Difference between ArrayList and Vector in Java
ArrayList
and Vector
are two commonly used classes in Java that provide dynamic arrays. They are similar in many ways but have some fundamental differences that developers should be aware of when choosing between them. In this article, we will explore the dissimilarities between ArrayList
and Vector
.
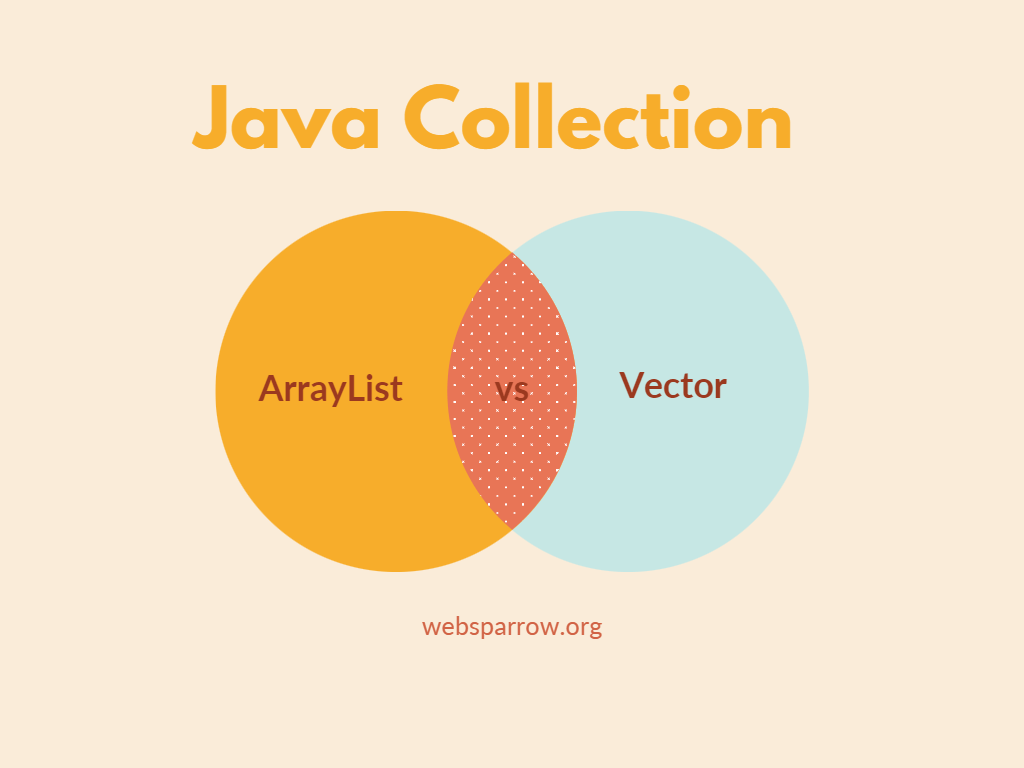
1. Synchronization
One of the most significant differences between ArrayList
and Vector
is their synchronization behavior. Vector
is synchronized by default, meaning that all its methods are thread-safe. This synchronization ensures that multiple threads cannot modify the Vector
simultaneously, preventing potential data corruption and maintaining data integrity.
On the other hand, ArrayList
is not synchronized, and if multiple threads access and modify an ArrayList
concurrently, you need to provide external synchronization to avoid data inconsistencies.
ArrayList<Integer> arrayList = new ArrayList<>();
Vector<Integer> vector = new Vector<>();
// Thread 1
arrayList.add(1);
vector.add(1);
// Thread 2
arrayList.add(2);
vector.add(2);
In this example, if multiple threads simultaneously add elements to the ArrayList
, it may lead to data inconsistencies. However, the Vector
implementation ensures that thread synchronization is maintained, preventing data corruption.
2. Performance
Due to the synchronized nature of Vector
, it incurs additional overhead in terms of performance compared to ArrayList
. The synchronization mechanisms in Vector
ensure thread safety but introduce extra locks and checks, impacting the overall execution speed.
ArrayList
, being unsynchronized, offers better performance in single-threaded environments. If you are working in a single-threaded scenario or can provide external synchronization, ArrayList
is a more efficient choice.
ArrayList<Integer> arrayList = new ArrayList<>();
Vector<Integer> vector = new Vector<>();
// Adding elements to ArrayList
long arrayListStartTime = System.nanoTime();
for (int i = 0; i < 1000000; i++) {
arrayList.add(i);
}
long arrayListEndTime = System.nanoTime();
long arrayListExecutionTime = arrayListEndTime - arrayListStartTime;
// Adding elements to Vector
long vectorStartTime = System.nanoTime();
for (int i = 0; i < 1000000; i++) {
vector.add(i);
}
long vectorEndTime = System.nanoTime();
long vectorExecutionTime = vectorEndTime - vectorStartTime;
System.out.println("ArrayList Execution Time: " + arrayListExecutionTime);
System.out.println("Vector Execution Time: " + vectorExecutionTime);
In the above code, you will notice that ArrayList
performs faster compared to Vector
because ArrayList
does not have the synchronization overhead.
Post you may like: How to iterate ArrayList in Java
3. Usage Scenarios
Given their different characteristics, ArrayList
and Vector
are suitable for different scenarios. Vector
is typically preferred in multi-threaded environments where thread safety is a concern. It can be useful when you need to share the collection among multiple threads without worrying about external synchronization. ArrayList
, on the other hand, is ideal for single-threaded scenarios where performance is a priority and you can handle synchronization manually if required.
4. Growth Rate
Another distinguishing factor between ArrayList
and Vector
is their growth rate strategy. When an ArrayList
or Vector
exceeds its current capacity while adding elements, it needs to increase its size to accommodate additional elements. ArrayList
increases its size by a fixed percentage (typically 50% of the current capacity) using the grow()
method.
In contrast, Vector
doubles its size using the grow()
method when it needs to expand its capacity. Doubling the size allows Vector
to reduce the frequency of reallocation, resulting in better performance when a large number of elements are added.
ArrayList<Integer> arrayList = new ArrayList<>();
Vector<Integer> vector = new Vector<>();
// Adding elements to ArrayList
for (int i = 0; i < 10; i++) {
arrayList.add(i);
System.out.println("ArrayList Capacity: " + arrayList.size());
}
// Adding elements to Vector
for (int i = 0; i < 10; i++) {
vector.add(i);
System.out.println("Vector Capacity: " + vector.size());
}
In this example, you will observe that the ArrayList
capacity increases by approximately 50% with each expansion, while the Vector
capacity doubles.
5. Legacy Status
The Vector
class is part of Java’s legacy collection framework introduced in JDK 1.0, while ArrayList
was introduced in JDK 1.2 as part of the more modern and efficient collections framework. Although Vector
is still supported for backward compatibility, ArrayList
is generally recommended for new code due to its improved performance and flexibility.
6. Usage Scenarios
Given their different characteristics, ArrayList
and Vector
are suitable for different scenarios. Vector
is typically preferred in multi-threaded environments where thread safety is a concern. It can be useful when you need to share the collection among multiple threads without worrying about external synchronization. ArrayList
, on the other hand, is ideal for single-threaded scenarios where performance is a priority and you can handle synchronization manually if required.
7. Conclusion
Since, ArrayList
and Vector
share similar functionalities, their synchronization behavior, performance, growth rate, and legacy status set them apart. If you need thread safety and can tolerate some performance overhead, Vector
is a good choice.
However, if you require better performance and can handle synchronization externally, ArrayList
is the recommended option. Consider your specific requirements and choose the appropriate class accordingly to ensure optimal performance and maintainable code.