Base64Encoder- How to display byte array image in JSP using Struts2
Retrieving images from database and display it on JSP page is a little bit tricky but we have many options to do that. In my previous Struts example (How to display Image in JSP from database using Struts 2), we have used the Servlet
and Struts tag
for display the images from the database.
In this Struts 2 example, we are going to use BASE64Encoder
class to encode the image byte array data into String
. This class implements an encoder for encoding byte data using the Base64 encoding scheme as specified in RFC 4648 and RFC 2045.
Project Structure in Eclipse
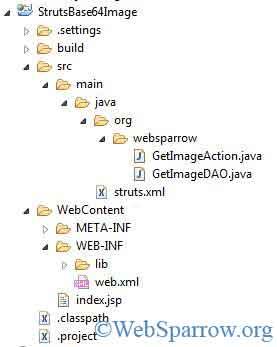
Create the database connection class and method to communicate with the database and fetch the image from the database.
package org.websparrow;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
public class GetImageDAO {
// method for communicate with database - MySQL
public static Connection connect() throws Exception {
Class.forName("com.mysql.jdbc.Driver");
return (Connection) DriverManager.getConnection("jdbc:mysql://127.0.0.1:3306/websparrow", "root", "");
}
// method for fetching the data from database
public static ResultSet restriveImage(String imgId) {
ResultSet rs1 = null;
try {
String query = "SELECT name,image FROM image_upload WHERE id=?";
PreparedStatement ps = connect().prepareStatement(query);
ps.setString(1, imgId);
rs1 = ps.executeQuery();
return rs1;
} catch (Exception e) {
e.printStackTrace();
return null;
} finally {
try {
if (connect() != null) {
connect().close();
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
}
Now create the action class.
package org.websparrow;
import java.sql.Blob;
import java.sql.ResultSet;
import com.opensymphony.xwork2.ActionSupport;
import sun.misc.BASE64Encoder;
public class GetImageAction extends ActionSupport {
private String imgId, name, image;
ResultSet rs = null;
@Override
public String execute() throws Exception {
rs = org.websparrow.GetImageDAO.restriveImage(imgId);
if (rs != null) {
while (rs.next()) {
name = rs.getString("name");
Blob ph = rs.getBlob("image");
byte data[] = ph.getBytes(1, (int) ph.length());
// encoding the byte image string
BASE64Encoder base64Encoder = new BASE64Encoder();
StringBuilder imageString = new StringBuilder();
imageString.append("data:image/png;base64,");
imageString.append(base64Encoder.encode(data));
image = imageString.toString();
}
}
return "SUCCESS";
}
public String getImgId() {
return imgId;
}
public void setImgId(String imgId) {
this.imgId = imgId;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getImage() {
return image;
}
public void setImage(String image) {
this.image = image;
}
}
Map the action class in struts.xml
<struts>
<constant name="struts.devMode" value="true" />
<package name="default" extends="struts-default" namespace="/">
<action name="getImage" class="org.websparrow.GetImageAction">
<result name="SUCCESS">/index.jsp</result>
</action>
</package>
</struts>
Create the JSP page.
<%@taglib prefix="s" uri="/struts-tags"%>
<!DOCTYPE html>
<html>
<head>
<title>Display image in JSP using Base64</title>
<style>
table, th, td {
border: 1px solid black;
border-collapse: collapse;
}
</style>
</head>
<body>
<div style="margin-left: 15%; margin-top: 20px;">
<h2>How to display byte image in JSP using Struts2</h2>
<form action="getImage">
ID: <input type="text" name="imgId" value="<s:property value='imgId'/>" placeholder="Enter the Id no..." />
<button type="submit">Get Image</button>
</form>
<table style="width: 60%; margin-top: 30px; text-align: center;">
<tr>
<th>Name</th>
<th>Image</th>
</tr>
<tr>
<td><i><s:property value="name" /></i></td>
<td><img width="100" height="100" src="<s:property value="image" />"></td>
</tr>
</table>
</div>
</body>
</html>
Output:
Finally, all is done, now run the application and enter the value in the input box.
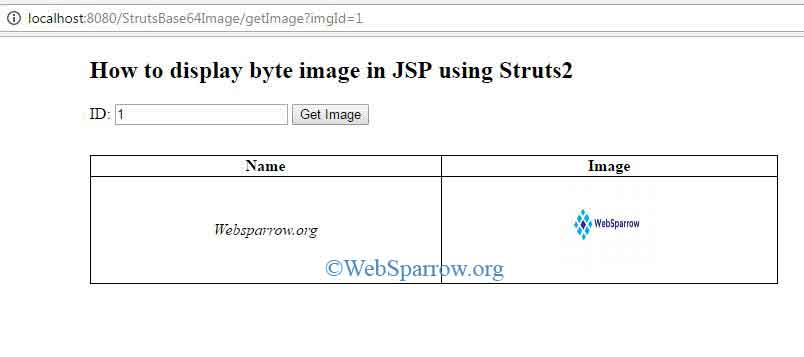
Download Source Code – base64encoder-how-to-display-byte-array-image-in-jsp-using-struts2.zip