Spring Data MongoRepository Interface Methods Example
In this article, we’ll explore the methods available in the MongoRepository
interface provided by Spring Data in the package org.springframework.data.mongodb.repository
. MongoRepository
extends the PagingAndSortingRepository
and QueryByExampleExecutor
interfaces that further extends the CrudRepository interface.
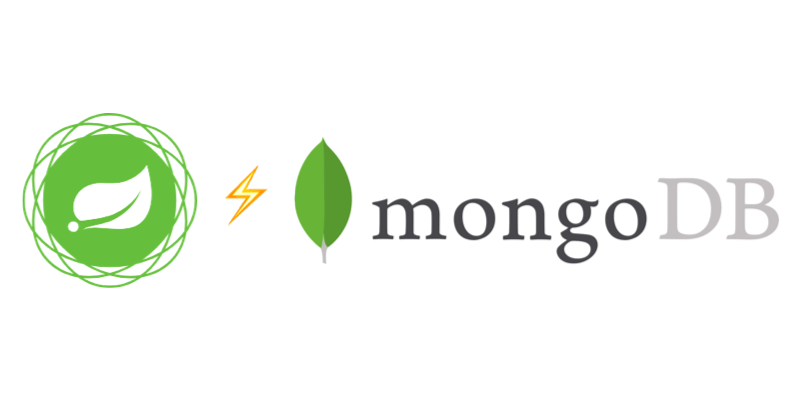
MongoRepository
provides all the necessary methods which help to create a CRUD application and it also supports the custom derived query methods.
public interface MongoRepository<T, ID> extends PagingAndSortingRepository<T, ID>, QueryByExampleExecutor<T>{}
where:
T is replaced by the document POJO class e.g. Person
, Employee
, etc.
ID is the data type used for id in the POJO class e.g. String
, Long
, Integer
.
There are only two new methods introduced in the
MongoRepository
interface and the rest are inherited from the CrudRepository interface.
1. insert(S entity)
insert(S entity)
method is used to save/persist the data into the MongoDB database and return the instance of save the entity.
Method signature:
<S extends T> S insert(S entity);
Example:
School school= new School("Delhi Public1 School", 1998, new String[]{"Science", "Art"}, 600);
schoolRepository.insert(school);
2. insert(Iterable<S> entities)
insert(Iterable<S> entities)
method is used to save the list of entities/documents in the MongoDB database at once.
Method signature:
<S extends T> List<S> insert(Iterable<S> entities);
Example:
List<School> schools = Arrays.asList(
new School("Delhi Public1 School", 1998, new String[]{"Science", "Art"}, 600),
new School("Mumbai Public School", 1995, new String[]{"Science"}, 450),
new School("Jaipur Public School", 2002, new String[]{"Science", "Art", "Commerce"}, 755),
new School("Kolkata Public School", 1995, new String[]{"Art"}, 300)
);
schoolRepository.insert(schools);
References
- Interface MongoRepository<T,ID>
- Spring Data CrudRepository Interface Example
- Spring Data JPA Derived Query Methods Example
Similar Posts
- Spring AOP pointcut example using XML configuration
- Difference Between @Component and @ComponentScan Annotations in Spring Boot
- Spring Boot Cookies Example
- Spring Collection (List, Set and Map) Dependency Injection Example
- Spring Boot Security- How to change default login page
- Spring Task Scheduler Example using @Scheduled Annotation
- Spring MVC user registration and login example using JdbcTemplate + MySQL
- Failed to start bean ‘documentationPluginsBootstrapper’; nested exception is java.lang.NullPointerException
- Spring dependency check using @Required annotation example
- Spring AOP + AspectJ @Pointcut Annotation Example