How to consume REST APIs in React using Axios
Axios is a popular JavaScript library that simplifies the process of making HTTP requests, including REST API calls, in React. In this article, we’ll explore how to call a REST API in React using Axios.
Prerequisites
To follow along with this tutorial, you should have a basic understanding of React and JavaScript. Make sure you have Node.js and npm (Node Package Manager) installed on your system.
Step 1: Setting Up a React Application
First, let’s create a new React application using Create React App. Open your terminal and execute the following command:
npx create-react-app axios-react-rest-api-call
Once the process is complete, navigate to the newly created project folder:
cd axios-react-rest-api-call
Step 2: Installing Axios
Next, install Axios as a dependency in your project. In the terminal, run the following command:
npm install axios
Axios will now be added to your project’s node_modules
folder.
Step 3: Making API Calls with Axios
Now that we have Axios installed, let’s import it into our React component and make an API call. Open the src/App.js
file in your code editor and replace the existing code with the following:
import React, { useEffect, useState } from 'react';
import axios from 'axios';
const App = () => {
const [data, setData] = useState([]);
useEffect(() => {
const fetchData = async () => {
try {
const USER_API = 'https://jsonplaceholder.typicode.com/users';
const response = await axios.get(USER_API);
setData(response.data);
} catch (error) {
console.error('Error fetching data:', error);
}
};
fetchData();
}, []);
return (
<div>
<h1>React- Calling REST API using Axios</h1>
<ul>
{data.map((item) => (
<li key={item.id}>{item.name}</li>
))}
</ul>
</div>
);
};
export default App;
What we have done here?
- We import React, the
useEffect
anduseState
hooks, and the Axios library. - Inside our functional component
App
, we define a state variabledata
using theuseState
hook. This variable will store the API response data. - In the
useEffect
hook, we define an asynchronous functionfetchData
that makes an HTTP GET request to the specified API endpoint usingaxios.get()
. - The API response is then stored in the
data
state variable usingsetData(response.data)
. - In case of an error, we log the error to the console.
- Finally, in the component’s JSX, we render the fetched data by mapping over the
data
array and displaying each item’sname
property.
Step 4: Running the Application
To see the result, start the development server by running the following command in your terminal:
npm start
This command will start the React development server, and you can view your application in your browser at http://localhost:3000
.
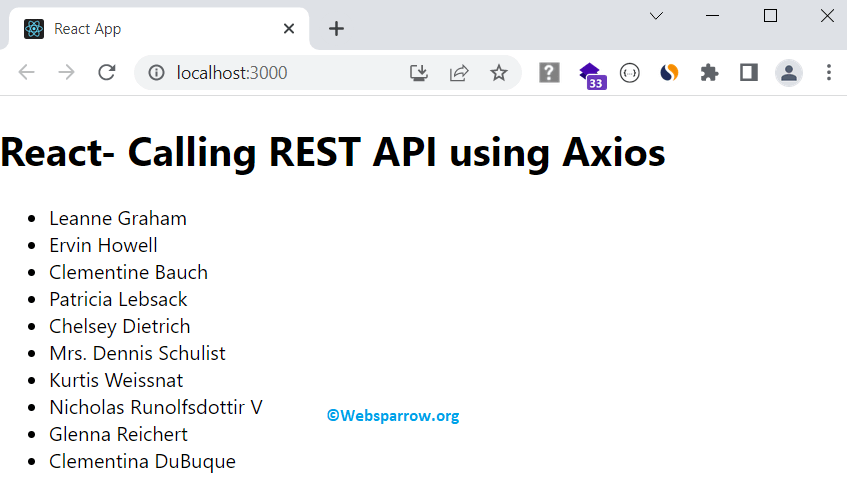
References
- How to call a REST API in React
- React- Getting Started
- React- Introducing Hooks
- Getting Started | Axios Docs