Struts 2 File Upload Example
In this Struts 2 tutorial, we will help you to upload a file in Struts 2 application. In Struts 2, <s:file>
tag is used to create a file upload component. Every file upload request passes through the fileUpload interceptor
. This interceptor help to control the file upload request like controlling file size, file type, etc.
In this example, we have used 2 parameters of fileUpload interceptor
.
- allowedTypes: control the type of file like jpg, zip, txt, etc
- maximumSize: control the size of the file.
Software Used
- Eclipse IDE
- Tomcat 8
- JDK 8
Dependencies Required
To upload the file in your Struts 2 application, you must have below dependencies in your build path.
- Commons-FileUpload
- Commons-IO
If you are using Maven then you can add these libraries as dependencies in your project’s pom.xml
.
<dependency>
<groupId>commons-fileupload</groupId>
<artifactId>commons-fileupload</artifactId>
<version>1.2.1</version>
</dependency>
<dependency>
<groupId>commons-io</groupId>
<artifactId>commons-io</artifactId>
<version>1.3.2</version>
</dependency>
Note: fileUpload interceptor will automatically inject the uploaded file detail while setting the name like imageFileName and imageContentType.
Project Structure in Eclipse
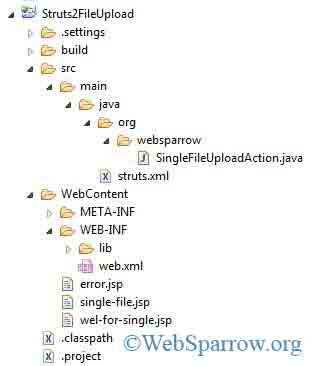
web.xml
Adding the Struts 2 filter in web.xml
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_3_1.xsd" id="WebApp_ID" version="3.1">
<display-name>Struts2FileUpload</display-name>
<welcome-file-list>
<welcome-file>single-file.jsp</welcome-file>
</welcome-file-list>
<filter>
<filter-name>struts2</filter-name>
<filter-class>org.apache.struts2.dispatcher.ng.filter.StrutsPrepareAndExecuteFilter</filter-class>
</filter>
<filter-mapping>
<filter-name>struts2</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
</web-app>
Action Class
Inside the action, we need to declare the input variable and define the business logic or how to handle the incoming file.
package org.websparrow;
import com.opensymphony.xwork2.ActionSupport;
import java.io.File;
import org.apache.commons.io.FileUtils;
import org.apache.struts2.ServletActionContext;
public class SingleFileUploadAction extends ActionSupport {
private static final long serialVersionUID = -7116807617477612442L;
private File img;
private String imgContentType;
private String imgFileName;
@Override
public String execute() throws Exception {
try {
// get the context path to save the file
String path = ServletActionContext.getServletContext().getRealPath("/");
System.out.println("Image Location:" + path);
// handling the file
File file = new File(path, imgFileName);
FileUtils.copyFile(img, file);
} catch (Exception e) {
e.printStackTrace();
}
return "SUCCESS";
}
public File getImg() {
return img;
}
public void setImg(File img) {
this.img = img;
}
public String getImgContentType() {
return imgContentType;
}
public void setImgContentType(String imgContentType) {
this.imgContentType = imgContentType;
}
public String getImgFileName() {
return imgFileName;
}
public void setImgFileName(String imgFileName) {
this.imgFileName = imgFileName;
}
}
JSP Page
Define your user interface page and define the enctype="multipart/form-data"
in form attribute.
Note: enctype=”multipart/form-data” must defined with
method="POST"
.
<%@taglib prefix="s" uri="/struts-tags"%>
<!DOCTYPE html>
<html>
<head>
<title>Struts 2 File Upload Example</title>
</head>
<body>
<h1>Struts 2 File Upload Example</h1>
<s:form action="SingleFileUpload" method="post" enctype="multipart/form-data">
<s:file name="img" label="Select Image"></s:file>
<s:submit value="Upload"></s:submit>
</s:form>
</body>
</html>
Displaying the SUCCESS and ERROR response.
<%@ taglib prefix="s" uri="/struts-tags"%>
<html>
<body>
<h2>Struts 2 File Upload Example</h2>
Image:
<s:property value="img" />
<br />
Content Type:
<s:property value="imgContentType" />
<br />
Uploaded Image:
<br />
<img src="<s:property value='imgFileName'/>" width="500" height="400" />
</body>
</html>
<%@taglib prefix="s" uri="/struts-tags"%>
<!DOCTYPE html>
<html>
<head>
</head>
<body>
<h1>Error</h1>
<s:fielderror />
</body>
</html>
Struts.xml
Inside the struts.xml we need to map the SUCCESS and ERROR responses and fileUpload interceptor
.
