Java – Capitalize the first letter of a String
In this short tutorial, you will get an idea of how to capitalize the first letter of a string. In Java, it can be possible in many ways like using core Java programming or using any third-party library e.g. Apache Commons Lang 3.
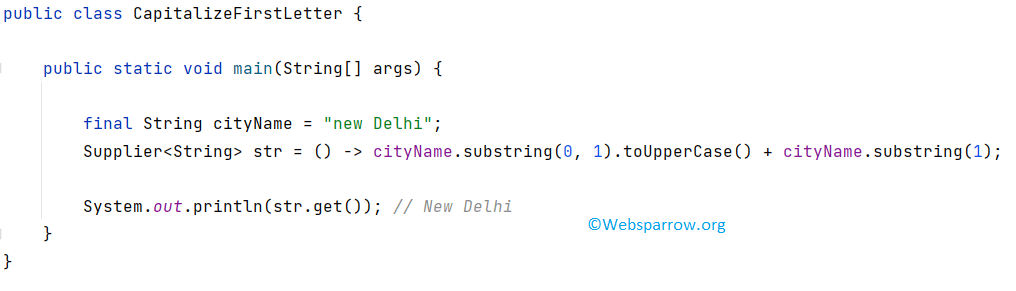
Similar Post: Capitalize the first letter of each word in a String using Java
1. Java 8 Lambda Expression
Java 8 introduced the Supplier<T>
functional interface to implement the lambda expression in Java.
final String cityName = "new Delhi";
Supplier<String> str = () -> cityName.substring(0, 1).toUpperCase() + cityName.substring(1);
System.out.println(str.get()); // New Delhi
2. Core Java Programming
With Core Java programming, it will just increase the number of lines in your codebase, and the logic will remain the same as Java 8 Lambda Expression.
package org.websparrow;
import java.util.function.Supplier;
public class CapitalizeFirstLetter {
public static void main(String[] args) {
final String cityName1 = capitalizeFirstLetter("varanasi");
System.out.println(cityName1); // Varanasi
final String cityName2 = capitalizeFirstLetter("VARANASI");
System.out.println(cityName2); // VARANASI
final String cityName3 = capitalizeFirstLetter("$varanasi");
System.out.println(cityName3); // $varanasi
}
public static String capitalizeFirstLetter(final String str) {
if (null == str || str.trim().equals("")) {
throw new RuntimeException("String is not valid");
}
return str.substring(0, 1).toUpperCase().concat(str.substring(1));
}
}
Output:
Varanasi
VARANASI
$varanasi
3. Apache Commons Lang 3
With Apache Commons Lang 3 library, you can use its StringUtils.capitalize(String str)
API to capitalize the first letter of a string. You can grab the Apache commons-lang3
by adding the below dependency to your pom.xml file.
<dependencies>
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.12.0</version>
</dependency>
</dependencies>
// Apache Commons Lang 3, StringUtils
final String name1 = StringUtils.capitalize("atul");
System.out.println(name1); // Atul
final String name2 = StringUtils.capitalize("atul rai");
System.out.println(name2); // Atul rai
final String name3 = StringUtils.capitalize("ATUL RAI");
System.out.println(name3); // ATUL RAI
final String name4 = StringUtils.capitalize("@atul rai");
System.out.println(name4); // @atul rai
In Apache
commons-lang3
library, there is anotherWordUtils.capitalize(String str)
API that capitalizes the first letter of each word of a string, but it is deprecated from the 3.6.0 version.// Apache Commons Lang 3, WordUtils final String foo = WordUtils.capitalize("cow"); System.out.println(foo); // Cow final String boo = WordUtils.capitalize("i'm a holy cow."); System.out.println(boo); // I'm A Holy Cow.
References
- Interface Supplier<T>- Java 8
- StringUtils- Apache Commons Lang 3
- WordUtils- Apache Commons Lang 3
- How to capitalize the first letter of a String in PHP