Spring Boot + Jasper Report Example
This guide shows you Spring Boot + Jasper Report example. Jasper Report is an open source Java reporting tool. It can generate verity of reports like PDF, Excel, etc.
Similar Post: Spring Boot + Jasper Report + MySQL Database Example
What we’ll build
In this example, we will create the list of Employee, design the report, and fill the list of employees into the report. At last, it generates a PDF report.
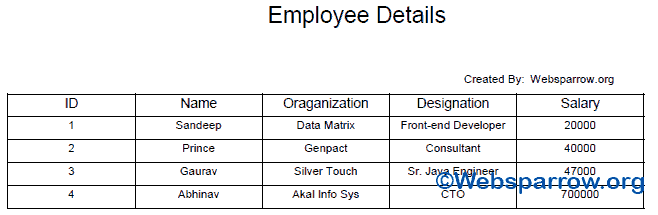
Technology Used
Find the list of technologies used in this example.
- STS 4
- Java 8
- Spring Boot 2.1.4.RELEASE
- Jaspersoft iReport Designer
Dependency Required
Add the following dependencies to your pom.xml.
<dependency>
<groupId>net.sf.jasperreports</groupId>
<artifactId>jasperreports</artifactId>
<version>6.4.0</version>
</dependency>
Steps to follow
To generate the error-free report, we need to follow the below steps:
Step 1: Create a list of employees.
private List<Employee> empList = Arrays.asList(
new Employee(1, "Sandeep", "Data Matrix", "Front-end Developer", 20000),
new Employee(2, "Prince", "Genpact", "Consultant", 40000),
new Employee(3, "Gaurav", "Silver Touch ", "Sr. Java Engineer", 47000),
new Employee(4, "Abhinav", "Akal Info Sys", "CTO", 700000));
Step 2: Compile the report(.jrxml
to .jasper
) that we have designed using
Jaspersoft iReport Designer tool.
JasperReport jasperReport = JasperCompileManager.compileReport(reportPath + "\\employee-rpt.jrxml");
Step 3: Pass the list employees into JRBeanCollectionDataSource
.
JRBeanCollectionDataSource jrBeanCollectionDataSource = new JRBeanCollectionDataSource(empList);
Step 4: Add the parameter (optional).
Map<String, Object> parameters = new HashMap<>();
parameters.put("createdBy", "Websparrow.org");
Step 5: Fill the list of employee and parameters into the report by calling JasperFillManager.fillReport()
;
JasperPrint jasperPrint = JasperFillManager.fillReport(jasperReport, parameters, jrBeanCollectionDataSource);
Step 6: Finally export the report to a PDF file.
JasperExportManager.exportReportToPdfFile(jasperPrint, reportPath + "\\Emp-Rpt.pdf");
Report Templates
Report designs are defined in JRXML files.
<?xml version="1.0" encoding="UTF-8"?>
<jasperReport xmlns="http://jasperreports.sourceforge.net/jasperreports" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://jasperreports.sourceforge.net/jasperreports http://jasperreports.sourceforge.net/xsd/jasperreport.xsd" name="employee-rpt" pageWidth="595" pageHeight="842" columnWidth="555" leftMargin="20" rightMargin="20" topMargin="20" bottomMargin="20">
<property name="ireport.zoom" value="1.0"/>
<property name="ireport.x" value="0"/>
<property name="ireport.y" value="0"/>
<parameter name="createdBy" class="java.lang.String"/>
<field name="id" class="java.lang.Integer"/>
<field name="name" class="java.lang.String"/>
<field name="oraganization" class="java.lang.String"/>
<field name="designation" class="java.lang.String"/>
<field name="salary" class="java.lang.Integer"/>
<background>
<band splitType="Stretch"/>
</background>
<title>
<band height="42" splitType="Stretch">
<staticText>
<reportElement x="64" y="0" width="481" height="42"/>
<textElement textAlignment="Center">
<font size="20" isBold="true"/>
</textElement>
<text><![CDATA[Employee Details]]></text>
</staticText>
</band>
</title>
<columnHeader>
<band height="61" splitType="Stretch">
<staticText>
<reportElement x="0" y="41" width="111" height="20"/>
<box>
<pen lineWidth="0.25"/>
<topPen lineWidth="0.25"/>
<leftPen lineWidth="0.25"/>
<bottomPen lineWidth="0.25"/>
<rightPen lineWidth="0.25"/>
</box>
<textElement textAlignment="Center">
<font size="12" isBold="true" isItalic="false"/>
</textElement>
<text><![CDATA[ID]]></text>
