Java 8 – Find Non Duplicate Elements from List
In Java 8, you can use the Stream API and lambda expression features to find the non-duplicates element from a list.
Example:
//Test Case 1
int[] nums = [1, 4, 8, 1, 11, 5, 4];
Output = [8, 11, 5]
//Test Case 2
String[] cities = ["Bengaluru", "Varanasi", "New Delhi", "Bengaluru"];
Output = ["Varanasi", "New Delhi"]
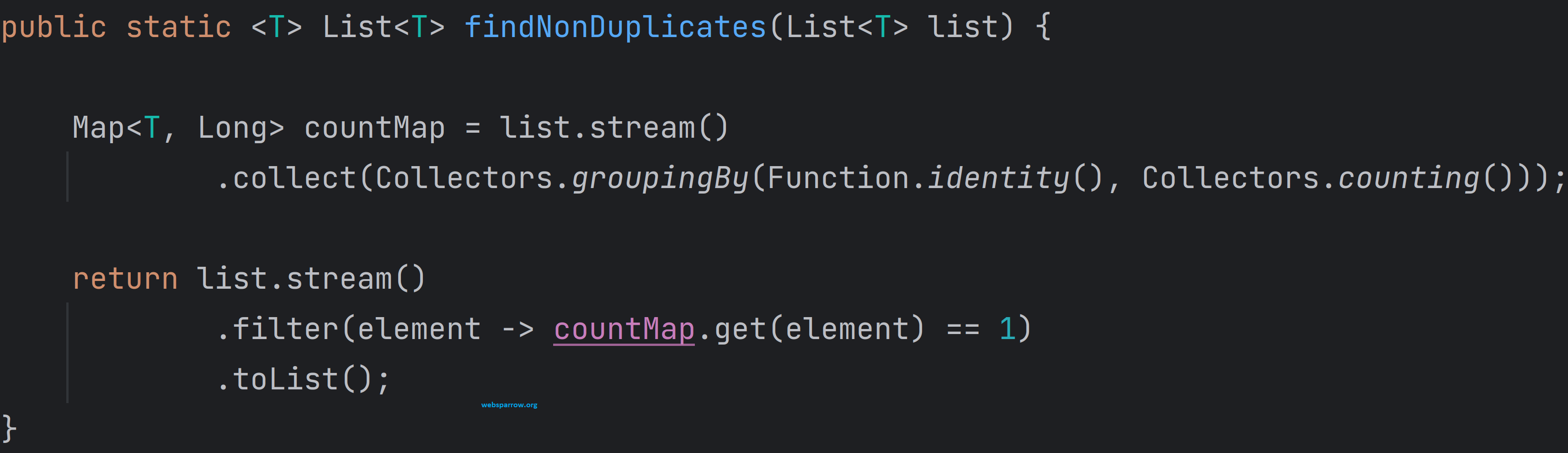
Here’s an example that demonstrates the usage using Java 8 features:
FindNonDuplicate.java
package org.websparrow;
import java.util.Arrays;
import java.util.List;
import java.util.Map;
import java.util.function.Function;
import java.util.stream.Collectors;
public class FindNonDuplicate {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 4, 8, 1, 11, 5, 4);
List<Integer> nonDuplicateNums = findNonDuplicates(numbers);
System.out.println("Non Duplicate Numbers: " + nonDuplicateNums);
List<String> cities = Arrays.asList("Bengaluru", "Varanasi", "New Delhi", "Bengaluru");
List<String> nonDuplicateCities = findNonDuplicates(cities);
System.out.println("Non Duplicate Cities: " + nonDuplicateCities);
}
public static <T> List<T> findNonDuplicates(List<T> list) {
Map<T, Long> countMap = list.stream()
.collect(Collectors.groupingBy(Function.identity(), Collectors.counting()));
return list.stream()
.filter(element -> countMap.get(element) == 1)
.toList();
}
}
In the above code, the findNonDuplicates()
method uses the Collectors.groupingBy()
collector to group the elements in the list based on their identity (i.e., the element itself) and count their occurrences using Collectors.counting()
. The result is a map (countMap
) with each element as the key and its count as the value.
Then, using the stream again, we filter out the elements that have a count of 1 (i.e., non-duplicates) by accessing the count from the countMap
and collect them into a new list using toList()
.
The output will be:
console.log
Non Duplicate Numbers: [8, 11, 5]
Non Duplicate Cities: [Varanasi, New Delhi]
References
- Java 8- Find the nth Highest Salary
- Java 8 Stream- Sum of List of Integers
- Collectors – JavaDoc
- Stream API – JavaDoc