How to add and rotate Image in PDF using iText and Java
In this example, we are going to show you how to add, rotate and change the position of an image on PDF file using iText API
in Java.
iText API
provides the Image class to handle the graphic element (JPEG, PNG or GIF) that has to be inserted into the document.
Image image = Image.getInstance("images/pm-modi.jpg");
Note : In my all examples I have used the
scaleToFit(float fitWidth, float fitHeight)
methods to change the image size.
Adding Image
This example will help you how to add the image in the document.
AddImage.java
package org.websparrow.itext;
import java.io.FileOutputStream;
import com.itextpdf.text.Document;
import com.itextpdf.text.Image;
import com.itextpdf.text.PageSize;
import com.itextpdf.text.pdf.PdfWriter;
public class AddImage {
public static void main(String[] args) {
try {
// Creating document and set the page size and margin
Document document = new Document(PageSize.A4, 20, 20, 20, 20);
PdfWriter.getInstance(document, new FileOutputStream("add-image.pdf"));
document.open();
// get the instance of image
Image image = Image.getInstance("images/pm-modi.jpg");
image.scaleToFit(90f, 90f);
document.add(image);
document.close();
System.out.println("Images added...");
} catch (Exception e) {
e.printStackTrace();
}
}
}
Output:
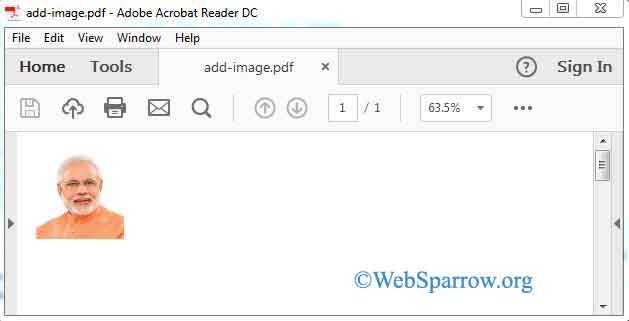
Rotate Image
Rotate the added image in the document.
RotateImage.java
package org.websparrow.itext;
import java.io.FileOutputStream;
import com.itextpdf.text.Document;
import com.itextpdf.text.Image;
import com.itextpdf.text.PageSize;
import com.itextpdf.text.pdf.PdfWriter;
public class RotateImage {
public static void main(String[] args) {
try {
// Creating document and set the page size and margin
Document document = new Document(PageSize.A4, 20, 20, 20, 20);
PdfWriter.getInstance(document, new FileOutputStream("rotate-image.pdf"));
document.open();
Image image = Image.getInstance("images/pm-modi.jpg");
image.scaleToFit(90f, 90f);
image.setRotation(180f);
document.add(image);
document.close();
System.out.println("Images rotated...");
} catch (Exception e) {
e.printStackTrace();
}
}
}
Output:
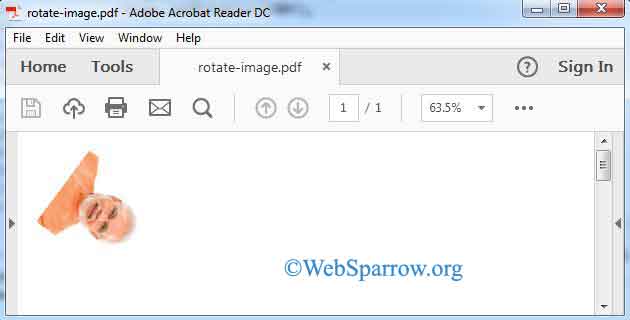
Positioning Image
In this example, we have to change the position of the image using the X-axis and Y-axis.
ImagePositioning.java
package org.websparrow.itext;
import java.io.FileOutputStream;
import com.itextpdf.text.Document;
import com.itextpdf.text.Image;
import com.itextpdf.text.PageSize;
import com.itextpdf.text.pdf.PdfWriter;
public class ImagePositioning {
public static void main(String[] args) {
try {
// Creating document and set the page size and margin
Document document = new Document(PageSize.A4, 20, 20, 20, 20);
PdfWriter.getInstance(document, new FileOutputStream("image-position.pdf"));
document.open();
Image image = Image.getInstance("images/pm-modi.jpg");
image.scaleToFit(90f, 90f);
image.setAbsolutePosition(350f, 15f);
document.add(image);
document.close();
System.out.println("Image Positioned...");
} catch (Exception e) {
e.printStackTrace();
}
}
}
Output:
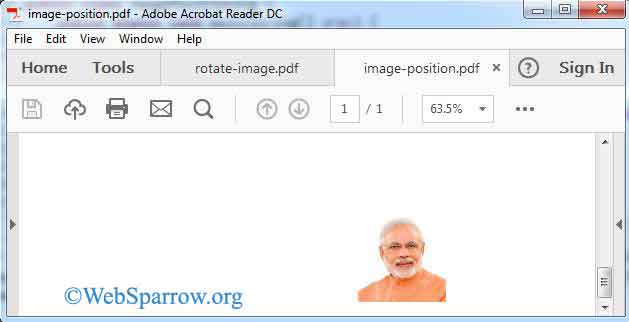