Spring Task Scheduler Example using @Scheduled Annotation
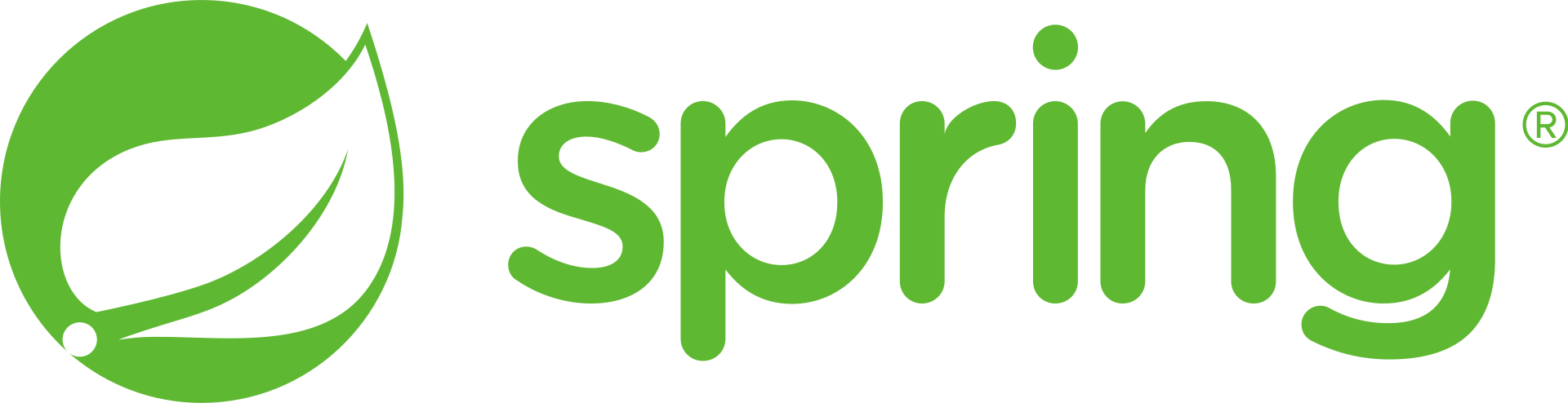
This guide walks you through the steps for scheduling tasks with Spring. We can schedule the execution of the task using @Scheduled
annotation. The @Scheduled
annotation applied at the method level. It accepts the below condition on which Spring scheduler executes the task but before scheduled a task we need to enable scheduling.
1. Enable Scheduling
In Spring or Spring Boot application, enabling task scheduling is as simple as eating a piece of cake. It can be done just by adding @EnableScheduling
annotation at the class level.
@SpringBootApplication
@EnableScheduling
public class SchedulerApp {
...
}
2. Schedule a Task using Cron Expressions
cron
is from UNIX cron utility which gives the flexibility of a cron expression to control the schedule of our tasks:
@Scheduled(cron = "0 * * * * MON-FRI")
public void task() {
System.out.println("The current date & time is: " + LocalDateTime.now());
}
Above cron
expression executes the task()
method from Monday to Friday at every one minute. Similarly, below cron
expression executes the task()
method at every 3 seconds interval. Check out more cron expressions.
@Scheduled(cron = "*/3 * * * * ?")
public void task() {
System.out.println("The current date & time is: " + LocalDateTime.now());
}
3. Schedule a Task at Fixed Delay
fixedDelay
specify the execution control with a fixed period in milliseconds between the end of the last invocation and the start of the next. The task always waits until the previous one is finished.
@Scheduled(fixedDelay = 1500)
public void task() {
System.out.println("The current date & time is: " + LocalDateTime.now());
}
4. Schedule a Task at a Fixed Rate
fixedRate
execute the task with a fixed period in milliseconds between invocations even if the last invocation may be still running. fixedRate
option should be used when each execution of the task is independent.
@Scheduled(fixedRate = 2000)
public void task() {
System.out.println("The current date & time is: " + LocalDateTime.now());
}
Now let’s jump to the actual piece of coding but before starting it, we need to set up the project, add the Spring dependency and required tools and technology.
Technologies Used
Find the list of all technologies used in this application.
- Spring Tool Suite 4
- JDK 8
- Spring Boot 2.1.7.RELEASE
Dependencies Required
There is no requirement of any special plugins or JAR, spring-boot-starter is enough for it.
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.1.7.RELEASE</version>
<relativePath /> <!-- lookup parent from repository -->
</parent>
<groupId>org.websparrow</groupId>
<artifactId>spring-scheduler</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>spring-scheduler</name>
<description>Demo project for Spring Scheduler</description>
<properties>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
Add @EnableScheduling Annotation
Annotate the Spring starter class by adding @EnableScheduling
annotation to enable scheduling functionality in the application.
package org.websparrow;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.scheduling.annotation.EnableScheduling;
@SpringBootApplication
@EnableScheduling
public class SchedulerApp {
public static void main(String[] args) {
SpringApplication.run(SchedulerApp.class, args);
}
}
Task which will be scheduled
Create a MyScheduledTask
class which contains all those methods which are executed at some interval by Spring scheduler.
package org.websparrow.task;
import java.time.LocalDateTime;
import org.springframework.scheduling.annotation.Scheduled;
import org.springframework.stereotype.Component;
@Component
public class MyScheduledTask {
// Remove the comments from the method one by one to test it.
@Scheduled(cron = "*/3 * * * * ?")
public void task1() {
System.out.println("The current date & time is: " + LocalDateTime.now());
}
/*
@Scheduled(fixedDelay = 2000)
public void task2() {
System.out.println("The current date & time is: " + LocalDateTime.now());
}
*/
/*
@Scheduled(fixedRate = 1500)
public void task3() {
System.out.println("The current date & time is: " + LocalDateTime.now());
}
*/
}
Now run the application and you can see the task1()
will execute at every 3 seconds and print the following the console log:
The current date & time is: 2019-08-15T21:56:00.027
The current date & time is: 2019-08-15T21:56:03.001
The current date & time is: 2019-08-15T21:56:06.001
The current date & time is: 2019-08-15T21:56:09.001
The current date & time is: 2019-08-15T21:56:12.001
The current date & time is: 2019-08-15T21:56:15.001
execution will continue...
Download Source Code: spring-task-scheduler-example-using-scheduled-annotation.zip