Sending email using Struts 2 and JavaMail API
In this tutorial, you will learn how to send an email in your Struts 2 application using JavaMail API
via Gmail SMTP. JavaMail API provides a platform-independent and protocol-independent framework to build mail and messaging applications.
Required Library: In this example, you need to add below libraries in your project build path.
If your project is Maven based you can add the dependency in your pom.xml.
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.websparrow</groupId>
<artifactId>Test</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>war</packaging>
<dependencies>
<dependency>
<groupId>org.apache.struts</groupId>
<artifactId>struts2-core</artifactId>
<version>2.3.20</version>
</dependency>
<dependency>
<groupId>javax.mail</groupId>
<artifactId>mail</artifactId>
<version>1.4</version>
</dependency>
</dependencies>
<build>
<sourceDirectory>src</sourceDirectory>
<plugins>
<plugin>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.3</version>
<configuration>
<source>1.8</source>
<target>1.8</target>
</configuration>
</plugin>
<plugin>
<artifactId>maven-war-plugin</artifactId>
<version>2.6</version>
<configuration>
<warSourceDirectory>WebContent</warSourceDirectory>
<failOnMissingWebXml>false</failOnMissingWebXml>
</configuration>
</plugin>
</plugins>
</build>
</project>
Note: You may face the
javax.mail.AuthenticationFailedException
exception. To resolve this check this tutorial javax.mail.AuthenticationFailedException
Software Used
- Eclipse IDE
- Tomcat 8
- JDK 8
Project Structure in Eclipse
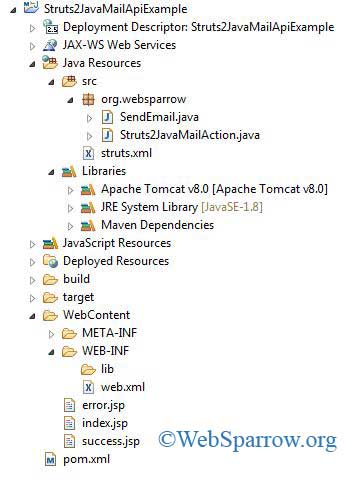
Add Struts 2 Filter
Define the struts 2 filters into web.xml
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" id="WebApp_ID" version="2.5">
<display-name>Struts2JavaMailApiExample</display-name>
<welcome-file-list>
<welcome-file>index.jsp</welcome-file>
</welcome-file-list>
<filter>
<filter-name>struts2</filter-name>
<filter-class>org.apache.struts2.dispatcher.ng.filter.StrutsPrepareAndExecuteFilter</filter-class>
</filter>
<filter-mapping>
<filter-name>struts2</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
</web-app>
Create Action Class
The next step is creating the Action Class that control the sending of email. In this Action class, we have to call the method sendMail of SendEmail class and passes the parameter.
package org.websparrow;
import com.opensymphony.xwork2.ActionSupport;
public class Struts2JavaMailAction extends ActionSupport {
private static final long serialVersionUID = 8676674317393590961L;
// getter and setters...
private String senderEmail, senderPassword, receiverEmail, subject, message;
SendEmail obj = null;
int resp = 0;
@Override
public String execute() throws Exception {
obj = new SendEmail();
resp = obj.sendMail(senderEmail, senderPassword, receiverEmail, subject, message);
if (resp == 1) {
return "SUCCESS";
} else {
return "ERROR";
}
}
}
Now create a class SendMail which has a method sendMail requires the below parameters.
- from – Email address of the sender. Gmail Account
- password – Password of above email.
- to – Email address of the receiver.
- subject – Subject of the email.
- msg – Contents of the message.
