Securing Passwords with Spring Security Password Encoder
One of the critical aspects of application security is properly handling user passwords. Storing passwords in plain text or using weak hashing algorithms can expose sensitive user information to potential attacks. To address this concern, Spring Security provides a Password Encoder mechanism that securely encodes and verifies passwords.
In this blog, we’ll explore how to use Spring Security’s Password Encoder to protect user passwords in a Spring application. We’ll cover the following topics:
- Introduction to Password Encoding
- Spring Security’s Password Encoder
- Example Application
- Conclusion
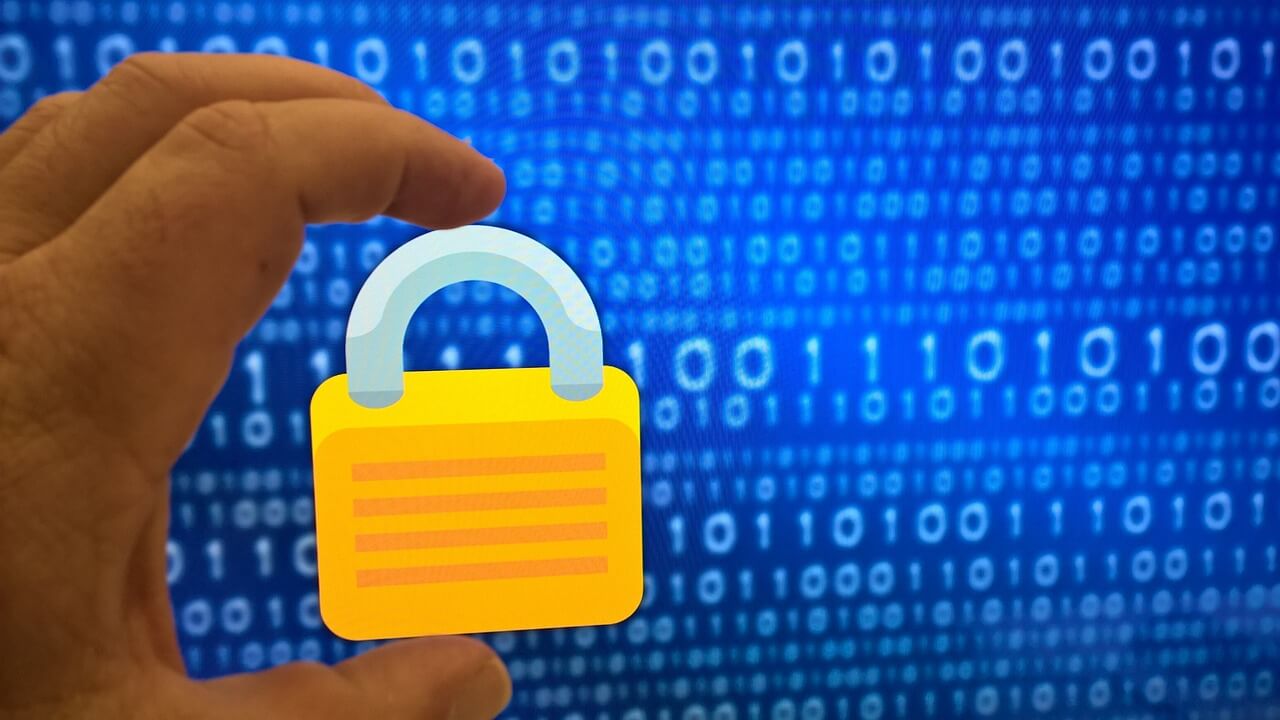
1. Introduction to Password Encoding
Password encoding is a process of transforming plain-text passwords into a secure and irreversible format. The primary purpose of encoding is to protect user passwords even if the stored data is compromised. When a user registers or changes their password, the application encodes the password using a hashing algorithm and stores the encoded value in the database.
During authentication, the entered password is encoded using the same algorithm, and the encoded values are compared. If they match, the password is considered valid, and the user is granted access.
The key characteristics of a good password encoding mechanism are:
- Irreversibility: The encoded password should not be reversible to its original plain-text form. This prevents unauthorized individuals from obtaining the actual password even if they gain access to the stored data.
- Unique Encodings: Encoding the same password multiple times should result in different encoded values. This adds an extra layer of security by preventing the identification of common passwords or patterns.
- Salted Encoding: Salting involves adding random data (a salt) to the password before encoding it. This ensures that even if two users have the same password, their encoded values will be different, making it harder for attackers to identify common passwords.
Related Posts:
2. Spring Security’s Password Encoder
Spring Security provides a comprehensive set of features for securing Spring applications, including built-in support for password encoding. The PasswordEncoder
interface, along with various implementations, allows you to easily encode and verify passwords.
2.1 Common Password Encoder Implementations
Spring Security offers several password encoder implementations, each using a different hashing algorithm. Some of the commonly used implementations are:
- BCryptPasswordEncoder: Uses the bcrypt algorithm, which is a popular choice due to its scalability and resistance to brute-force attacks.
- SCryptPasswordEncoder: Uses the scrypt algorithm, which is known for its memory-hardness properties and increased resistance against hardware attacks.
