Spring Boot + MongoDB CRUD Example
In this article, we’ll build a Spring Boot REST API which performs the create, read, update, and delete (CRUD) operation using Spring Data and MongoDB database. Spring Data provides a MongoRepository
interface in the package org.springframework.data.mongodb.repository
which contains all the methods necessary for CRUD operation.
Similar Posts:
What we’ll build
We will create a brand new Spring Boot application and expose the following API’s which perform the CRUD operation with the MongoDB database.
HTTP Method | Endpoint | Action |
---|---|---|
POST | api/schools | Save a new school into the database |
GET | api/schools | Fetch all the schools |
PUT | api/schools | Update the existing school |
DELETE | api/schools/:id | Delete the existing school from the database by its ID |
P.S. Every school document has a unique ID.
What we’ll need
- About 30 minute
- JDK 1.8 or later
- Spring Boot 2.3.4.RELEASE
- Spring Data
- Gradle 4+ or Maven 3.2+
- MongoDB 4.4.1 Database
- Your favorite IDE:
- Spring Tool Suite (STS)
- Eclipse
- IntelliJ IDEA
Dependencies Required
Here is the pom.xml file including the required dependencies used in this project.
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.3.4.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>org.websparrow</groupId>
<artifactId>spring-boot-mongodb-crud</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>spring-boot-mongodb-crud</name>
<properties>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-mongodb</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
Project Structure
The final project structure of our application in IntelliJ IDEA will look like as follows:
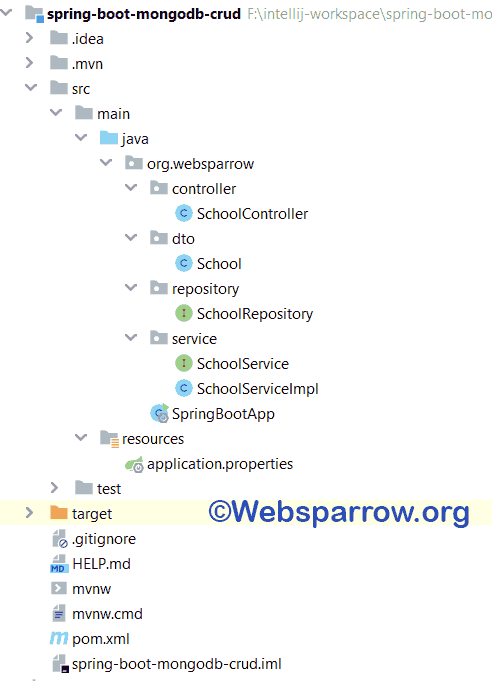
Configuration
The very first step is to configure the database connection string in the application.properties file under the src/main/resources
folder.
#Database connection strings
spring.data.mongodb.uri=mongodb://localhost/springboot_mongodb_crud
Data Model
Create a POJO class School
having the following attributes: id, name, establishmentYear, availableCourses, and strength and generates its Getters and Setters…
package org.websparrow.dto;
import org.springframework.data.annotation.Id;
import org.springframework.data.mongodb.core.mapping.Document;
@Document
public class School {
@Id
private String id;
private String name;
private int establishmentYear;
private String[] availableCourses;
private int strength;
// Generates Getters and Setters...
public School() {}
}
@Document
annotation identifies a domain object to be persisted to MongoDB.
@Id
annotation tells MongoDB to generate a unique Id for every document (demarcates an identifier).
Repository
Create a SchoolRepository
interface which extends MongoRepository
.
package org.websparrow.repository;
import org.springframework.data.mongodb.repository.MongoRepository;
import org.springframework.stereotype.Repository;
import org.websparrow.dto.School;
@Repository
public interface SchoolRepository extends MongoRepository<School, String> {
}
Service
Declare all the methods in the SchoolService
interface to perform the CRUD operation on the school data.
package org.websparrow.service;
import org.springframework.stereotype.Service;
import org.websparrow.dto.School;
import java.util.List;
import java.util.Map;
@Service
public interface SchoolService {
School create(School school);
List<School> read();
School update(School school);
Map<String, String> delete(String id);
}
SchoolServiceImpl
is the implementation class of the SchoolService
interface.
package org.websparrow.service;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import org.websparrow.dto.School;
import org.websparrow.repository.SchoolRepository;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
@Service
public class SchoolServiceImpl implements SchoolService {
@Autowired
private SchoolRepository schoolRepository;
@Override
public School create(School school) {
return schoolRepository.insert(school);
}
@Override
public List<School> read() {
return schoolRepository.findAll();
}
@Override
public School update(School school) {
return schoolRepository.save(school);
}
@Override
public Map<String, String> delete(String id) {
// Total count of data before delete
long beforeDelete = schoolRepository.count();
schoolRepository.deleteById(id);
// Total count of data after delete
long afterDelete = schoolRepository.count();
String messageValue = beforeDelete == afterDelete ? "Something went wrong!" : "Record deleted";
Map<String, String> deleteMap = new HashMap<>();
deleteMap.put("message", messageValue);
return deleteMap;
}
}
Controller
Create SchoolController
class handles the user request to perform create, read, update, and delete operation and response accordingly. Learn more about @RestController and @Autowired annotation.
package org.websparrow.controller;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.MediaType;
import org.springframework.web.bind.annotation.*;
import org.websparrow.dto.School;
import org.websparrow.service.SchoolService;
import java.util.List;
import java.util.Map;
@RestController
@RequestMapping("api/schools")
public class SchoolController {
@Autowired
private SchoolService schoolService;
@PostMapping(consumes = MediaType.APPLICATION_JSON_VALUE, produces = MediaType.APPLICATION_JSON_VALUE)
public School saveSchool(@RequestBody School school) {
return schoolService.create(school);
}
@GetMapping(produces = MediaType.APPLICATION_JSON_VALUE)
public List<School> getAllSchools() {
return schoolService.read();
}
@PutMapping(consumes = MediaType.APPLICATION_JSON_VALUE, produces = MediaType.APPLICATION_JSON_VALUE)
public School updateSchool(@RequestBody School school) {
return schoolService.update(school);
}
@DeleteMapping("/{id}")
public Map<String, String> deleteSchool(@PathVariable String id) {
return schoolService.delete(id);
}
}
Run it
Create an SpringBootApp
class and run it.
package org.websparrow;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class SpringBootApp {
public static void main(String[] args) {
SpringApplication.run(SpringBootApp.class, args);
}
}
Test the application
Now everything is done. Let’s test the application. To test the application open the Postman and follow the steps:
Download Source Code: spring-boot-mongodb-crud-example.zip
References
- Spring Data CrudRepository Interface Example
- Spring Boot RESTful CRUD Example with MySQL Database
- Accessing Data with MongoDB