Java Just-In-Time (JIT) Compiler Overview
Java, a widely-used programming language, is renowned for its portability and ease of development. A crucial aspect behind its popularity is the Java Virtual Machine (JVM), which enables the execution of Java programs on different platforms. At the heart of JVM lies the Just-In-Time (JIT) compiler, a powerful component responsible for dynamically optimizing and enhancing the performance of Java applications.
What is Just-In-Time (JIT) Compilation?
The JIT compiler is a dynamic execution mechanism that converts Java bytecode, an intermediate representation of Java programs, into native machine code at runtime. Unlike ahead-of-time compilation, where all the code is compiled before execution, JIT compilation takes place on-demand during program execution. It selectively identifies sections of the code, known as hot spots, which are frequently executed, and optimizes them for improved performance.
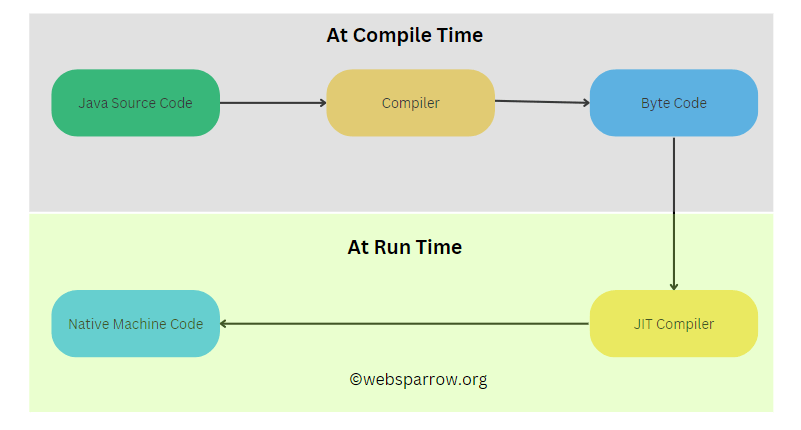
Example: Fibonacci Sequence Calculation
To better understand the role of the JIT compiler, let’s consider a simple example of calculating the Fibonacci sequence. Using a recursive function, we’ll create a Java program that computes the nth Fibonacci number.
package org.websparrow;
public class Fibonacci {
public static int fibonacci(int n) {
if (n <= 1) {
return n;
}
return fibonacci(n - 1) + fibonacci(n - 2);
}
public static void main(String[] args) {
int n = 10;
int result = fibonacci(n);
System.out.println("The " + n + "th Fibonacci number is: " + result);
}
}
When we run this program, the JVM initially interprets the bytecode sequentially, executing the recursive calls for each Fibonacci number. However, as the computation progresses, the JIT compiler comes into play.
JIT Compilation in Action: As the JVM executes the Fibonacci program, the JIT compiler identifies the recursive function as a hot spot due to its repetitive invocation. To optimize its performance, the JIT compiler dynamically compiles the frequently accessed portion of the code, i.e., the fibonacci
method.
During the first invocation, the JIT compiler may choose to interpret the method. However, on subsequent invocations, it dynamically compiles the method into highly efficient native machine code, tailored to the specific hardware and runtime environment. This native code replaces the interpreted bytecode, resulting in significantly faster execution.
The Impact of JIT Compilation: With the JIT compiler’s optimizations, the execution of the Fibonacci program becomes noticeably faster after the initial compilation. As a result, the performance gains achieved by the JIT compilation mechanism can be substantial, especially for computationally intensive tasks and long-running applications.
Advantages of JIT Compilation
- Improved Performance: By selectively compiling hot spots into native machine code, JIT compilation allows Java programs to approach the performance of natively compiled languages.
- Adaptive Optimization: The JIT compiler adapts to the program’s behavior and dynamically recompiles code segments if required, accommodating changes in execution patterns and ensuring optimal performance.
- Platform Independence: JIT compilation allows developers to write Java code once and execute it on different platforms without worrying about low-level hardware details.
Summary
The Java Just-In-Time (JIT) compiler plays a vital role in optimizing the performance of Java applications. By dynamically compiling frequently executed sections of code into native machine code, the JIT compiler bridges the gap between platform independence and execution speed. This results in significant performance improvements, allowing Java programs to rival the performance of natively compiled languages. Understanding the JIT compilation process empowers developers to write efficient and high-performance Java applications.