How to get the value of selected option in a select box using jQuery
In this example, we are going to help to get the value of the selected option in the select box using jQuery. jQuery provides two methods to get the value of the selected option from the select box.
1- val()
– method returns the value of selected attribute value.
var selectedVal = $("#myselect option:selected").val();
2- text()
– method return String of selected option.
var selectedVal = $("#myselect option:selected").text();
Let’s understand with the help of an image.
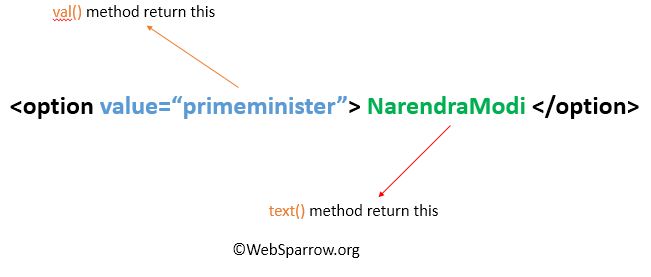
Value of single selected option
JS Snippet
$(document).ready(function() {
$("#myselect").change(function() {
// var selectedVal = $("#myselect option:selected").text();
var selectedVal = $("#myselect option:selected").val();
alert("Hi, your favorite programming language is " + selectedVal);
});
});
Check the full example
<!DOCTYPE html>
<html>
<head>
<script src="js/jquery-3.1.1.min.js"></script>
<script>
$(document).ready(function () {
$("#myselect").change(function () {
// var selectedVal = $("#myselect option:selected").text();
var selectedVal = $("#myselect option:selected").val();
alert("Hi, your favorite programming language is " + selectedVal);
});
});
</script>
</head>
<body>
<h1>Get the value of selected option in a select box using jQuery</h1>
<label>Select Programming Language:</label>
<select id="myselect">
<option value="Java">Java</option>
<option value="C">C</option>
<option value="PHP">PHP</option>
<option value="C#">C#</option>
<option value="C++">C++</option>
</select>
</body>
</html>
Value of multiple selected option
JS Snippet
$(document).ready(function() {
$("button").click(function() {
var wbst = [];
$.each($("#multiselected option:selected"), function() {
wbst.push($(this).val());
});
alert("Your favorite website is - " + wbst.join(", "));
});
});
Check the full example
<!DOCTYPE html>
<html>
<head>
<script src="js/jquery-3.1.1.min.js"></script>
<script type="text/javascript">
$(document).ready(function () {
$("button").click(function () {
var wbst = [];
$.each($("#multiselected option:selected"), function () {
wbst.push($(this).val());
});
alert("Your favorite website is - " + wbst.join(", "));
});
});
</script>
</head>
<body>
<h1>Get the value of multiple selected option in a select box using jQuery</h1>
<form>
<label>Select Website :</label>
<select id="multiselected" multiple="multiple" size="3">
<option value="websparrow.org">websparrow.org</option>
<option value="google.com">google.com</option>
<option value="facebook.com">facebook.com</option>
<option value="twitter.com">twitter.com</option>
<option value="linkedin.com">linkedin.com</option>
</select>
<button type="button">Get Values</button>
</form>
</body>
</html>