Java Heap Memory vs Stack Memory: Understanding the Differences
On this page, we will explore the differences between Java Heap Memory vs Stack Memory and understand the difference. Java’s memory model consists of two primary memory areas: the heap and the stack and understanding memory management is crucial for developing efficient and robust applications.
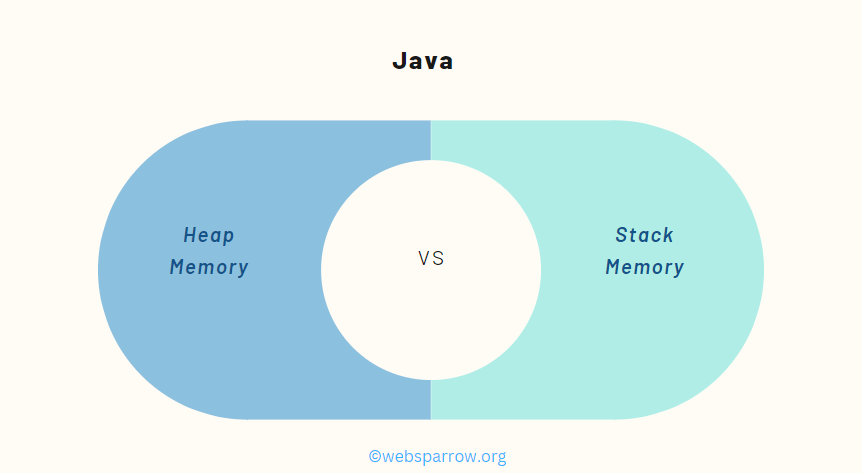
1. Java Heap Memory
The heap memory is a region where objects are dynamically allocated during runtime. It is a shared resource accessible to all threads in a Java application. The Java Virtual Machine (JVM) manages the heap memory and automatically handles the allocation and deallocation of objects. Here are some key points about the Java heap memory:
- Object Allocation: When you create an object using the
new
keyword, it is allocated to the heap memory. The size of an object is determined by the JVM based on its class definition. - Dynamic Memory: The heap memory is dynamic in nature, meaning it can grow or shrink during the execution of a program. Objects can be created and destroyed on the heap at runtime.
- Garbage Collection: The JVM employs automatic garbage collection to free up memory occupied by unreferenced objects. Garbage collection identifies and removes objects that are no longer reachable, making the memory available for new object allocations.
Let’s consider the below example where we create a Person
object and store it in the heap memory:
public class Person {
private String name;
private int age;
// Constructor
public Person(String name, int age) {
this.name = name;
this.age = age;
}
// Getters and setters
// ...
public static void main(String[] args) {
Person person = new Person("John Doe", 25);
// 'person' object is allocated on the heap
// ...
}
}
In the above example, the person
object is allocated on the heap memory using the new
keyword. The memory for the object is managed by the JVM, and it will be deallocated automatically by the garbage collector when it is no longer in use.
Post you may like: Stack implementation in Java using Array
2. Java Stack Memory
The stack memory is used to store local variables and method calls. It is organized as a stack data structure and operates in a last-in, first-out (LIFO) manner. Each thread in a Java application has its own stack memory, which is isolated from other threads. Here are some characteristics of the Java stack memory:
- Method Calls: When a method is called, a stack frame is created in the stack memory. The stack frame contains the method’s local variables, parameters, and the return address.
- Local Variables: Local variables defined within a method, including primitive data types and object references, are stored on the stack memory.
- Method Invocation and Return: When a method is invoked, a new stack frame is created and pushed onto the stack. When the method returns, its stack frame is popped from the stack, and the control returns to the calling method.
Consider the following code snippet that demonstrates method calls and stack memory usage:
public class StackExample {
public static void methodA() {
int x = 10;
methodB();
// After methodB() returns, control comes back here
}
public static void methodB() {
int y = 20;
// ...
}
public static void main(String[] args) {
methodA();
// ...
}
}
In the above example, when methodA()
is called from the main
method, a stack frame is created for methodA()
. Inside methodA()
, the local variable x
is stored on the stack. When methodB()
is called from methodA()
, a new stack frame is created for methodB()
, and the local variable y
is stored on the stack. Once methodB()
returns, its stack frame is popped, and the control returns to methodA()
.
Summary
The heap memory is used for dynamic allocation of objects, while the stack memory stores method calls and local variables. Both the heap and stack memory work together to provide a comprehensive memory management model in Java, allowing developers to focus on writing code without worrying about low-level memory operations.