Java final keyword: final variable, method and class example
In this Java tutorial, we will learn about the final
keyword uses. In the Java programming language, the final
keyword is used in several different contexts to define an entity that can only be assigned once. For example…
- final variables
- final methods and
- final classes
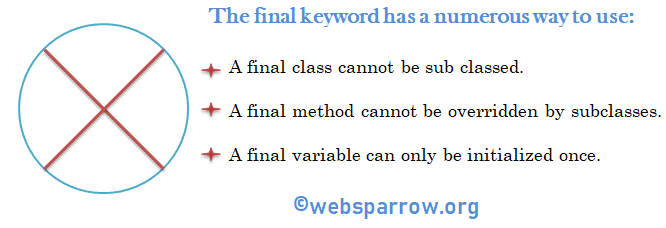
Final Variable
Whenever you have declared any variable as final
and initialized its value then you cannot modify its values. It will become constant.
In this example, we have declared a variable as final
and initialized its value. So whenever we try to reassign the variable value it will throw compile time error.
package org.websparrow;
public class FinalVariable {
public static void main(String[] args) {
// variable declared as final and assigned its value
final String website = "websparrow.org";
try {
// try to reassign the value
website = "www.websparrow.org";
System.out.println("Name of webiste is: " + website);
} catch (Exception e) {
e.printStackTrace();
}
}
}
Exception in thread "main" java.lang.Error: Unresolved compilation problem:
The final local variable website cannot be assigned. It must be blank and not using a compound assignment
at org.websparrow.FinalVariable.main(FinalVariable.java:11)
Similarly, we have declared another variable as final but not assigned its value. It will compile with no error. But once the variable value will be assigned then it cannot be reassigned.
package org.websparrow;
public class FinalVariable2 {
public static void main(String[] args) {
// variable declared as final but not assigned its value
final String website;
try {
// assigned its value but further we cannot reassign its value again
website = "websparrow.org";
System.out.println("Name of webiste is: " + website);
} catch (Exception e) {
e.printStackTrace();
}
}
}
Name of webiste is: websparrow.org
Final Method
Similarly, when a method declared as final
, it cannot be overrides. It will also throw compile time error. Check this example.
package org.websparrow;
class TestClassA {
// method declared as final
public final void sayHello() {
System.out.println("Hello World");
}
}
public class FinalMethod extends TestClassA {
// try to override
public void sayHello() {
System.out.println("Hello Java");
}
public static void main(String[] args) throws Exception {
FinalMethod fm = new FinalMethod();
fm.sayHello();
}
}
Cannot override the final method from TestClassA.
Final Class
And now whenever you declared a class as final
, it cannot be subclassed. In simple words, final classes cannot be extended by other class. It will also throw compile time error.
package org.websparrow;
// class declared as final
final class TestClassB {
public void welcome() {
System.out.println("Welcome");
}
}
// try to extend the final class
public class FinalClass extends TestClassB {
public static void main(String[] args) {
FinalClass fc = new FinalClass();
fc.welcome();
}
}
The type FinalClass cannot subclass the final class TestClassB