package org.websparrow;
import java.util.Properties;
import javax.mail.Message;
import javax.mail.MessagingException;
import javax.mail.PasswordAuthentication;
import javax.mail.Session;
import javax.mail.Transport;
import javax.mail.internet.InternetAddress;
import javax.mail.internet.MimeMessage;
public class SendEmail {
public int sendMail(String from, String password, String to, String subject, String msg) {
// setting gmail smtp properties
Properties props = new Properties();
props.put("mail.smtp.auth", "true");
props.put("mail.smtp.starttls.enable", "true");
props.put("mail.smtp.host", "smtp.gmail.com");
props.put("mail.smtp.port", "587");
// check the authentication
Session session = Session.getInstance(props, new javax.mail.Authenticator() {
protected PasswordAuthentication getPasswordAuthentication() {
return new PasswordAuthentication(from, password);
}
});
try {
Message message = new MimeMessage(session);
message.setFrom(new InternetAddress(from));
// recipients email address
message.setRecipients(Message.RecipientType.TO, InternetAddress.parse(to));
// add the Subject of email
message.setSubject(subject);
// message body
message.setText(msg);
Transport.send(message);
return 1;
} catch (MessagingException e) {
e.printStackTrace();
return 0;
}
}
}
Create View Component
In this example, we have created 3 JSP pages.
index.jsp for taking the input from the user.
success.jsp for email successfully sent.
error.jsp for error message if any.
<%@taglib prefix="s" uri="/struts-tags"%>
<html>
<head>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css" />
<title>Sending email using Struts 2 and JavaMail API</title>
<style type="text/css">
.jumbotron {
background: #358CCE;
color: #FFF;
border-radius: 0px;
}
.jumbotron-sm {
padding-top: 24px;
padding-bottom: 24px;
}
.jumbotron small {
color: #FFF;
}
.h2 small {
font-size: 18px;
}
</style>
</head>
<body>
<div class="jumbotron jumbotron-sm">
<div class="container">
<div class="row">
<div class="col-sm-12 col-lg-12">
<h2 class="h2">
WebSparrow.org <small>Sending email using Struts 2 and JavaMail API</small>
</h2>
</div>
</div>
</div>
</div>
<div class="container">
<div class="row">
<div class="col-md-8">
<div class="well well-sm">
<form action="sendemail" method="post">
<div class="row">
<div class="col-md-6">
<div class="form-group">
<label for="senderEmail">Login</label> <input type="text" name="senderEmail" class="form-control" id="senderEmail" placeholder="Sender email" required="required" />
<br> <input type="password" class="form-control" id="senderPassword" placeholder="Password" name="senderPassword" required="required" />
</div>
<div class="form-group">
<label for="receiverEmail">Receiver Email</label> <input type="text" name="receiverEmail" class="form-control" id="receiverEmail" placeholder="Receiver email"
required="required" />
</div>
<div class="form-group">
<label for="subject">Subject</label> <input type="text" class="form-control" id="subject" name="subject" placeholder="Subject" required="required" />
</div>
</div>
<div class="col-md-6">
<div class="form-group">
<label for="message"> Message</label>
<textarea name="message" id="message" class="form-control" rows="11" cols="25" required="required" placeholder="Message"></textarea>
</div>
</div>
<div class="col-md-12">
<button type="submit" class="btn btn-primary pull-right" id="btnContactUs">Send Message</button>
</div>
</div>
</form>
</div>
</div>
</div>
</div>
</body>
</html>
<html>
<head>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css" />
<title>Sending email using Struts 2 and JavaMail API</title>
<style type="text/css">
.jumbotron {
background: #358CCE;
color: #FFF;
border-radius: 0px;
}
.jumbotron-sm {
padding-top: 24px;
padding-bottom: 24px;
}
.jumbotron small {
color: #FFF;
}
.h2 small {
font-size: 18px;
}
</style>
</head>
<body>
<div class="jumbotron jumbotron-sm">
<div class="container">
<div class="row">
<div class="col-sm-12 col-lg-12">
<h2 class="h2">
WebSparrow.org <small>Sending email using Struts 2 and JavaMail API</small>
</h2>
</div>
</div>
</div>
</div>
<div class="container">
<p style="color: green;">Email Sent Successfully</p>
</div>
</body>
</html>
<html>
<head>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css" />
<title>Sending email using Struts 2 and JavaMail API</title>
<style type="text/css">
.jumbotron {
background: #358CCE;
color: #FFF;
border-radius: 0px;
}
.jumbotron-sm {
padding-top: 24px;
padding-bottom: 24px;
}
.jumbotron small {
color: #FFF;
}
.h2 small {
font-size: 18px;
}
</style>
</head>
<body>
<div class="jumbotron jumbotron-sm">
<div class="container">
<div class="row">
<div class="col-sm-12 col-lg-12">
<h2 class="h2">
WebSparrow.org <small>Sending email using Struts 2 and JavaMail API</small>
</h2>
</div>
</div>
</div>
</div>
<div class="container">
<p style="color: red;">Some error occurred. For more info check the console.</p>
</div>
</body>
</html>
Configuration File
Create the struts.xml file in the source package and map the Action class.
