How to display Image in JSP from database using Struts 2
In this Struts 2 tutorials we are going to retrieve the images from database and display on JSP pages. As we know that to store the images in database we use the BLOB
datatype. To retrieve the images we convert it into byte [] array
and write into OutputStream
.
Code Snippet :
Blob ph = rs.getBlob("image");
byte data[] = ph.getBytes(1, (int) ph.length());
OutputStream out = response.getOutputStream();
out.write(data);
Software Used
- Eclipse IDE
- MySQL Database
- JDK 8
- Tomcat 8
Required JARS
To retrieve the image from database these JARS are required.
- All struts 2 core jars
- commons-fileupload-x.x.x.jar
Project Structure in Eclipse
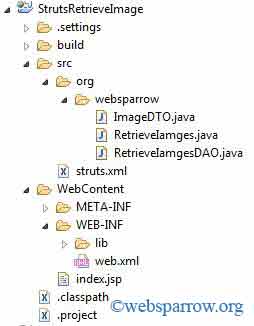
Step 1: Add the struts 2 jars in lib folder.
Step 2: Add the struts 2 filter in web.xml.
Step 3: Create DTO class for the getter and setter of the fields.
package org.websparrow;
import java.sql.Blob;
public class ImageDTO {
int id;
String name;
Blob image;
// generate getters and setters
}
Step 4: Create DAO calss for access the database.
package org.websparrow;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
public class RetrieveIamgesDAO {
public Connection connect() throws Exception {
Class.forName("com.mysql.jdbc.Driver");
return (Connection) DriverManager.getConnection("jdbc:mysql://127.0.0.1:3306/websparrow", "root", "");
}
public ResultSet getData() {
try {
return connect().prepareStatement("SELECT NAME,ID FROM image_upload").executeQuery();
} catch (Exception e) {
e.printStackTrace();
return null;
}
}
public ResultSet getPhoto(String id) {
try {
PreparedStatement ps = connect().prepareStatement("SELECT IMAGE FROM image_upload WHERE ID=?");
ps.setString(1, id);
return ps.executeQuery();
} catch (Exception e) {
e.printStackTrace();
return null;
}
}
}
Step 5: Create the Action Class to handle the data.
package org.websparrow;
import java.io.OutputStream;
import java.sql.Blob;
import java.sql.ResultSet;
import java.util.ArrayList;
import java.util.List;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.apache.struts2.ServletActionContext;
import com.opensymphony.xwork2.ActionSupport;
public class RetrieveIamges extends ActionSupport {
List<ImageDTO> dataList = null;
ResultSet rs = null;
RetrieveIamgesDAO daoObj = null;
ImageDTO dataBean = null;
@Override
public String execute() throws Exception {
HttpServletResponse response = ServletActionContext.getResponse();
