Spring MVC Database Connectivity using XML Configuration
In this article, you will learn Spring MVC database connectivity using XML configuration. Spring framework provides DriverManagerDataSource
class to manage the database connection in its JDBC module. DriverManagerDataSource
have fields like driverClassName, URL, username, and password to create the database connection object.
As mentioned in the headline, we going to create database connection object using XML configuration and check if the connection is not null then return the SUCCESS message otherwise FAILURE message.
Similar Post: Spring MVC Database Connectivity Example using Annotation and Java Based Configuration
Dependency Required
Find the list of dependencies used in this example.
<dependencies>
<!-- spring mvc dependency -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>5.0.2.RELEASE</version>
</dependency>
<!-- spring jdbc dependency -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-jdbc</artifactId>
<version>5.0.2.RELEASE</version>
</dependency>
<!-- mysql databse connector -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>6.0.6</version>
</dependency>
</dependencies>
Technology Used
Find the list of all technologies used in this application.
- Eclipse Oxygen
- Tomcat 9
- JDK 8
- Maven 3
- Spring5.0.2.RELEASE
Project Structure
Final project structure of our application in Eclipse IDE will look like as follows.
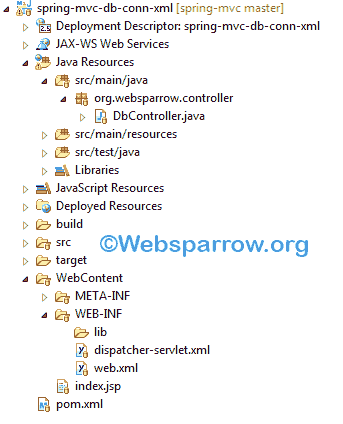
Configure Front Controller
Add the Spring MVC front controller i.e. DispatcherServlet
to your web.xml file.
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" id="WebApp_ID"
version="2.5">
<display-name>spring-mvc-db-conn-xml</display-name>
<servlet>
<servlet-name>dispatcher</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>dispatcher</servlet-name>
<url-pattern>*.htm</url-pattern>
</servlet-mapping>
</web-app>
Create Controller
To check database connection, create a DbController
class that implements Controller
interface which has one method handleRequest(request, response)
and its return type is ModelAndView
.
package org.websparrow.controller;
import java.util.HashMap;
import java.util.Map;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.sql.DataSource;
import org.springframework.web.servlet.ModelAndView;
import org.springframework.web.servlet.mvc.Controller;
public class DbController implements Controller {
DataSource dataSource;
public void setDataSource(DataSource dataSource) {
this.dataSource = dataSource;
}
public ModelAndView handleRequest(HttpServletRequest request, HttpServletResponse response) throws Exception {
Map<String, String> map = new HashMap<String, String>();
if (dataSource.getConnection() != null) {
map.put("msg", "Database connection established successfully.");
} else {
map.put("msg", "Failed to connect database.");
}
return new ModelAndView("index", map);
}
}
Map Action Request
Map the controller class to the action name and set the database credentials into the DriverManagerDataSource
property.
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:p="http://www.springframework.org/schema/p" xmlns:context="http://www.springframework.org/schema/context"
xmlns:mvc="http://www.springframework.org/schema/mvc"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd
http://www.springframework.org/schema/mvc
http://www.springframework.org/schema/mvc/spring-mvc.xsd">
<bean class="org.springframework.web.servlet.view.InternalResourceViewResolver">
<property name="prefix" value="/" />
<property name="suffix" value=".jsp" />
</bean>
<bean id="dmds" class="org.springframework.jdbc.datasource.DriverManagerDataSource">
<property name="driverClassName" value="com.mysql.jdbc.Driver" />
<property name="url" value="jdbc:mysql://localhost:3306/websparrow" />
<property name="username" value="root" />
<property name="password" value="" />
</bean>
<bean name="/checkConnection.htm" class="org.websparrow.controller.DbController">
<property name="dataSource" ref="dmds"></property>
</bean>
</beans>
View Components
Create the JSP pages that visible on the user machine.
<html>
<body>
<h2>Spring MVC Database Connectivity Example using XML configuration</h2>
<b>Message:</b> ${msg}
</body>
</html>
Output
Finally, everything was completed. Run your project and will get the following result.
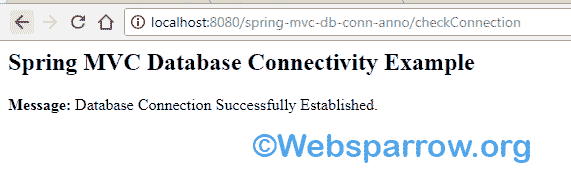
References
- Spring MVC Database Connectivity Example using Annotation and Java Based Configuration
- Spring 5 MVC Hello World using XML configuration
- Spring Web MVC
- Front Controller- Dispatcher Servlet
Download Source Code: spring-mvc-database-connectivity-using-xml-configuration.zip