</staticText>
<staticText>
<reportElement x="111" y="41" width="111" height="20"/>
<box>
<pen lineWidth="0.25"/>
<topPen lineWidth="0.25"/>
<leftPen lineWidth="0.25"/>
<bottomPen lineWidth="0.25"/>
<rightPen lineWidth="0.25"/>
</box>
<textElement textAlignment="Center">
<font size="12" isBold="true" isItalic="false"/>
</textElement>
<text><![CDATA[Name]]></text>
</staticText>
<staticText>
<reportElement x="222" y="41" width="111" height="20"/>
<box>
<pen lineWidth="0.25"/>
<topPen lineWidth="0.25"/>
<leftPen lineWidth="0.25"/>
<bottomPen lineWidth="0.25"/>
<rightPen lineWidth="0.25"/>
</box>
<textElement textAlignment="Center">
<font size="12" isBold="true" isItalic="false"/>
</textElement>
<text><![CDATA[Oraganization]]></text>
</staticText>
<staticText>
<reportElement x="333" y="41" width="111" height="20"/>
<box>
<pen lineWidth="0.25"/>
<topPen lineWidth="0.25"/>
<leftPen lineWidth="0.25"/>
<bottomPen lineWidth="0.25"/>
<rightPen lineWidth="0.25"/>
</box>
<textElement textAlignment="Center">
<font size="12" isBold="true" isItalic="false"/>
</textElement>
<text><![CDATA[Designation]]></text>
</staticText>
<staticText>
<reportElement x="444" y="41" width="111" height="20"/>
<box>
<pen lineWidth="0.25"/>
<topPen lineWidth="0.25"/>
<leftPen lineWidth="0.25"/>
<bottomPen lineWidth="0.25"/>
<rightPen lineWidth="0.25"/>
</box>
<textElement textAlignment="Center">
<font size="12" isBold="true" isItalic="false"/>
</textElement>
<text><![CDATA[Salary]]></text>
</staticText>
<textField>
<reportElement x="456" y="21" width="99" height="20"/>
<textElement/>
<textFieldExpression><![CDATA[$P{createdBy}]]></textFieldExpression>
</textField>
<staticText>
<reportElement x="398" y="21" width="58" height="20"/>
<textElement/>
<text><![CDATA[Created By:]]></text>
</staticText>
</band>
</columnHeader>
<detail>
<band height="20" splitType="Stretch">
<textField>
<reportElement x="0" y="0" width="111" height="20"/>
<box>
<pen lineWidth="0.25"/>
<topPen lineWidth="0.25"/>
<leftPen lineWidth="0.25"/>
<bottomPen lineWidth="0.25"/>
<rightPen lineWidth="0.25"/>
</box>
<textElement textAlignment="Center"/>
<textFieldExpression><![CDATA[$F{id}]]></textFieldExpression>
</textField>
<textField>
<reportElement x="111" y="0" width="111" height="20"/>
<box>
<pen lineWidth="0.25"/>
<topPen lineWidth="0.25"/>
<leftPen lineWidth="0.25"/>
<bottomPen lineWidth="0.25"/>
<rightPen lineWidth="0.25"/>
</box>
<textElement textAlignment="Center"/>
<textFieldExpression><![CDATA[$F{name}]]></textFieldExpression>
</textField>
<textField>
<reportElement x="222" y="0" width="111" height="20"/>
<box>
<pen lineWidth="0.25"/>
<topPen lineWidth="0.25"/>
<leftPen lineWidth="0.25"/>
<bottomPen lineWidth="0.25"/>
<rightPen lineWidth="0.25"/>
</box>
<textElement textAlignment="Center"/>
<textFieldExpression><![CDATA[$F{oraganization}]]></textFieldExpression>
</textField>
<textField>
<reportElement x="333" y="0" width="111" height="20"/>
<box>
<pen lineWidth="0.25"/>
<topPen lineWidth="0.25"/>
<leftPen lineWidth="0.25"/>
<bottomPen lineWidth="0.25"/>
<rightPen lineWidth="0.25"/>
</box>
<textElement textAlignment="Center"/>
<textFieldExpression><![CDATA[$F{designation}]]></textFieldExpression>
</textField>
<textField>
<reportElement x="444" y="0" width="111" height="20"/>
<box>
<pen lineWidth="0.25"/>
<topPen lineWidth="0.25"/>
<leftPen lineWidth="0.25"/>
<bottomPen lineWidth="0.25"/>
<rightPen lineWidth="0.25"/>
</box>
<textElement textAlignment="Center"/>
<textFieldExpression><![CDATA[$F{salary}]]></textFieldExpression>
</textField>
</band>
</detail>
</jasperReport>
Model Class
Create a simple Employee
class with its fields. Generate the Getters and Setters method of all fields and parameterized constructor.
