How to get user details in Spring Security
This article will focus on how to retrieve the user details in Spring Security. To get current logged-in user details like username and role Spring Security provide an Authentication
interface.
It represents the token for an authentication request or for an authenticated principal once the request has been processed by the authenticate(Authentication authentication)
method of AuthenticationManager
.
Let’s jump to the actual part of coding.
1. Create some dummy users:
@Configuration
@EnableWebSecurity
public class SecurityConfiguration extends WebSecurityConfigurerAdapter {
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.inMemoryAuthentication()
.withUser("manish")
.password(passwordEncoder().encode("admin@123")).roles("ADMIN")
.and()
.withUser("sandeep")
.password(passwordEncoder().encode("user@123")).roles("USER");
}
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
}
2. Controller class to fetch logged-in user information:
@RestController
public class UserController {
@GetMapping("/user")
public String userInfo(Authentication authentication) {
String userName = authentication.getName();
String role = authentication.getAuthorities().stream()
.findAny().get().getAuthority();
return "Your user name is: " + userName + " and your role is: " + role;
}
}
2.1 User authority/role can retrieve by user Enhanced-For-Loop also:
String role = "";
for (GrantedAuthority authority : authentication.getAuthorities()) {
role = authority.getAuthority();
}
Alternatively, we can also use the getPrincipal()
method:
UserDetails userDetails = (UserDetails) authentication.getPrincipal();
System.out.println("User has authorities: " + userDetails.getAuthorities());
Output:
After the successful login, you will get the current logged-in user details as shown below:
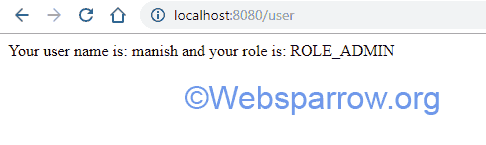
References
- Getting Started with Spring Security
- Spring Security Role Based Authorization Example
- Spring Boot + Spring Security with JPA authentication and MySQL