Java 8 Stream API allMatch(), anyMatch() and noneMatch() method Example
On this page, we will learn about Java 8 Stream API allMatch()
, anyMatch()
and noneMatch()
method with an example. allMatch()
, anyMatch()
and noneMatch()
are the Terminal Operations methods of Stream API. Terminal operations return a non-stream (cannot be chained) result like primitive or object or collection, or no value at all.
All three method’s return type is boolean
and all three take predicates
as an argument. The method signature of methods are as follows:
boolean allMatch(Predicate<? super T> predicate);
boolean anyMatch(Predicate<? super T> predicate);
boolean noneMatch(Predicate<? super T> predicate);
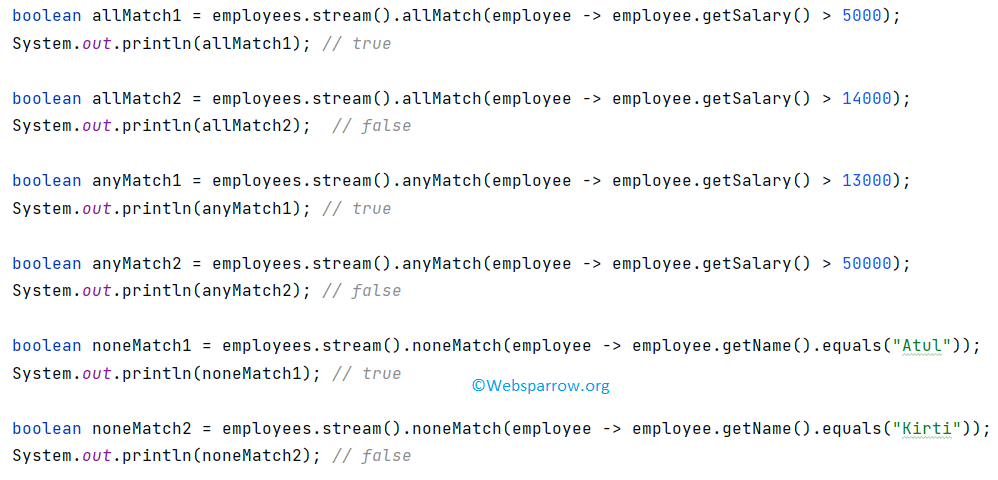
Let’s suppose, we have a list of employees and we want to check the following conditions:
List<Employee> employees = List.of(
new Employee(101, "Manish", 35000, "IT"),
new Employee(12, "Priyanka", 12000, "SALES"),
new Employee(67, "Kirti", 10000, "HR"),
new Employee(55, "Sagar", 25000, "SALES"),
new Employee(87, "Shilpa", 25000, "IT")
);
Condition 1: Return true
if all employee’s salary is greater than 5000
.
Condition 2: Return true
if any employee’s salary is greater than 13000
.
Condition 3: Return true
if any employee’s name is not equal to "Atul"
(the list doesn’t contain any employee whose name is "Atul"
).
1. allMatch()
To satisfy Condition 1, allMatch()
method is the best option. It will iterate all the lists of employees to check the value of the given predicate. Returns true
if the condition is satisfied otherwise return false
.
boolean allMatch1 = employees.stream()
.allMatch(employee -> employee.getSalary() > 5000);
System.out.println(allMatch1); // true
boolean allMatch2 = employees.stream()
.allMatch(employee -> employee.getSalary() > 14000);
System.out.println(allMatch2); // false
Verification: If we go through the salary of all employees in the above list, all employee’s salary is greater than 5000
that’s why it returns the allMatch1 value is true
and because the employees having id = 12 & 67
, do not have a salary more than 14000
, so allMatch2 value is false
.
2. anyMatch()
anyMatch()
method returns true
if a single value is satisfied with given predicates otherwise it will return false
. anyMatch()
method is best suitable for Condition 2.
boolean anyMatch1 = employees.stream()
.anyMatch(employee -> employee.getSalary() > 13000);
System.out.println(anyMatch1); // true
boolean anyMatch2 = employees.stream()
.anyMatch(employee -> employee.getSalary() > 50000);
System.out.println(anyMatch2); // false
Verification: anyMatch1
value is true
because all employee’s salary is greater than 13000
except for employee id = 12 & 67
so the condition is satisfied but the anyMatch2 value is false
because not a single employee’s salary is greater than 50000
.
3. noneMatch()
For Condition 3, the noneMatch()
method is appropriate. It will return true
if the given predicated value doesn’t satisfied with the condition and if the condition is satisfied, it will return false
. Don’t confuse here, let’s try to understand with an example.
Suppose, you have a list of employees and you want to check whether an employee with the name XYZ is present in that employee list or not. noneMatch()
method will return true
if no employee with the name XYZ is available in the list and as method name suggests no-match and if any employee with the name XYZ is available in the list, it will return false
.
boolean noneMatch1 = employees.stream()
.noneMatch(employee -> employee.getName().equals("Atul"));
System.out.println(noneMatch1); // true
boolean noneMatch2 = employees.stream()
.noneMatch(employee -> employee.getName().equals("Kirti"));
System.out.println(noneMatch2); // false
Verification: If we check the name of all employees in the list, no employee name equals “Atul” and here the condition is satisfied so the noneMatch1 value is true
but the noneMatch2 value is false
because an employee whose id = 67
matched to the name “Kirti”.
Let’s check out the complete example.
package org.websparrow;
import java.util.List;
public class Java8StreamMatch {
public static void main(String[] args) {
List<Employee> employees = List.of(
new Employee(101, "Manish", 35000, "IT"),
new Employee(12, "Priyanka", 12000, "SALES"),
new Employee(67, "Kirti", 10000, "HR"),
new Employee(55, "Sagar", 25000, "SALES"),
new Employee(87, "Shilpa", 25000, "IT")
);
System.out.println("\n---------allMatch---------\n");
boolean allMatch1 = employees.stream().allMatch(employee -> employee.getSalary() > 5000);
System.out.println(allMatch1); // true
boolean allMatch2 = employees.stream().allMatch(employee -> employee.getSalary() > 14000);
System.out.println(allMatch2); // false
System.out.println("\n---------anyMatch---------\n");
boolean anyMatch1 = employees.stream().anyMatch(employee -> employee.getSalary() > 13000);
System.out.println(anyMatch1); // true
boolean anyMatch2 = employees.stream().anyMatch(employee -> employee.getSalary() > 50000);
System.out.println(anyMatch2); // false
System.out.println("\n---------noneMatch---------\n");
boolean noneMatch1 = employees.stream().noneMatch(employee -> employee.getName().equals("Atul"));
System.out.println(noneMatch1); // true
boolean noneMatch2 = employees.stream().noneMatch(employee -> employee.getName().equals("Kirti"));
System.out.println(noneMatch2); // false
}
}
Output:
---------allMatch---------
true
false
---------anyMatch---------
true
false
---------noneMatch---------
true
false
References
- allMatch()- Java 8 Stream
- anyMatch() – Java 8 Stream
- noneMatch() – Java 8 Stream
- Java 8 Stream- Sum of List of Integers
- Java 8 – How to sort Set with stream.sorted()