package org.websparrow.report.dto;
public class Employee {
// Generate Getters and Setters...
private int id;
private String name;
private String oraganization;
private String designation;
private int salary;
public Employee(int id, String name, String oraganization, String designation, int salary) {
super();
this.id = id;
this.name = name;
this.oraganization = oraganization;
this.designation = designation;
this.salary = salary;
}
}
Service Class
Inside the service class, we follow all the steps mentioned above. The service class is responsible to serve the report.
package org.websparrow.report.service;
import java.util.Arrays;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.springframework.stereotype.Service;
import org.websparrow.report.dto.Employee;
import net.sf.jasperreports.engine.JasperCompileManager;
import net.sf.jasperreports.engine.JasperExportManager;
import net.sf.jasperreports.engine.JasperFillManager;
import net.sf.jasperreports.engine.JasperPrint;
import net.sf.jasperreports.engine.JasperReport;
import net.sf.jasperreports.engine.data.JRBeanCollectionDataSource;
@Service
public class EmployeeReportService {
private List<Employee> empList = Arrays.asList(
new Employee(1, "Sandeep", "Data Matrix", "Front-end Developer", 20000),
new Employee(2, "Prince", "Genpact", "Consultant", 40000),
new Employee(3, "Gaurav", "Silver Touch ", "Sr. Java Engineer", 47000),
new Employee(4, "Abhinav", "Akal Info Sys", "CTO", 700000));
public String generateReport() {
try {
String reportPath = "F:\\Content\\Report";
// Compile the Jasper report from .jrxml to .japser
JasperReport jasperReport = JasperCompileManager.compileReport(reportPath + "\\employee-rpt.jrxml");
// Get your data source
JRBeanCollectionDataSource jrBeanCollectionDataSource = new JRBeanCollectionDataSource(empList);
// Add parameters
Map<String, Object> parameters = new HashMap<>();
parameters.put("createdBy", "Websparrow.org");
// Fill the report
JasperPrint jasperPrint = JasperFillManager.fillReport(jasperReport, parameters,
jrBeanCollectionDataSource);
// Export the report to a PDF file
JasperExportManager.exportReportToPdfFile(jasperPrint, reportPath + "\\Emp-Rpt.pdf");
System.out.println("Done");
return "Report successfully generated @path= " + reportPath;
} catch (Exception e) {
e.printStackTrace();
return e.getMessage();
}
}
}
Execute it
The App
class contains the main method and start the application. It also initialized the EmployeeReportService
class when the server startup and generate the report. For more info check the console log of your IDE.
package org.websparrow.report;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.context.annotation.Bean;
import org.websparrow.report.service.EmployeeReportService;
@SpringBootApplication
public class App {
public static void main(String[] args) {
SpringApplication.run(App.class, args);
}
@Bean
public String generateReport(final EmployeeReportService employeeReportService) {
String msg = employeeReportService.generateReport();
System.out.println(msg);
return msg;
}
}
If the application is web-based, we can call it via controller class as well.
package org.websparrow.report.controller;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import org.websparrow.report.service.EmployeeReportService;
@RestController
@RequestMapping("/employee")
public class EmpReportController {
@Autowired
private EmployeeReportService employeeReportService;
@GetMapping("/report")
public String empReport() {
return employeeReportService.generateReport();
}
}
To get the employee PDF report, hit the http://localhost:8080/employee/report URL on your web browser. Finally, we will get the below report on the specified location.
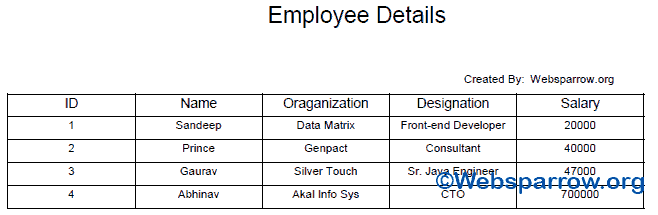
Download Source Code: spring-boot-jasper-report-example.zip