How to consume RESTful web service using jQuery
On this page, you will learn how to consume RESTful web service using jQuery. jQuery is the most popular JavaScript library used worldwide for client-side validate, calling ajax, etc.
Related Post: Spring Boot RESTful Web Service Example
The jQuery client will be accessed by opening the consume-restful-webservice-jquery.html file in your browser, and will consume the service accepting requests at:
http://rest-service.guides.spring.io/greeting
The service will respond with a JSON representation of a greeting:
{
"id": 166,
"content": "Hello, World!"
}
The jQuery client will render the ID and content into the DOM.
consume-rest.js
$(document).ready(function () {
$.ajax({
url: "http://rest-service.guides.spring.io/greeting",
success: function (data) {
$("#id").append(data.id);
$("#content").append(data.content);
},
error: function (xhr, ajaxOptions, thrownError) {
alert(xhr.responseText + "\n" + xhr.status + "\n" + thrownError);
}
});
});
Create the HTML page that will load the client into the user’s web browser:
consume-restful-webservice-jquery.html
<!DOCTYPE html>
<html>
<head>
<title>How to consume RESTful Web Service using jQuery</title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<script src="js/consume-rest.js"></script>
</head>
<body>
<div>
<h3>How to consume RESTful Web Service using jQuery</h3>
<p id="id">ID: </p>
<p id="content">Content: </p>
</div>
</body>
</html>
Run the HTML file in your favorite web browser and you will get the result as shown below.
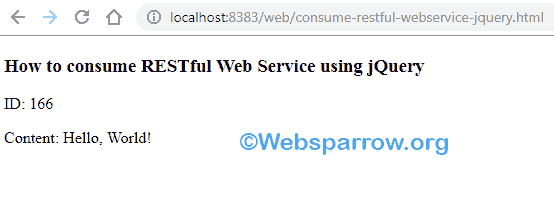