How to iterate list on JSP in Spring MVC
To iterate the ArrayList or any list on JSP in Spring MVC framework, you can use the JSTL (JavaServer Pages Standard Tag Library) library. It provides many features like handling core, database operation, function, and i18n support.
To use the JSTL core tags in JSP pages by adding the following taglib
directive:
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
And to iterate list values, JSTL core have <c:forEach/> tag. It will display the list values one by one.
<c:forEach var="emp" items="${empList}">
...
</c:forEach>
Dependency Required
You need to add Spring MVC and JSTL dependency in your project.
<dependencies>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>5.0.2.RELEASE</version>
</dependency>
<dependency>
<groupId>jstl</groupId>
<artifactId>jstl</artifactId>
<version>1.2</version>
</dependency>
</dependencies>
Controller
Create a controller ListController
class that returns the list to the view.
package org.websparrow.controller;
import java.util.ArrayList;
import java.util.List;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.servlet.ModelAndView;
@Controller
public class ListController {
@RequestMapping(value = "/list", method = RequestMethod.GET)
public ModelAndView listAction() {
List<String> empList = new ArrayList<>();
empList.add("Atul");
empList.add("Abhinav");
empList.add("Prince");
empList.add("Gaurav");
ModelAndView mv = new ModelAndView();
mv.setViewName("index");
mv.addObject("empList", empList);
return mv;
}
}
Views
Create a JSP page to iterate the list.
<%@ page isELIgnored="false" %>
<%@taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
<html>
<body>
<h2>How to iterate list on JSP in Spring MVC</h2>
<p><b>Simple List:</b><p>
${empList}
<p><b>Iterated List:</b><p>
<ol>
<c:forEach var="emp" items="${empList}">
<li>${emp}</li>
</c:forEach>
</ol>
</body>
</html>
Output
Run the application and hit the following URL into your browser…
localhost:8080/spring-mvc-iterate-list/list
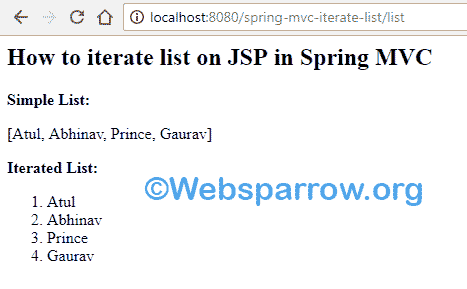
References
Download Source Code: how-to-iterate-list-on-jsp-in-spring-mvc.zip