HttpServletRequest request = ServletActionContext.getRequest();
String id = request.getParameter("id");
try {
dataList = new ArrayList<>();
rs = new RetrieveIamgesDAO().getData();
if (rs != null) {
while (rs.next()) {
dataBean = new ImageDTO();
dataBean.setId(rs.getInt("id"));
dataBean.setName(rs.getString("name"));
dataList.add(dataBean);
}
}
try {
rs = new RetrieveIamgesDAO().getPhoto(id);
if (rs.next()) {
Blob ph = rs.getBlob("image");
byte data[] = ph.getBytes(1, (int) ph.length());
OutputStream out = response.getOutputStream();
out.write(data);
out.flush();
out.close();
}
} catch (Exception e) {
e.printStackTrace();
e.getMessage();
}
} catch (Exception e) {
e.printStackTrace();
}
return "SUCCESS";
}
public List<ImageDTO> getDataList() {
return dataList;
}
public void setDataList(List<ImageDTO> dataList) {
this.dataList = dataList;
}
}
Step 6: Map the action in struts.xml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE struts PUBLIC
"-//Apache Software Foundation//DTD Struts Configuration 2.0//EN"
"http://struts.apache.org/dtds/struts-2.0.dtd">
<struts>
<constant name="struts.devMode" value="true" />
<package name="default" extends="struts-default" namespace="/">
<action name="Image" class="org.websparrow.RetrieveIamges">
<result name="SUCCESS">/index.jsp</result>
</action>
</package>
</struts>
Step 7: User interface page
<%@taglib prefix="s" uri="/struts-tags"%>
<!DOCTYPE html>
<html>
<head>
<title>Struts 2 image retrieve example- Websparrow.org</title>
<style>
table, th, td {
border: 1px solid black;
border-collapse: collapse;
}
</style>
</head>
<body style="background-color: #f8f8f8; margin-left: 100px; margin-top: 20px; font-size: 20px;">
<h1>Struts 2 retrieve Image from Database example</h1>
<s:form action="Image" method="POST">
<button type="submit">Fetch Records</button>
</s:form>
<div style="margin-top: 40px; margin-right: 100px;">
<table style="width: 90%; text-align: center;">
<thead>
<tr style="background-color: #E0E0E1;">
<th>ID</th>
<th>NAME</th>
<th>Image</th>
</tr>
</thead>
<s:iterator value="dataList">
<tr>
<td>
<s:property value="id" />
</td>
<td>
<s:property value="name" />
</td>
<td>
<img width="100" height="100" src="<s:url value='Image.action?id='/><s:property value='id' />">
</td>
</tr>
</s:iterator>
</table>
</div>
</body>
</html>
Output:
Now you are all done. Run your application.
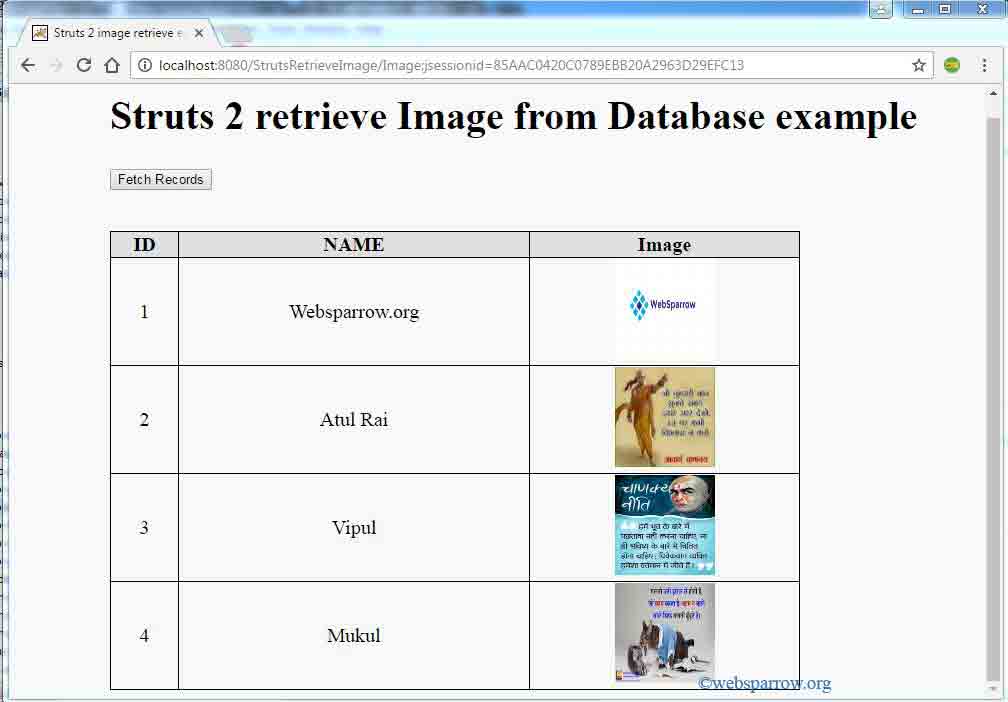
Download Source Code – how-to-display-image-in-jsp-from-database-using-struts2.zip