Find second index of a substring in a String in Java
In Java, we can use the overloaded method indexOf(String str, int fromIndex)
of the String
class to get the second index of a substring from a given string.
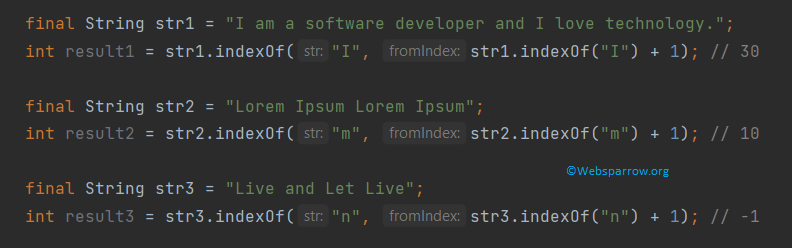
The return type of indexOf(String str, int fromIndex)
method is int and it will return -1 if there is no such occurrence found.
public int indexOf(String str, int fromIndex)
1. Java indexOf(String str, int fromIndex)
A complete Java example to find the second occurrence of a substring in a string.
SecondIndex.java
package org.websparrow;
public class SecondIndex {
public static void main(String[] args) {
// Test Case 1
final String str1 = "I am a software developer and I love technology.";
int result1 = getSecondIndex(str1, "I"); // 30
System.out.println(result1);
// Test Case 2
final String str2 = "Lorem Ipsum Lorem Ipsum";
int result2 = getSecondIndex(str2, "m"); // 10
System.out.println(result2);
// Test Case 3
final String str3 = "Live and Let Live";
int result3 = getSecondIndex(str3, "n"); // -1
System.out.println(result3);
}
private static int getSecondIndex(final String str, final String subStr) {
return str.indexOf(subStr, str.indexOf(subStr) + 1);
}
}
Output
30
10
-1
2. Apache Commons Lang 3, StringUtils
Alternatively, we can use the Apache commons-lang3
library, StringUtils.ordinalIndexOf(CharSequence str, CharSequence searchStr, int ordinal);
API to get the second index of a substring from a given string.
pom.xml
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.12.0</version>
</dependency>
SecondIndexApache.java
package org.websparrow;
import org.apache.commons.lang3.StringUtils;
public class SecondIndexApache {
public static void main(String[] args) {
// Test Case 1
final String str1 = "I am a software developer and I love technology.";
int result1 = StringUtils.ordinalIndexOf(str1, "I", 2); // 30
System.out.println(result1);
// Test Case 2
final String str2 = "Lorem Ipsum Lorem Ipsum";
int result2 = StringUtils.ordinalIndexOf(str2, "m", 2); // 10
System.out.println(result2);
// Test Case 3
final String str3 = "Live and Let Live";
int result3 = StringUtils.ordinalIndexOf(str3, "n", 2); // -1
System.out.println(result3);
}
}
Output
30
10
-1