<struts>
<package name="default" extends="struts-default">
<action name="SingleFileUpload" class="org.websparrow.SingleFileUploadAction">
<interceptor-ref name="fileUpload">
<param name="maximumSize">2097152</param>
<param name="allowedTypes">
image/png,image/gif,image/jpeg,image/pjpeg
</param>
</interceptor-ref>
<interceptor-ref name="defaultStack"></interceptor-ref>
<result name="SUCCESS">wel-for-single.jsp</result>
<result name="input">error.jsp</result>
</action>
</package>
</struts>
Output:
Now you are done and run you application and make sure you are allowed to upload png, gif, jpeg, pjpeg type of file and maximum size of the file is 2MB.
If images are not in dedicated directories, go to image location printed in console.
Screen 1
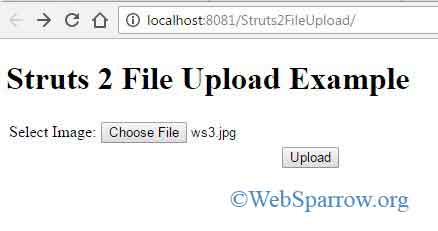
Screen 2
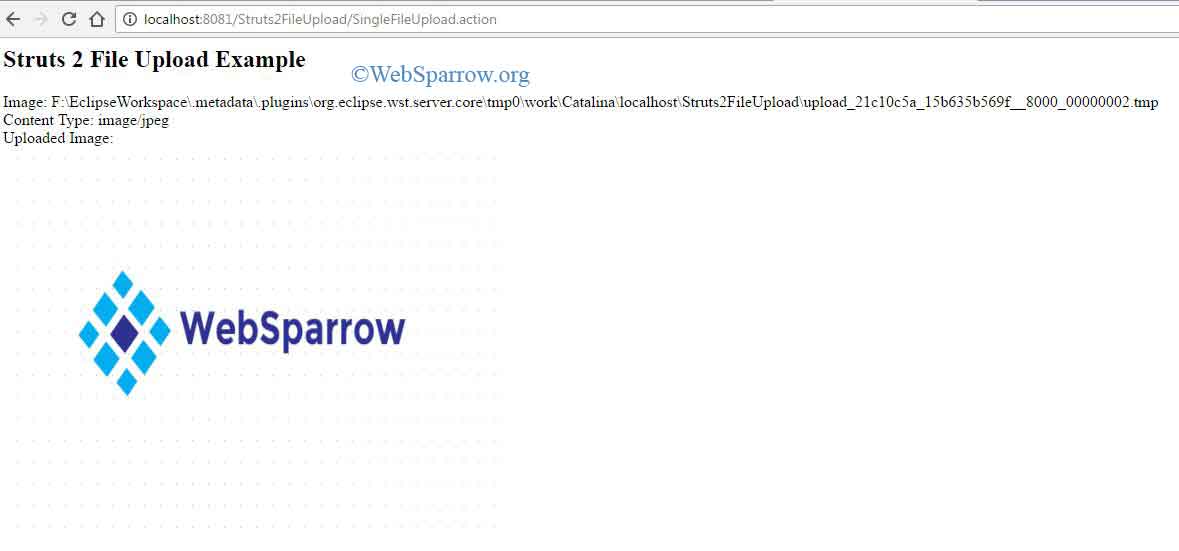
Screen 3
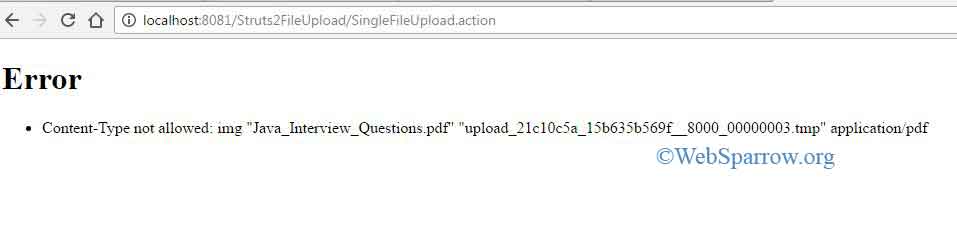
Download Source Code – struts2-file-upload-example.zip