iText API- Protect PDF Document with Password in Java
In this Java tutorial, we are going to show how you will protect your pdf document with a password using iText API. PdfWriter.setEncryption()
method is used to encrypt the PDF document while creating it. We need to pass the arguments in the method while creating new documents.
setEncryption
public void setEncryption(byte[] userPassword, byte[] ownerPassword, int permissions, int encryptionType)
Sets the encryption options for this document. The userPassword and the ownerPassword can be null or have zero length. In this case, the ownerPassword is replaced by a random string. The open permissions for the document can be AllowPrinting, AllowModifyContents, AllowCopy, AllowModifyAnnotations, AllowFillIn, AllowScreenReaders, AllowAssembly and AllowDegradedPrinting.
Parameters:
userPassword – the user password. Can be null or empty.
ownerPassword – the owner password. Can be null or empty.
permissions – the user permissions.
encryptionType – can be any of the following:
- PdfWriter#STANDARD_ENCRYPTION_40
- PdfWriter#STANDARD_ENCRYPTION_128
- PdfWriter#ENCRYPTION_AES_128
- PdfWriter#ENCRYPTION_AES_256
Throws:
DocumentException
– if the document is already open
JAR Dependencies
To resolve the JAR dependencies, add following in your pom.xml
<dependency>
<groupId>com.itextpdf</groupId>
<artifactId>itextpdf</artifactId>
<version>5.5.10</version>
</dependency>
<dependency>
<groupId>org.bouncycastle</groupId>
<artifactId>bcprov-jdk15on</artifactId>
<version>1.51</version>
</dependency>
You can provide multiple permissions by ORing different values. For example
PdfWriter.ALLOW_PRINTING | PdfWriter.ALLOW_COPY | PdfWriter.ALLOW_SCREENREADERS
Check the Full Example
package org.websparrow.itext;
import java.io.FileOutputStream;
import com.itextpdf.text.Document;
import com.itextpdf.text.PageSize;
import com.itextpdf.text.Paragraph;
import com.itextpdf.text.pdf.PdfWriter;
public class ProtectPdfDocument {
private static String USER_PASS = "RAM123";
private static String OWNER_PASS = "GOD999";
public static void main(String[] args) {
try {
Document document = new Document(PageSize.A4, 20, 20, 20, 20);
PdfWriter pdfWriter = PdfWriter.getInstance(document, new FileOutputStream("iText/protected-doc.pdf"));
pdfWriter.setEncryption(USER_PASS.getBytes(), OWNER_PASS.getBytes(), PdfWriter.ALLOW_COPY,
PdfWriter.ENCRYPTION_AES_128);
document.open();
document.add(new Paragraph("Welcome to WebSparrow.org"));
document.add(new Paragraph("Don't worry, your pdf document is protected with password."));
document.close();
System.out.println("PDf protected with password.");
} catch (Exception e) {
e.printStackTrace();
}
}
}
Output:
Now your generated PDF is password protected. Use the RAM123 to open the document.
Screen 1
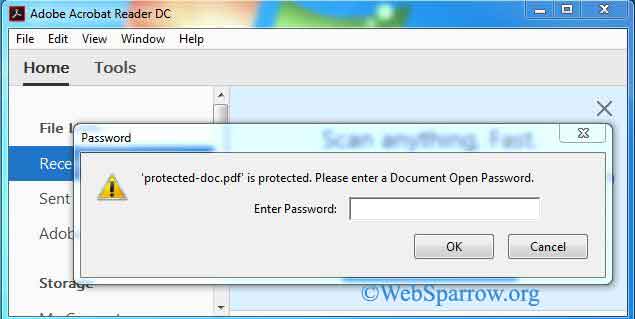
Screen 2
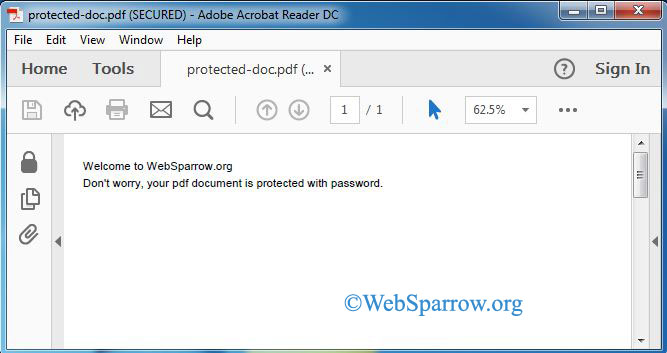