Overview of Java 8 Date and Time API
Java 8 introduced a modern Date and Time API to address the shortcomings of the older java.util.Date
and java.util.Calendar
classes. In this blog, we’ll explore the Java 8 Date and Time API, its features, benefits, and advantages.
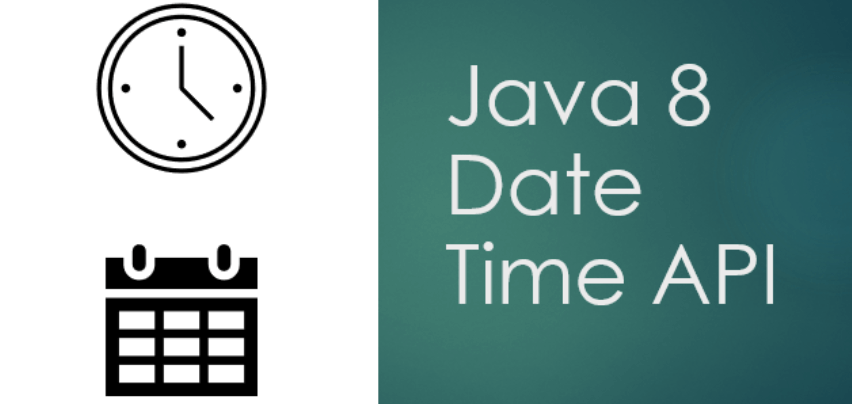
1. Overview
The new Date and Time API brings clarity and flexibility to date and time handling in Java. It introduces immutability, thread safety, better domain modeling, and comprehensive formatting options. The API is part of the java.time
package and introduces key classes like LocalDate
, LocalTime
, LocalDateTime
, ZonedDateTime
, Period
, and Duration
.
1.1 LocalDate
LocalDate
represents a date (year, month, and day) without time or time zone information.
package org.websparrow.dateandtime;
import java.time.LocalDate;
public class LocalDateExample {
public static void main(String[] args) {
LocalDate today = LocalDate.now();
System.out.println("Today's Date: " + today);
}
}
Output:
Today's Date: 2023-08-19
1.1.1 LocalDate with Arithmetic Operation
package org.websparrow.dateandtime;
import java.time.LocalDate;
import java.time.Period;
public class DateArithmetic {
public static void main(String[] args) {
LocalDate today = LocalDate.now();
LocalDate futureDate = today.plus(Period.ofMonths(3));
System.out.println("Future Date: " + futureDate);
}
}
Output:
Future Date: 2023-11-19
1.2 LocalTime
LocalTime
represents a time without a date or time zone.
package org.websparrow.dateandtime;
import java.time.LocalTime;
public class LocalTimeExample {
public static void main(String[] args) {
LocalTime currentTime = LocalTime.now();
System.out.println("Current Time: " + currentTime);
}
}
Output: (Note: The exact time value will vary depending on when you run the code.)
Current Time: 20:30:45.123456789
1.3 LocalDateTime
LocalDateTime
combines date and time, without time zone information.
package org.websparrow.dateandtime;
import java.time.LocalDateTime;
public class LocalDateTimeExample {
public static void main(String[] args) {
LocalDateTime currentDateTime = LocalDateTime.now();
System.out.println("Current Date and Time: " + currentDateTime);
}
}
Output:
Current Date and Time: 2023-08-19T20:40:45.123456789
1.4 ZonedDateTime
ZonedDateTime
represents a date and time with a specific time zone.
package org.websparrow.dateandtime;
import java.time.ZonedDateTime;
import java.time.ZoneId;
public class ZonedDateTimeExample {
public static void main(String[] args) {
ZonedDateTime zonedDateTime = ZonedDateTime.now(ZoneId.of("America/New_York"));
System.out.println("Current Date and Time in New York: " + zonedDateTime);
}
}
Output:
Current Date and Time in New York: 2023-08-19T11:30:45.123456789-04:00[America/New_York]
1.5 Period
Period
represents a date-based amount of time, such as days, months, and years.
package org.websparrow.dateandtime;
import java.time.LocalDate;
import java.time.Period;
public class PeriodExample {
public static void main(String[] args) {
LocalDate startDate = LocalDate.of(2023, 1, 1);
LocalDate endDate = LocalDate.of(2023, 8, 19);
Period period = Period.between(startDate, endDate);
System.out.println("Period between start and end dates: " + period);
}
}
Output:
Period between start and end dates: P0Y7M18D
The output Period between start and end dates: P0Y7M18D is in the ISO-8601 format for representing durations or periods. Let’s break down what each part of this format means:
P
: Indicates the start of a period or duration.0Y
: Zero years. This means there are no years in the period.7M
: Seven months. This indicates that the period includes seven months.18D
: Eighteen days. This indicates that the period also includes eighteen days.
So, the period between the start and end dates in the example is 7 months and 18 days, with no years included in the duration.
1.6 Duration
Duration
represents a time-based amount of time, such as hours, minutes, and seconds.
package org.websparrow.dateandtime;
import java.time.LocalTime;
import java.time.Duration;
public class DurationExample {
public static void main(String[] args) {
LocalTime startTime = LocalTime.of(9, 0);
LocalTime endTime = LocalTime.of(11, 30);
Duration duration = Duration.between(startTime, endTime);
System.out.println("Duration between start and end times: " + duration);
}
}
Output:
Duration between start and end times: PT2H30M
The output Duration between start and end times: PT2H30M, the output represents a duration of 2 hours and 30 minutes. Let’s break down the parts of this ISO-8601 duration format:
PT
: Indicates the start of a time duration.2H
: Two hours. This indicates that the duration includes two hours.30M
: Thirty minutes. This indicates that the duration also includes thirty minutes.
So, the duration between the start and end times in the example is 2 hours and 30 minutes.
2. Key Features of Date/Time API
The new API is driven by below core ideas:
2.1 Immutability and Thread Safety:
Unlike the mutable Date
and Calendar
classes, Java 8’s API introduces immutable classes, ensuring safer concurrent programming.
2.2 Comprehensive Formatting:
The DateTimeFormatter
class provides customizable date and time formatting, making it easy to display dates in various formats.
2.3 Time Zone Support:
The ZoneId
and ZonedDateTime
classes allow handling time zones effectively, making cross-timezone applications much simpler.
2.4 Fluent API Design:
The API uses a fluent interface design, improving code readability and expressiveness.
2.5 Enhanced Domain Modeling:
New classes like Period
and Duration
offer better representation of date-based and time-based amounts, simplifying calculations.
3. Summary
Java 8’s Date and Time API represents a leap forward in handling date and time in Java programming. Its advancements over the old classes ensure better clarity, accuracy, and ease of use. Java 8 new Date and Time API enhance your code’s reliability and maintainability, making Java programming a smoother experience.
References
- Java 8 – Difference between two LocalDate
- Java 8– Calculate date & time difference between two Zone
- Java 8 – Calculate difference between two LocalDateTime
- Package java.time – JavaDoc