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE struts PUBLIC
"-//Apache Software Foundation//DTD Struts Configuration 2.0//EN"
"http://struts.apache.org/dtds/struts-2.0.dtd">
<struts>
<constant name="struts.devMode" value="true" />
<package name="default" namespace="/" extends="struts-default">
<action name="sendemail" class="org.websparrow.Struts2JavaMailAction">
<result name="SUCCESS">/success.jsp</result>
<result name="ERROR">/error.jsp</result>
</action>
</package>
</struts>
Output:
To test the application start Tomcat server deploy your project and check your email. Find the screenshot of the application.
Fill out the details.
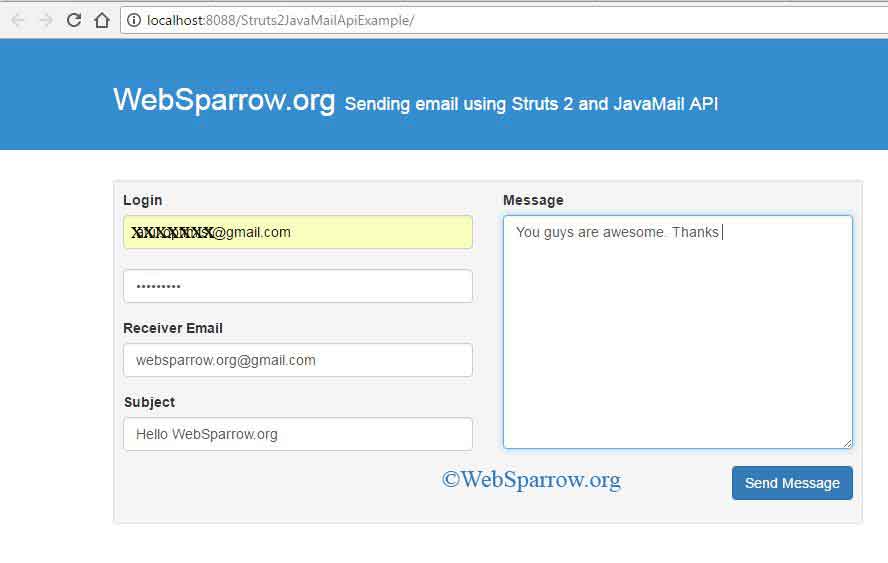
If everything is OK, you will be redirected to the success page.
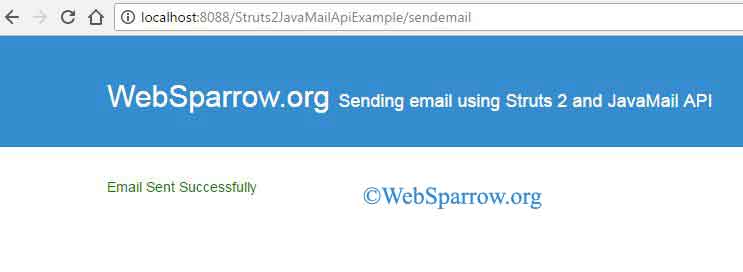
If there is any problem, you will be redirected to the error page. To test this I have entered the wrong password.
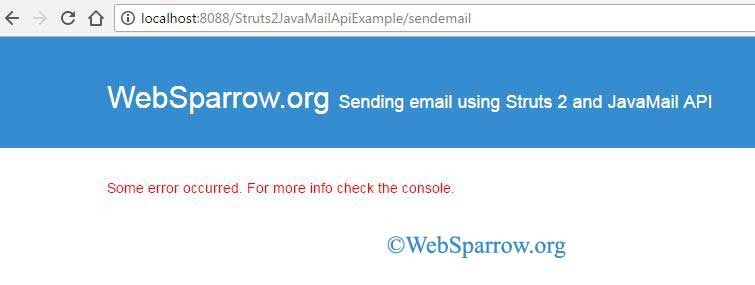
Download Source Code – sending-email-using-struts-2-and-javamail-api.zip