React useState Hook Example
In this blog, we will learn the useState
hook and explore its features and benefits with examples. The useState
hook is a built-in hook in React that enables you to add a state to functional components. It provides a way to declare variables that persist between re-renders of the component, making it easier to manage and update the component state without relying on class components or external state management libraries.
Syntax and Usage
The useState
hook is imported from the ‘react‘ package and can be used in functional components as follows:
import React, { useState } from 'react';
const MyComponent = () => {
const [state, setState] = useState(initialValue);
// Component logic and JSX
return (
// JSX to render
);
};
Here, the useState
function takes an initial value as its parameter and returns an array with two elements: the current state value (state
) and a function to update the state (setState
). The state
variable holds the current value of the state, while setState
is used to update the state.
Creating a Counter Component with useState
Let’s create a simple counter component to demonstrate the usage of the useState
hook:
import React, { useState } from 'react';
const Counter = () => {
const [count, setCount] = useState(0);
const increment = () => {
setCount(count + 1);
};
const decrement = () => {
setCount(count - 1);
};
return (
<div>
<h2>Counter: {count}</h2>
<button onClick={increment}>Increment</button>
<button onClick={decrement}>Decrement</button>
</div>
);
};
export default Counter;
In this example, we initialize the state count
with an initial value of 0 using the useState
hook. The increment
and decrement
functions utilize the setCount
function to update the state by incrementing and decrementing the count
value respectively. The current value of count
is displayed in the JSX using curly braces {}
.
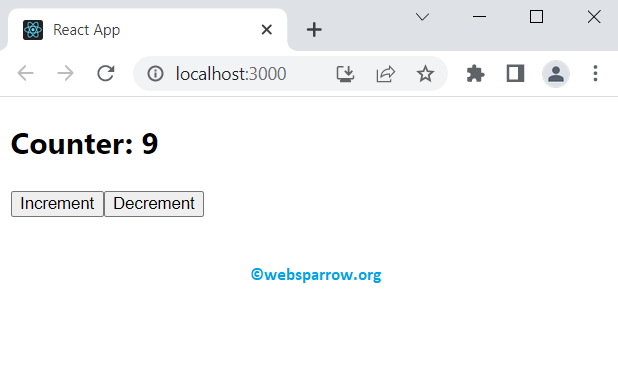
Benefits and Advantages of useState Hook
- Simplicity and Readability: The
useState
hook simplifies state management in functional components, making the code more readable and concise compared to class components. - Improved Performance: React’s
useState
hook uses an optimized mechanism to update state, resulting in better performance compared to traditional class components. - No Need for Constructor or Binding: With the
useState
hook, you can directly declare and use state within your functional component, eliminating the need for a constructor or explicit binding. - Multiple State Variables: You can use the
useState
hook multiple times within a single component to manage different state variables independently. - Easy Migration: The
useState
hook is part of React’s official API and is fully backward compatible. It allows for a smoother migration path for functional components adopting Hooks from class components.