Java var Keyword: Enhancing Code Readability and Flexibility
With the release of Java 10, a 'var'
keyword was introduced. The 'var'
keyword allows developers to declare local variables without explicitly stating their types, while still maintaining strong typing and compile-time checks. In this blog post, we will dive into the 'var'
keyword, understand its benefits, and explore some real-world examples of its usage.
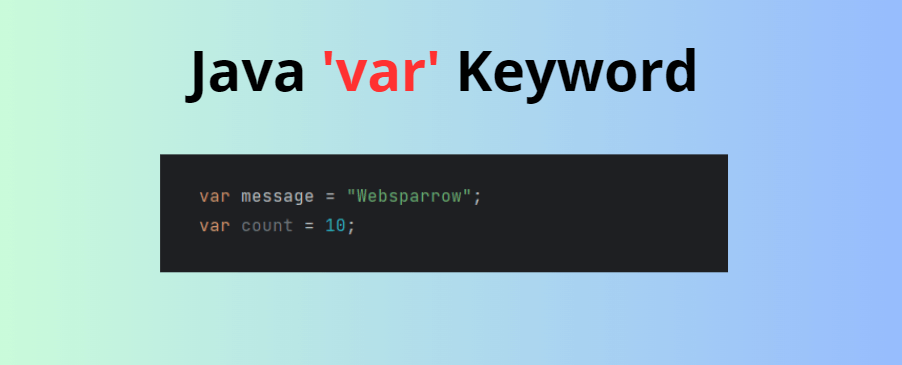
Understanding the 'var'
Keyword
In Java, variable declarations typically include the type followed by the variable name. For example:
String name= "Websparrow";
int count = 10;
With the introduction of the 'var'
keyword, we can rewrite the above code as:
var message = "Websparrow";
var count = 10;
The 'var'
keyword infers the type of the variable based on the expression used to initialize it. The type inference is performed at compile time, ensuring that the code remains strongly typed and that any type-related errors are caught early in the development process.
Advantages of Using the 'var'
Keyword
- Enhanced Code Readability: By using the
'var'
keyword, we can reduce the verbosity of our code, especially when working with complex types. It allows us to focus more on the intent of the code rather than the specific types involved. This can lead to cleaner and more readable code, as demonstrated in the examples below. - Improved Flexibility: The
'var'
keyword promotes flexibility by allowing us to easily change the type of a variable without having to modify its declaration. This can be particularly helpful when refactoring code or when working with APIs that return different types depending on certain conditions.
Let’s explore some practical examples that demonstrate the usage and benefits of the 'var'
keyword:
1. Enhanced Iteration
List<String> names = Arrays.asList("Atul", "Manish", "Sagar");
for (var name : names) {
System.out.println(name);
}
In the above example, the 'var'
keyword eliminates the need to repeat the type 'String'
when iterating over the 'names'
list. This leads to cleaner code without sacrificing type safety.
2. Improved Lambda Expressions
Runnable task = () -> {
var message = "Executing task...";
System.out.println(message);
};
In this example, the 'var'
keyword simplifies the declaration of the 'message'
variable within the lambda expression, making the code more concise and readable.
3. Stream API Usage
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
var sum = numbers.stream()
.filter(n -> n % 2 == 0)
.mapToInt(Integer::intValue)
.sum();
System.out.println("Sum of even numbers: " + sum);
By using the 'var'
keyword in the 'sum'
variable declaration, we avoid repeating the cumbersome 'int'
type while performing a series of operations on the stream.
Also read Limitations of the var Keyword in Java
Summary
The 'var'
keyword introduced in Java 10 has brought a new level of flexibility and readability to the language. By inferring the variable type at compile time, it enables developers to write cleaner and more concise code without sacrificing the benefits of strong typing.
However, it is important to use the 'var'
keyword judiciously, ensuring that code remains clear and maintainable. By incorporating the 'var'
keyword effectively, Java developers can enhance their productivity and overall code quality.