Java Stream map() vs flatMap() Method
Java Stream is a powerful feature introduced in Java 8 that allows developers to process collections of data in a functional and declarative way. Two commonly used methods in the Stream API are map()
and flatMap()
. While both methods are used for transforming elements in a stream, they have distinct differences in functionality.
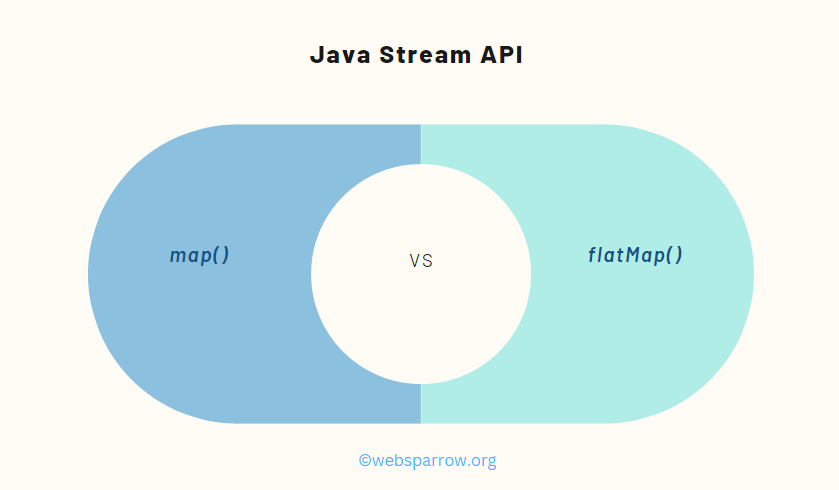
In this article, we will explore the differences between map()
and flatMap()
and provide examples to illustrate their usage.
1. The map()
Method
The map()
method is used to transform each element of a stream into another object. It takes a Function
as a parameter, which specifies how the transformation should be applied. The result is a new stream containing the transformed elements. The size of the resulting stream will be the same as the original stream.
Here’s an example to illustrate the usage of map()
:
package org.websparrow;
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class JavaStreamMapTest {
public static void main(String[] args) {
List<String> names = Arrays.asList("India", "Thailand", "Singapore", "Malaysia");
List<String> upperCaseNames = names.stream()
.map(String::toUpperCase)
.collect(Collectors.toList());
System.out.println(upperCaseNames);
}
}
In this example, we have a list of names. By using the map()
method with the toUpperCase()
method reference, we transform each name to uppercase. The resulting stream is then collected into a new list called upperCaseNames
. The output will be:
[INDIA, THAILAND, SINGAPORE, MALAYSIA]
Similar Posts:
2. The flatMap()
Method
The flatMap()
method is used to flatten a stream of collections or arrays into a single stream. It takes a Function
that produces a stream as a parameter. This function is applied to each element of the original stream, and the resulting streams are then concatenated into a single stream.
Let’s consider an example to understand the usage of flatMap()
:
package org.websparrow;
import java.util.Arrays;
import java.util.Collection;
import java.util.List;
import java.util.stream.Collectors;
public class JavaStreamFlatMapTest {
public static void main(String[] args) {
List<List<Integer>> numbers = Arrays.asList(
Arrays.asList(1, 2, 3),
Arrays.asList(4, 5, 6),
Arrays.asList(7, 8, 9)
);
List<Integer> flattenedNumbers = numbers.stream()
.flatMap(Collection::stream)
.collect(Collectors.toList());
System.out.println(flattenedNumbers);
}
}
In the above example, we have a list of lists containing numbers. By using the flatMap()
method with the Collection::stream
method reference, we flatten the nested lists into a single stream of numbers. The resulting stream is then collected into a new list called flattenedNumbers
. The output will be:
[1, 2, 3, 4, 5, 6, 7, 8, 9]
Key Differences
The key differences between the map()
and flatMap()
methods are as follows:
- Transformation: The
map()
method transforms each element of a stream into another object, while theflatMap()
method transforms each element of a stream into a stream of objects. - Resulting Stream: The
map()
method produces a stream of the same size as the original stream, containing the transformed elements. TheflatMap()
method produces a single flattened stream by concatenating the resulting streams of each element. - Nested Collections: The
map()
method does not handle nested collections directly. It operates on a per-element basis. In contrast, theflatMap()
method is designed to handle nested collections, allowing you to flatten them into a single stream. - Complex Transformations: The
flatMap()
method is more flexible when dealing with complex transformations. It allows you to transform each element into multiple elements or even none at all by returning an empty stream.
Summary
The map()
and flatMap()
methods in Java Stream provide powerful mechanisms for transforming elements in a stream. The map()
method is suitable for straightforward one-to-one transformations, while the flatMap()
method is useful for handling nested collections and more complex transformations.
References
- Java 8 Stream Interface
- Stream.map() – JavaDoc
- Stream.flatMap() – JavaDoc
- Java 8 Stream- Sum of List of Integers