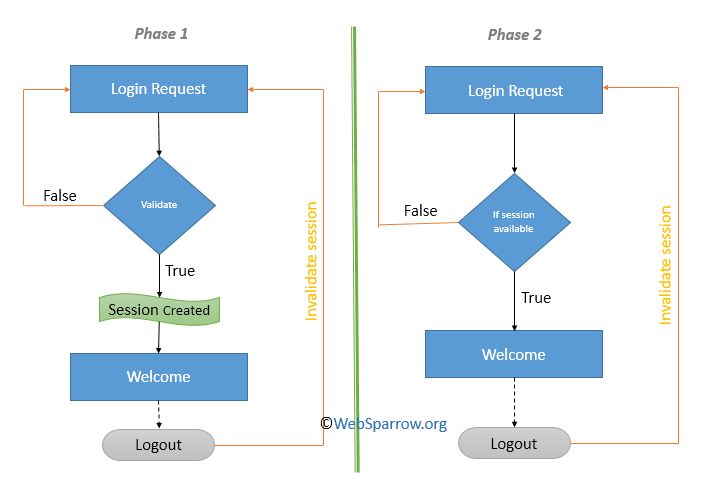
SessionMap class
A simple implementation of the Map interface to handle a collection of HTTP session attributes. The entrySet() method enumerates over all session attributes and creates a Set of entries.
public class SessionMap<K,V> extends AbstractMap<K,V> implements Serializable
Methods of SessionMap Class
- public void clear() –> Removes all attributes from the session as well as clears entries in this map.
- public boolean containsKey(Object key) –>
Checks if the specified session attribute with the given key exists. - public Set<Map.Entry<K,V>> entrySet() –>
Returns a Set of attributes from the HTTP session. - public V get(Object key) –>
Returns the session attribute associated with the given key or null if it doesn’t exist. - public void invalidate() –>
Invalidate the HTTP session. - public V put(K key, V value) –>
Saves an attribute in the session. - public V remove(Object key) –>
Removes the specified session attribute.
Software Used
- Eclipse IDE
- JDK 8
- Tomcat 8
Project Structure in Eclipse
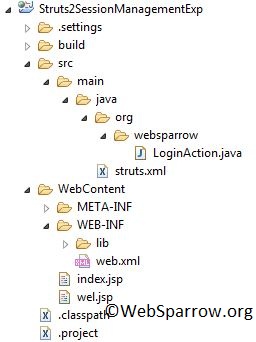
Add Struts 2 Filter in web.xml
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_3_1.xsd" id="WebApp_ID" version="3.1">
<display-name>Struts2SessionManagementExp</display-name>
<welcome-file-list>
<welcome-file>index.jsp</welcome-file>
</welcome-file-list>
<filter>
<filter-name>struts2</filter-name>
<filter-class>org.apache.struts2.dispatcher.ng.filter.StrutsPrepareAndExecuteFilter</filter-class>
</filter>
<filter-mapping>
<filter-name>struts2</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
</web-app>
Create Action Class for Login and Logout
package org.websparrow;
import java.util.Map;
import javax.servlet.http.HttpSession;
import org.apache.struts2.ServletActionContext;
import org.apache.struts2.dispatcher.SessionMap;
import org.apache.struts2.interceptor.SessionAware;
import com.opensymphony.xwork2.ActionSupport;
public class LoginAction extends ActionSupport implements SessionAware {
private static final long serialVersionUID = -3434561352924343132L;
// Generate getters and setters....
private String userId, userPass, msg;
private SessionMap<String, Object> sessionMap;
@Override
public void setSession(Map<String, Object> map) {
sessionMap = (SessionMap<String, Object>) map;
}
@Override
public String execute() throws Exception {
HttpSession session = ServletActionContext.getRequest().getSession(true);
if (userId != null) {
if (userPass.equals("websparrow")) {
// add the attribute in session
sessionMap.put("userId", userId);
return "SUCCESS";
} else {
msg = "Invalid Password";
return "LOGIN";
}
} else {
String getSessionAttr = (String) session.getAttribute("userId");
if (getSessionAttr != null) {
return "SUCCESS";
} else {
return "LOGIN";
}
}
}
public String logout() {
sessionMap.remove("userId");
sessionMap.invalidate();
return "LOGOUT";
}
}
Map Action Class in struts.xml
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE struts PUBLIC
"-//Apache Software Foundation//DTD Struts Configuration 2.0//EN"
"http://struts.apache.org/dtds/struts-2.0.dtd">
<struts>
<constant name="struts.devMode" value="true" />
<package name="default" extends="struts-default" namespace="/">
<action name="sessionman" class="org.websparrow.LoginAction" method="execute">
<result name="SUCCESS">/wel.jsp</result>
<result name="LOGIN">/index.jsp</result>
</action>
<action name="logout" class="org.websparrow.LoginAction" method="logout">
<result name="LOGOUT">/index.jsp</result>
</action>
</package>
</struts>
Create the View Component for Result
<%@taglib uri="/struts-tags" prefix="s"%>
<!DOCTYPE html>
<html>
<body style="margin-left: 30px; background-color: lightyellow;">
<h2>Struts2 login & logout example with Session Management</h2>
<s:form action="sessionman">
<s:textfield name="userId" label="User ID" />
<s:password name="userPass" label="Password" />
<s:submit value="Login" />
</s:form>
<s:property value="msg" />
</body>
</html>
<%@taglib uri="/struts-tags" prefix="s"%>
<!DOCTYPE html>
<html>
<body>
<h2>Struts2 login & logout example with Session Management</h2>
Hello, <s:property value="#session.userId" /> | <a href="logout">Logout</a>
</body>
</html>
Test Application :
To test your application follow the below steps
- Run your application using URL localhost:8080/Struts2SessionManagementExp/ and Login with credentials.
- If you logged in successfully that means session created and you will be redirected to welcome page.
- Now copy the URL localhost:8080/Struts2SessionManagementExp/sessionman and run it on different tab. You will be redirected to the same page.
- Now hit the logout button. It will invalidate the session and redirected to the login page.
- Repeat the Step 3 again. You will be redirected to the login page because there is no session.
- Done. Thank You.
Download Source Code – login-and-logout-using-session-in-struts2.zip