Difference between @PreAuthorize and @PostAuthorize Annotations in Spring Security
Spring Security is a powerful framework that provides robust authentication and authorization features for Java applications. Among its many capabilities, it offers the ability to control method-level access to secure your application. Two commonly used annotations for this purpose are @PreAuthorize
and @PostAuthorize
. In this blog, we will explore the key differences between these two annotations and how they can be used effectively in your Spring Security configuration.
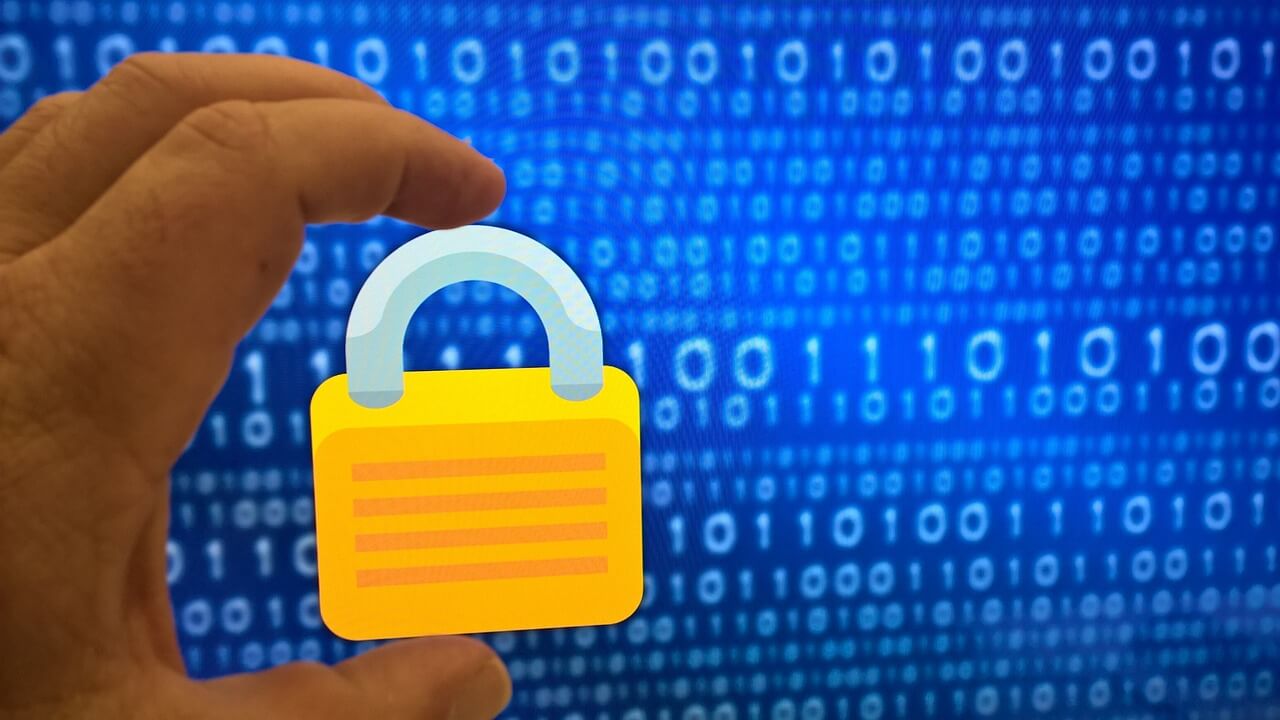
1. @PreAuthorize
The @PreAuthorize
annotation is used to specify access control before a method is executed. It allows you to define access restrictions based on a condition that is evaluated before the method body is executed. Here are some important points to understand about @PreAuthorize
:
- Pre-Execution Condition: The condition specified in
@PreAuthorize
is evaluated before the method execution. If the condition is not satisfied, the method will not be executed. - Method Parameter Access: You can reference method parameters and other Spring Security expressions within the condition to determine whether access should be granted. For example, you can check if the current user has a specific role or permission to access a particular resource.
- Usage:
@PreAuthorize
is typically used for guarding methods that perform sensitive or critical operations. It acts as a gatekeeper to ensure that only authorized users can execute these methods.
Example:
@PreAuthorize("hasRole('ROLE_ADMIN')")
public void deleteUser(User user) {
// Delete user logic
}
In this example, the deleteUser
method can only be executed by users with the ‘ROLE_ADMIN’ authority.
2. @PostAuthorize
The @PostAuthorize
annotation, on the other hand, is used to perform access control after a method has executed. It allows you to filter the results of a method based on a condition. Here’s what you should know about @PostAuthorize
:
- Post-Execution Filtering: The condition specified in
@PostAuthorize
is evaluated after the method has been executed. It allows you to filter or modify the method’s return value based on the condition. - Method Return Value Access: You can reference the return value of the method and other Spring Security expressions in the condition to determine if the result should be filtered.
- Usage:
@PostAuthorize
is commonly used when you want to filter the results of a method to ensure that only authorized data is returned to the user. For instance, you can use it to filter a list of records to show only those accessible by the current user.
Example:
@PostAuthorize("returnObject.owner == authentication.name")
public Record getRecord(int recordId) {
// Retrieve and return the record
}
In this example, the getRecord
method will return the record only if the owner of the record matches the currently authenticated user.
3. Summary
In summary, @PreAuthorize
and @PostAuthorize
are powerful tools in Spring Security for controlling method-level access. The key difference lies in when they evaluate access control conditions: @PreAuthorize
checks before the method execution, while @PostAuthorize
filters the results after the method has executed.
4. References
- Method Security- Spring Doc
- Spring Boot + Spring Security Authentication with LDAP
- Spring Security Role Based Authorization Example