- StandardPasswordEncoder: Uses a simple hashing algorithm based on the
java.security.MessageDigest
class. Note that this implementation is considered less secure than bcrypt or scrypt. - NoOpPasswordEncoder: A no-op implementation that does not perform any encoding. This is primarily used for testing or when you have encoded passwords from an external system.
It’s crucial to choose a strong password encoder implementation based on your security requirements and the hashing algorithm’s strength.
Post you may like: Types of Password Encoders in Spring Security
2.2 Using Password Encoder in Spring Security
To use a password encoder in your Spring application, you need to define a PasswordEncoder
bean in your application context. Here’s an example configuration class:
package org.websparrow;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder;
import org.springframework.security.crypto.password.PasswordEncoder;
@Configuration
public class SecurityConfig {
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
// Other security-related beans or configurations...
}
In the example above, we define a PasswordEncoder
bean using the BCryptPasswordEncoder
implementation. You can replace it with any other implementation based on your requirements.
Once the PasswordEncoder
bean is defined, you can use it in your application’s services or components to encode and verify passwords.
Let’s take an example of a user registration process where we encode the user’s password before storing it in the database. Here’s a simplified version of a UserService
class:
package org.websparrow;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.security.crypto.password.PasswordEncoder;
public class UserService {
@Autowired
private PasswordEncoder passwordEncoder;
public void registerUser(String username, String password) {
// Encode the password
String encodedPassword = passwordEncoder.encode(password);
// Save the username and encoded password to the database or any other storage mechanism
// ...
}
// Other user-related methods...
}
In the registerUser
method, we receive the username and plain-text password as parameters. The PasswordEncoder
bean is autowired into the service, allowing us to use it for encoding the password. We call the encode
method, passing the plain-text password, and obtain the encoded password.
After encoding, you can store the username and encoded password in your preferred storage mechanism, such as a database.
Now, let’s see an example of how to verify a password during authentication. Here’s a simplified version of an AuthenticationService
class:
package org.websparrow;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.security.crypto.password.PasswordEncoder;
public class AuthenticationService {
@Autowired
private PasswordEncoder passwordEncoder;
public boolean authenticate(String username, String password) {
// Retrieve the encoded password from the database or any other storage mechanism
String encodedPassword = // Retrieve the encoded password using the username
// Verify the entered password against the stored encoded password
return passwordEncoder.matches(password, encodedPassword);
}
// Other authentication-related methods...
}
In the authenticate
method, we retrieve the stored encoded password from the database or any other storage mechanism using the username. Then, we use the matches
method of the PasswordEncoder
to compare the entered password (plain text) with the stored encoded password. If the passwords match, the method returns true
, indicating successful authentication.
By utilizing the PasswordEncoder
provided by Spring Security, you can ensure that user passwords are securely encoded and verified within your application.
3. Example Application
To demonstrate the usage of Spring Security’s Password Encoder, let’s consider a simple web application for user registration and authentication.
3.1 Prerequisites
Make sure you have the following dependencies in your project’s pom.xml
file:
<dependencies>
...
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
...
</dependencies>
3.2 Application Structure
- Create a Spring Boot application with the necessary dependencies and configurations.
- Define the
SecurityConfig
configuration class, as shown earlier, to set up thePasswordEncoder
bean. - Create a
UserService
class to handle user registration and storage. - Implement an
AuthenticationService
class for user authentication.
3.3 Usage
3.3.1 User Registration:
@Autowired
private UserService userService;
@PostMapping("/register")
public String registerUser(@RequestParam("username") String username,
@RequestParam("password") String password) {
userService.registerUser(username, password);
return "Registration successful!";
}
3.3.2 User Authentication:
@Autowired
private AuthenticationService authenticationService;
@PostMapping("/login")
public String login(@RequestParam("username") String username,
@RequestParam("password") String password) {
if (authenticationService.authenticate(username, password)) {
return "Login successful!";
} else {
return "Invalid credentials!";
}
}
3.3.3 Secure Password Storage:
When saving user passwords in a database, storing them securely is essential. Here are some considerations to ensure secure password storage:
- Always use a secure and up-to-date hashing algorithm like
bcrypt
orscrypt
. - Generate a unique salt for each user and include it during password encoding. This adds an extra layer of security by preventing the identification of common passwords or patterns.
- Consider adding additional security measures like password policies (minimum length, complexity requirements) and enforcing password expiration and reset processes.
3.3.4 Password Strength and User Education:
While password encoding provides a layer of security, educating users about creating strong passwords is crucial. Encourage users to choose passwords that are long, complex, and unique. Additionally, consider implementing password strength checkers to guide users in creating strong passwords.
4. Conclusion
Securing user passwords is a critical aspect of application security. Spring Security provides a convenient way to encode and verify passwords using its Password Encoder mechanism. By choosing a strong password encoder implementation, properly storing passwords, and educating users about password strength, you can enhance the security of your application and protect user information.
Remember to implement additional security measures like secure session management, protection against common attacks (such as brute force and SQL injection), and regular security audits to ensure the overall security of your application.
By prioritizing password security and leveraging Spring Security’s features, you can significantly strengthen the authentication process and safeguard user accounts in your Spring applications.
5. References
- Spring Security Doc
- Password Encoder – Spring Security
- Spring Boot Security- How to change default login page
- Spring Boot + Spring Security Authentication with LDAP